在 Python 中将列表拆分成块
- 使用列表推导式方法将 Python 中的列表拆分为块
-
在 Python 中使用
itertools
方法将列表拆分为块状 -
在 Python 中使用
lambda
函数将 Python 中的列表拆分为块 -
在 Python 中使用
lambda
和islice
方法将列表拆分为块 -
在 Python 中使用
NumPy
方法将列表拆分为块 - 在 Python 中使用用户定义的函数将列表拆分成块
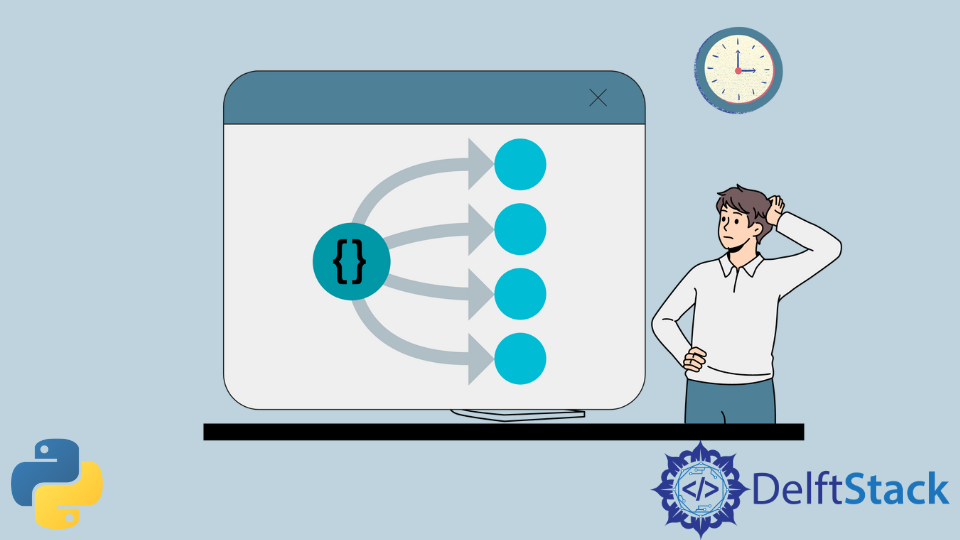
Python 数据结构中的一种可以在其中包含混合值或元素的结构叫做列表。本文将介绍将一个列表分割成块的各种方法。你可以使用任何符合你规范的代码示例。
使用列表推导式方法将 Python 中的列表拆分为块
我们可以使用列表推导式将一个 Python 列表分割成块。这是一种封装操作的有效方法,使代码更容易理解。
完整的示例代码如下。
test_list = ['1','2','3','4','5','6','7','8','9','10']
n=3
output=[test_list[i:i + n] for i in range(0, len(test_list), n)]
print(output)
输出:
[['1', '2', '3'], ['4', '5', '6'], ['7', '8', '9'], ['10']]
range(0, len(test_list), n)
返回一个从 0 开始到 len(test_list)
结束的数字范围,步长为 n
。例如,range(0, 10, 3)
将返回 (0, 3, 6, 9)
。
test_list[i:i + n]
得到从索引 i
开始,到 i + n
结束的列表块。拆分列表的最后一块是 test_list[9]
,但计算出的指数 test_list[9:12]
不会出错,而是等于 test_list[9]
。
在 Python 中使用 itertools
方法将列表拆分为块状
该方法提供了一个必须使用 for
循环进行迭代的生成器。生成器是描述迭代器的一种有效方式。
from itertools import zip_longest
test_list = ['1','2','3','4','5','6','7','8','9','10']
def group_elements(n, iterable, padvalue='x'):
return zip_longest(*[iter(iterable)]*n, fillvalue=padvalue)
for output in group_elements(3,test_list):
print(output)
输出:
('1', '2', '3')
('4', '5', '6')
('7', '8', '9')
('10', 'x', 'x')
[iter(iterable)]*n
生成一个迭代器,并在列表中迭代 n
次。然后通过 izip-longest
有效地对每一个迭代器进行轮回,由于这是一个类似的迭代器,所以每一次这样的调用都是高级的,结果每一次这样的 zip 轮回都会产生一个 n 个对象的元组。
在 Python 中使用 lambda
函数将 Python 中的列表拆分为块
可以使用一个基本的 lambda
函数将列表分成一定大小或更小的块。这个函数工作在原始列表和 N 个大小的变量上,遍历所有的列表项,并将其分成 N 个大小的小块。
完整的示例代码如下:
test_list = ['1','2','3','4','5','6','7','8','9','10']
x = 3
final_list= lambda test_list, x: [test_list[i:i+x] for i in range(0, len(test_list), x)]
output=final_list(test_list, x)
print('The Final List is:', output)
输出:
The Final List is: [['1', '2', '3'], ['4', '5', '6'], ['7', '8', '9'], ['10']]
在 Python 中使用 lambda
和 islice
方法将列表拆分为块
lambda
函数可以与 islice
函数一起使用,产生一个在列表上迭代的生成器。islice
函数创建一个迭代器,从可迭代元素中提取选定的项目。如果起始值是非零,那么在到达起始值之前将跳过可迭代的元素。然后,元素将被连续返回,除非一个步长设置的高于一个步长,导致元素被跳过。
完整的示例代码如下:
from itertools import islice
test_list = ['1','2','3','4','5','6','7','8','9','10']
def group_elements(lst, chunk_size):
lst = iter(lst)
return iter(lambda: tuple(islice(lst, chunk_size)), ())
for new_list in group_elements(test_list , 3):
print(new_list)
输出:
('1', '2', '3')
('4', '5', '6')
('7', '8', '9')
('10',)
在 Python 中使用 NumPy
方法将列表拆分为块
NumPy
库也可以用来将列表分成 N 个大小的块。array_split()
函数将数组划分为特定大小 n
的子数组。
完整的示例代码如下:
import numpy
n = numpy.arange(11)
final_list = numpy.array_split(n,4);
print("The Final List is:", final_list)
arange
函数根据给定的参数对数值进行排序,array_split()
函数根据给定的参数生成列表/数组。
输出:
The Final List is: [array([0, 1, 2]), array([3, 4, 5]), array([6, 7, 8]), array([ 9, 10])]
在 Python 中使用用户定义的函数将列表拆分成块
此方法将遍历列表并产生连续的 n 大小的块,其中 n 指的是需要执行拆分的数量。在这个函数中使用了一个关键字 yield
,它允许一个函数在暂停执行时,随着值的转动而停止和恢复。这是与普通函数的重要区别。普通函数不能返回到它离开的地方。当我们在函数中使用 yield
语句时,这个函数被称为生成器。生成器产生或返回值,不能命名为一个简单的函数,而是命名为一个可迭代的函数,即使用循环。
完整的示例代码如下。
test_list = ['1','2','3','4','5','6','7','8','9','10']
def split_list(lst, n):
for i in range(0, len(lst), n):
yield lst[i:i + n]
n = 3
output = list(split_list(test_list, n))
print(output)
输出:
[['1', '2', '3'], ['4', '5', '6'], ['7', '8', '9'], ['10']]