用 Ruby 编写 switch 语句
Nurudeen Ibrahim
2023年1月30日
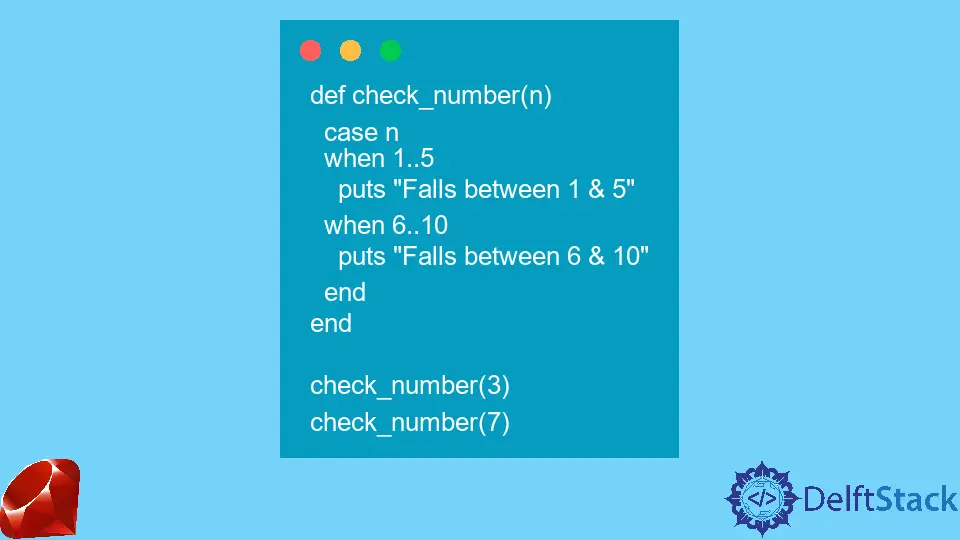
与 if
语句的工作方式类似,switch
语句允许我们根据某些特定条件控制代码的执行。本教程将介绍如何在 Ruby 中使用 switch
语句。
下面是 Ruby 中 switch
语句的语法。
case argument
when condition
# do something
else
# do something else if nothing else meets the condition
end
我们将研究一些常见的基本使用方法,然后介绍一些不太常见的复杂示例。
示例代码:
def continent_identifier(country)
case country
when "Nigeria"
puts "Africa"
when "Netherlands"
puts "Europe"
when "India"
puts "Asia"
else
puts "I don't know"
end
end
continent_identifier("India")
continent_identifier("Netherlands")
continent_identifier("Germany")
输出:
Asia
Europe
I don't know
上面是一个使用 switch
语句来识别一个国家的大陆的方法的基本示例。看上面的例子,以下是值得注意的。
- 与大多数其他编程语言中的
switch
语句不同,Ruby 的switch
语句不需要在每个when
的末尾使用break
。 - Ruby 的
switch
语句允许我们为每个when
指定多个值,因此如果我们的变量匹配任何值,我们就可以返回结果。一个例子如下所示。
示例代码:
def continent_identifier(country)
case country
when "Nigeria"
puts "Africa"
when "Netherlands", "Germany"
puts "Europe"
when "India"
puts "Asia"
else
puts "I don't know"
end
end
continent_identifier("India")
continent_identifier("Netherlands")
continent_identifier("Germany")
输出:
Asia
Europe
Europe
Ruby 的 switch
语句在底层使用 ===
运算符来比较 case
变量和提供的值,即 value === argument
。因此,Ruby switch
允许我们进行更巧妙的比较。
在 Ruby switch
中匹配 Ranges
在此示例中,我们检查提供的 case
变量是否包含在指定的任何 Ranges 中。
示例代码:
def check_number(n)
case n
when 1..5
puts "Falls between 1 & 5"
when 6..10
puts "Falls between 6 & 10"
end
end
check_number(3)
check_number(7)
输出:
Falls between 1 & 5
Falls between 6 & 10
在 Ruby switch
中匹配 Regex
我们还可以将正则表达式与 case
变量进行匹配,如下面的示例所示。
示例代码:
def month_indentifier(month)
case month
when /ber$/
puts "ends with 'ber'"
when /ary$/
puts "ends with 'ary'"
end
end
month_indentifier("February")
month_indentifier("November")
输出:
ends with 'ary'
ends with 'ber'
在 Ruby switch
中匹配 Proc
Ruby Proc
封装了可以存储在变量中或传递的代码块。你可以查看此处以获取有关 Ruby Proc
的更多说明。以下示例显示了如何在 switch
语句中使用 Ruby Proc
。
示例代码:
def check_number(number)
case number
when -> (n) { n.even? }
puts "It's even"
when -> (n) { n.odd? }
puts "It's odd"
end
end
check_number(3)
check_number(6)
输出:
It's odd
It's even