如何用 Python 将列表写入文件
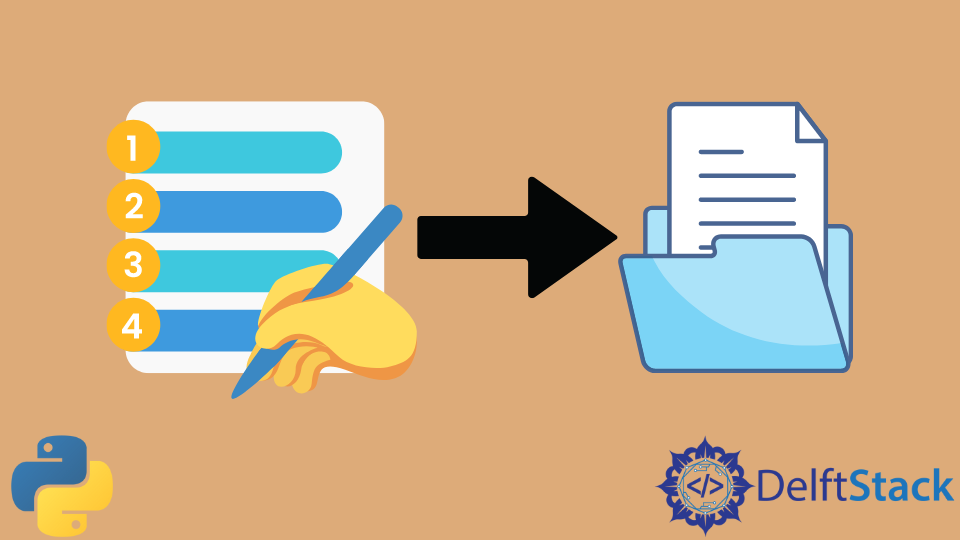
本教程说明了如何用 Python 将一个列表写到文件中。由于有多种方法可以将一个列表写入文件,本教程还列出了各种示例代码来进一步解释它们。
在 Python 中使用循环把一个列表写到文件上
使用循环将列表写到文件中是一种非常琐碎也是最常用的方法。循环用来迭代列表项,而 write()
方法则用来将列表项写入文件。
我们使用 open()
方法来打开目标文件。打开文件的模式应该是 w
,代表 write
。
示例代码如下:
listitems = ["ab", "cd", "2", "6"]
with open('abc.txt', 'w') as temp_file:
for item in listitems:
temp_file.write("%s\n" % item)
file = open('abc.txt', 'r')
print(file.read())
输出:
{'6','2', 'ab', 'cd'}
对于 Python 2.x,你也可以使用下面的方法。
listitems = ["ab", "cd", "2", "6"]
with open('xyz.txt', 'w') as temp_file:
for item in listitems:
print >> temp_file, item
这两个代码的结果都是用 Python 把列表写到一个文件中。
在 Python 中使用 Pickle
模块把一个列表写入一个文件
Python 的 Pickle
模块用于序列化或去序列化一个 Python 对象结构。如果你想序列化一个列表,以便以后在同一个 Python 文件中使用,可以使用 pickle
。由于 pickle
模块实现了二进制协议,所以文件也应该以二进制写入模式-wb
打开。
pickle.dump()
方法用于将一个列表写入文件。它把列表和文件的引用作为参数。
这种方法的示例代码如下:
import pickle
listitems = ["ab", "cd", "2", "6"]
with open('outputfile', 'wb') as temp:
pickle.dump(listitems, temp)
with open ('outfile', 'rb') as temp:
items = pickle.load(temp)
print(items)
输出:
{'ab','cd', '6', '2'}
使用 join()
方法将列表写入文件
另一种更直接的方法是使用 join()
方法在 Python 中把一个列表写入文件。它将列表中的元素作为输入。
下面是一个使用该方法的示例代码。
items = ["a", "b", "c", "d"]
with open("outputfile", "w") as opfile:
opfile.write("\n".join(items))
使用 JSON
模块将列表写入文件
在 Python 中,pickle
模块的使用非常特殊。JSON 使不同的程序更加高效和容易理解。JSON
模块用于将混合变量类型写入文件。
它使用 dump()
方法,将元素列表和对文件的引用作为输入。
示例代码如下:
import json
itemlist = [21, "Tokyo", 3.4]
with open('opfile.txt', 'w') as temp_op:
with open('opfile.txt', 'r') as temp_op:
templist = json.load(temp_op)
print(templist)
输出:
[21, "Tokyo", 3.4]
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn相关文章 - Python List
- 从 Python 列表中删除某元素的所有出现
- 在 Python 中将字典转换为列表
- 在 Python 中从列表中删除重复项
- 如何在 Python 中获取一个列表的平均值
- Python 列表方法 append 和 extend 之间有什么区别
- 如何在 Python 中将列表转换为字符串