在 Python 中生成范围内的随机整数
-
在 Python 中使用
random.randint()
函数在特定范围之间生成随机整数 -
在 Python 中使用
random.randrange()
函数在特定范围之间生成随机整数 -
在 Python 中使用
random.sample()
函数在特定范围之间生成随机整数 -
在 Python 中使用
NumPy
模块在特定范围之间生成随机整数
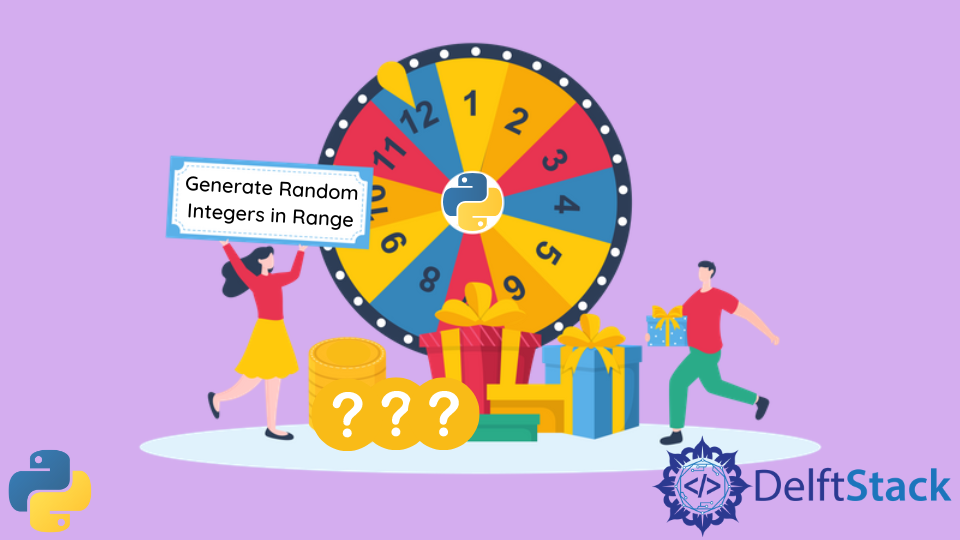
Python 是用于数据分析的非常有用的工具。当我们处理现实情况时,我们必须生成随机值以模拟情况并对其进行处理。
Python 具有可用的 random
和 NumPy
模块,它们具有有效的方法,可轻松地工作和生成随机数。
在本教程中,我们将在 Python 的特定范围之间生成一些随机整数。
在 Python 中使用 random.randint()
函数在特定范围之间生成随机整数
randint()
函数用于生成指定范围之间的随机整数。起点和终点位置作为参数传递给函数。
例如,
import random
x = random.randint(0,10)
print(x)
输出:
8
要使用此函数生成一个随机数列表,我们可以将列表推导方法与 for
循环一起使用,如下所示:
import random
x = [random.randint(0, 9) for p in range(0, 10)]
print(x)
输出:
[1, 6, 6, 5, 8, 8, 5, 5, 8, 4]
请注意,此方法仅接受整数值。
在 Python 中使用 random.randrange()
函数在特定范围之间生成随机整数
randrange()
函数还返回一个范围内的随机数,并且仅接受整数值,但是这里我们可以选择指定一个非常有用的参数称为 step
。step
参数使我们能够找到可被特定数字整除的随机数字。缺省情况下,该参数为 1。
例如,
import random
x = random.randrange(0,10,2)
print(x)
输出:
4
请注意,输出可被 2 整除。使用相同的列表推导方法,我们可以使用此函数生成一个随机数列表,如下所示。
import random
x = [random.randrange(0, 10, 2) for p in range(0, 10)]
print(x)
输出:
[8, 0, 6, 2, 0, 6, 8, 6, 0, 4]
在 Python 中使用 random.sample()
函数在特定范围之间生成随机整数
使用此函数,我们可以指定要生成的随机数的范围和总数。它还可以确保不存在重复的值。以下示例显示了如何使用此函数。
import random
x = random.sample(range(10),5)
print(x)
输出:
[7, 8, 5, 9, 6]
在 Python 中使用 NumPy
模块在特定范围之间生成随机整数
NumPy
模块还具有三个函数可用于完成此任务,并生成所需数量的随机整数并将其存储在 numpy 数组中。
这些函数是 numpy.random.randint()
,numpy.random.choice()
和 numpy.random.uniform()
。以下代码显示了如何使用这些函数。
例如,
import numpy as np
x1 = np.random.randint(low=0, high=10, size=(5,))
print(x1)
x2 = np.random.uniform(low=0, high=10, size=(5,)).astype(int)
print(x2)
x3 = np.random.choice(a=10, size=5)
print(x3)
输出:
[3 2 2 2 8]
[8 7 9 2 9]
[0 7 4 1 4]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn