在 Python 中漂亮地打印 XML 输出
Vaibhav Vaibhav
2023年1月30日
2022年5月18日
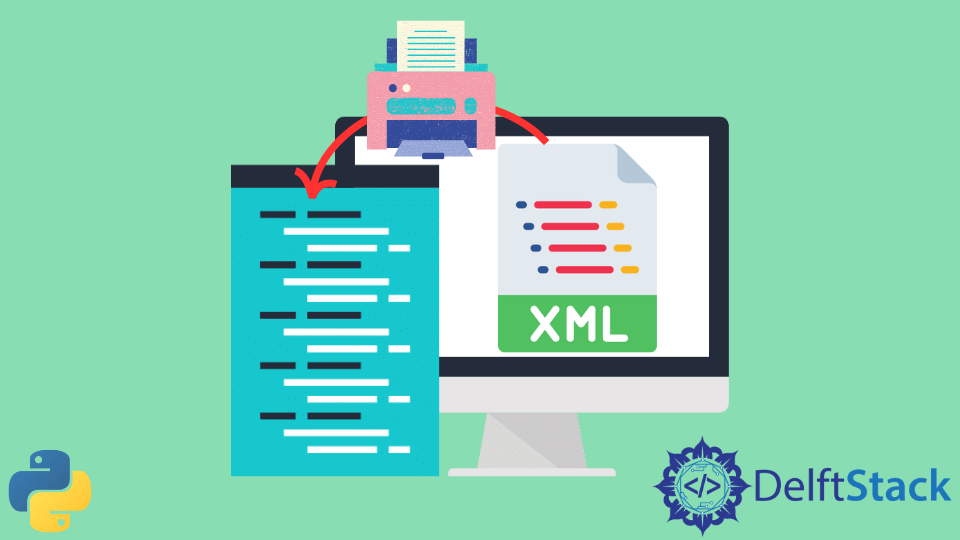
在读取文本文件、HTML 文件、XML 文件等时,文件的内容结构很差,并且包含不一致的缩进。这种差异使得难以理解输出。这个问题可以通过美化此类文件的输出来解决。美化包括修复不一致的缩进、去除随机空格等。
在本文中,我们将学习如何使 XML 文件输出更漂亮。为了让我们都在同一个页面上,以下 Python 代码将考虑此 XML 文件。如果你希望使用相同的文件,你只需将 XML 内容复制到名为 books.xml
的新文件中。
在 Python 中使用 BeautifulSoap
库制作漂亮的 XML 输出
BeautifulSoup
是一个基于 Python 的库,用于解析 HTML 和 XML 文件。它通常用于从网站和文档中抓取数据。我们可以使用这个库来美化 XML 文档的输出。
如果你的机器上没有安装此库,请使用以下任一 pip
命令。
pip install beautifulsoup4
pip3 install beautifulsoup4
我们必须安装另外两个模块才能使用这个库:html5lib
和 lxml
。html5lib
库是一个基于 Python 的库,用于解析 HTML 或超文本标记语言文档。而且,lxml
库是处理 XML 文件的实用程序的集合。使用以下 pip
命令在你的机器上安装这些库。
pip install html5lib
pip install lxml
请参考以下 Python 代码了解用法。
from bs4 import BeautifulSoup
filename = "./books.xml"
bs = BeautifulSoup(open(filename), "xml")
xml_content = bs.prettify()
print(xml_content)
输出:
<?xml version="1.0" encoding="utf-8"?>
<catalog>
<book id="bk101">
<author>
Gambardella, Matthew
</author>
<title>
XML Developer's Guide
</title>
<genre>
Computer
</genre>
<price>
44.95
</price>
<publish_date>
2000-10-01
</publish_date>
<description>
An in-depth look at creating applications
with XML.
</description>
</book>
...
<book id="bk111">
<author>
O'Brien, Tim
</author>
<title>
MSXML3: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
36.95
</price>
<publish_date>
2000-12-01
</publish_date>
<description>
The Microsoft MSXML3 parser is covered in
detail, with attention to XML DOM interfaces, XSLT processing,
SAX and more.
</description>
</book>
<book id="bk112">
<author>
Galos, Mike
</author>
<title>
Visual Studio 7: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
49.95
</price>
<publish_date>
2001-04-16
</publish_date>
<description>
Microsoft Visual Studio 7 is explored in depth,
looking at how Visual Basic, Visual C++, C#, and ASP+ are
integrated into a comprehensive development
environment.
</description>
</book>
</catalog>
上面的代码认为 books.xml
文件位于当前工作目录中。或者,我们也可以在 filename
变量中设置文件的完整路径。prettify()
函数美化了 XML 文件的输出。请注意,为了便于理解,已对输出进行了修剪。
在 Python 中使用 lxml
库使 XML 输出漂亮
我们可以单独使用 lxml
库来美化 XML 文件的输出。请参阅以下 Python 代码。
from lxml import etree
filename = "./books.xml"
f = etree.parse(filename)
content = etree.tostring(f, pretty_print = True, encoding = str)
print(content)
输出:
<?xml version="1.0" encoding="utf-8"?>
<catalog>
<book id="bk101">
<author>
Gambardella, Matthew
</author>
<title>
XML Developer's Guide
</title>
<genre>
Computer
</genre>
<price>
44.95
</price>
<publish_date>
2000-10-01
</publish_date>
<description>
An in-depth look at creating applications
with XML.
</description>
</book>
...
<book id="bk111">
<author>
O'Brien, Tim
</author>
<title>
MSXML3: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
36.95
</price>
<publish_date>
2000-12-01
</publish_date>
<description>
The Microsoft MSXML3 parser is covered in
detail, with attention to XML DOM interfaces, XSLT processing,
SAX and more.
</description>
</book>
<book id="bk112">
<author>
Galos, Mike
</author>
<title>
Visual Studio 7: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
49.95
</price>
<publish_date>
2001-04-16
</publish_date>
<description>
Microsoft Visual Studio 7 is explored in depth,
looking at how Visual Basic, Visual C++, C#, and ASP+ are
integrated into a comprehensive development
environment.
</description>
</book>
</catalog>
注意 pretty_print = True
参数。这个标志使输出更漂亮。
Author: Vaibhav Vaibhav