在 Python 中计算列表的标准差
-
在 Python 中使用
statistics
模块的pstdev()
函数计算列表的标准偏差 -
在 Python 中使用
NumPy
库的std()
函数计算列表的标准偏差 -
在 Python 中使用
sum()
函数和列表推导式计算列表的标准偏差
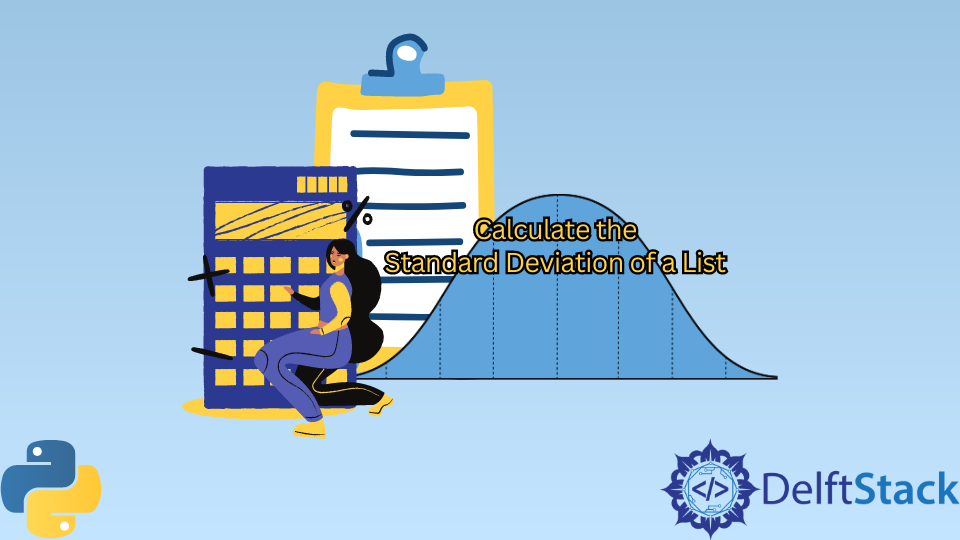
在 Python 中,有很多统计操作正在执行。这些操作之一是计算给定数据的标准偏差。数据的标准偏差告诉我们数据偏离平均值的程度。在数学上,标准偏差等于方差的平方根。
本教程将演示如何在 Python 中计算列表的标准偏差。
在 Python 中使用 statistics
模块的 pstdev()
函数计算列表的标准偏差
pstdev()
函数是 Python 的 statistics
模块下的命令之一。statistics
模块提供了对 Python 中的数值数据执行统计操作的函数,如均值、中位数和标准差。
statistics
模块的 pstdev()
函数帮助用户计算整个总体的标准偏差。
import statistics
list = [12, 24, 36, 48, 60]
print("List : " + str(list))
st_dev = statistics.pstdev(list)
print("Standard deviation of the given list: " + str(st_dev))
输出:
List : [12, 24, 36, 48, 60]
Standard deviation of the given list: 16.97056274847714
在上面的示例中,str()
函数将整个列表及其标准偏差转换为字符串,因为它只能与字符串连接。
在 Python 中使用 NumPy
库的 std()
函数计算列表的标准偏差
NumPy
代表 Numerical Python
是 Python 中广泛使用的库。这个库有助于处理数组、矩阵、线性代数和傅立叶变换。
NumPy
库的 std()
函数用于计算给定数组(列表)中元素的标准偏差。参考下面的示例。
import numpy as np
list = [12, 24, 36, 48, 60]
print("List : " + str(list))
st_dev = np.std(list)
print("Standard deviation of the given list: " + str(st_dev))
输出:
List : [12, 24, 36, 48, 60]
Standard deviation of the given list: 16.97056274847714
在 Python 中使用 sum()
函数和列表推导式计算列表的标准偏差
顾名思义,sum()
函数提供可迭代对象(如列表或元组)的所有元素的总和。列表推导式是一种从现有列表中存在的元素创建列表的方法。
sum()
函数和列表推导式可以帮助计算列表的标准偏差。这是一个示例代码。
import math
list= [12, 24, 36, 48, 60]
print("List : " + str(list))
mean = sum(list) / len(list)
var = sum((l-mean)**2 for l in list) / len(list)
st_dev = math.sqrt(var)
print("Standard deviation of the given list: " + str(st_dev))
输出:
List : [12, 24, 36, 48, 60]
Standard deviation of the given list: 16.97056274847714
在上面的示例中,导入了 math
模块。它提供了 sqrt()
函数来计算给定值的平方根。另外,请注意还使用了函数 len()
。此函数有助于提供给定列表的长度,例如,列表中的元素数。
该方法基于标准差的数学公式。首先,我们计算方差,然后求其平方根以找到标准偏差。
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn