在 Python 中运行 Bash 命令
Muhammad Waiz Khan
2023年1月30日
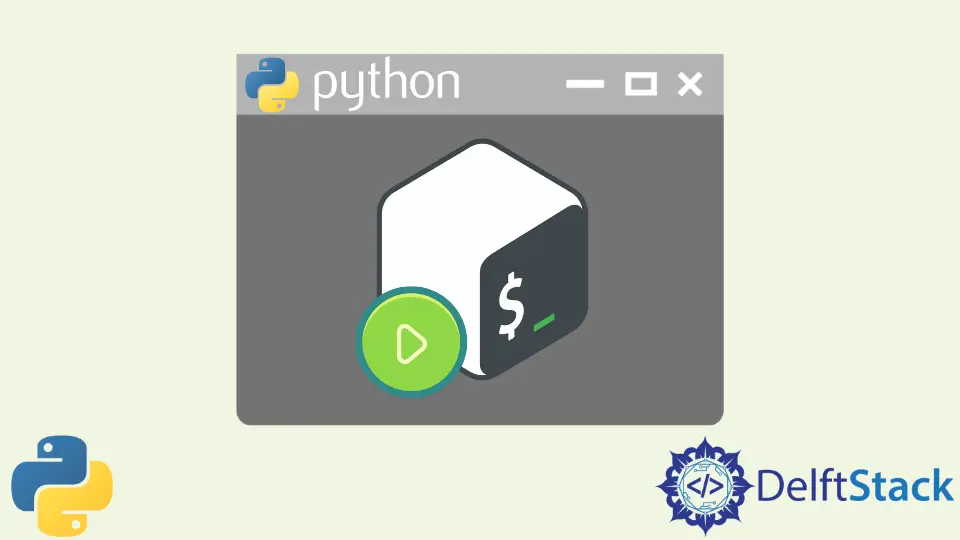
本教程将解释在 Python 中运行 bash 命令的各种方法。Bash 是 Linux 和 Unix 操作系统中使用的 shell 或命令语言解释器。而 bash 命令是用户向操作系统发出的执行特定任务的指令,如 cd
命令改变当前目录,mkd
命令新建目录,ls
命令列出目录中的文件和子目录等。
在 Python 中使用 subprocess
模块的 run()
方法运行 Bash 命令
subprocess
模块的 run()
方法接受以字符串形式传来的命令,为了获得输出或命令的输出错误,我们应该将 stdout
参数和 stderr
参数设置为 PIPE
。run
方法返回一个完成的进程,包含 stdout
、stderr
和 returncode
作为属性。
该代码示例代码演示了如何使用 run()
方法在 Python 中运行 bash 命令。
from subprocess import PIPE
comp_process = subprocess.run("ls", stdout=PIPE, stderr=PIPE)
print(comp_process.stdout)
在 Python 中使用 subprocess
模块的 Popen()
方法运行 Bash 命令
subprocess
模块中的 Popen()
方法与 run()
方法功能类似,但使用起来比较麻烦。Popen()
方法与 run()
方法不同,它不返回一个已完成的进程对象作为输出,而且 Popen()
方法启动的进程需要单独处理,使用起来比较棘手。
Popen()
方法返回的不是已完成的进程,而是一个进程对象作为输出。返回的进程需要使用 process.kill()
或 process.terminate()
方法来杀死。
和 run()
方法一样,我们需要设置 Popen()
的 stdout
和 stderr
参数来获取命令的输出和错误。而输出和错误可以通过返回的进程对象使用 process.communicate
方法进行访问。
下面的代码示例演示了如何使用 Popen()
方法运行 bash 命令,以及如何在 Python 中获取 stdout
和 stderr
值,然后杀死进程。
from subprocess import PIPE
process = subprocess.Popen("ls", stdout=PIPE, stderr=PIPE)
output, error = process.communicate()
print(output)
process.kill