Python 中的并行 for 循环
Shivam Arora
2023年1月30日
2021年7月10日
-
在 Python 中使用
multiprocessing
模块并行化for
循环 -
在 Python 中使用
joblib
模块并行化for
循环 -
在 Python 中使用
asyncio
模块并行化for
循环
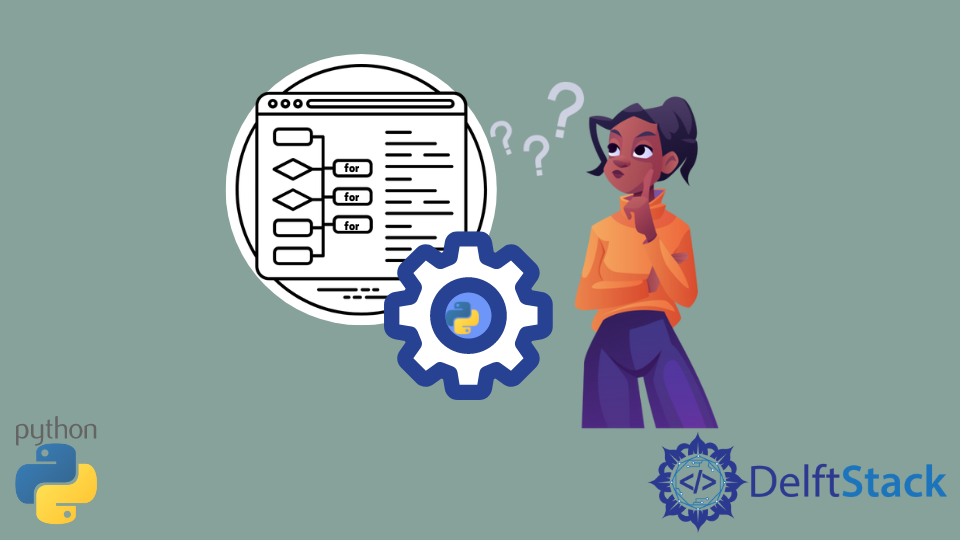
并行化循环意味着使用多个内核并行扩展所有进程。当我们有大量作业时,每个计算都不会等待并行处理中的前一个计算完成。相反,它使用不同的处理器来完成。
在本文中,我们将在 Python 中并行化 for
循环。
在 Python 中使用 multiprocessing
模块并行化 for
循环
为了并行化循环,我们可以使用 Python 中的 multiprocessing
包,因为它支持根据另一个正在进行的进程的请求创建子进程。
可以使用 multiprocessing
模块代替 for 循环来对可迭代对象的每个元素执行操作。可以使用 multiprocessing.pool()
对象,因为在 Python 中使用多线程不会因为全局解释器锁而给出更好的结果。
例如,
import multiprocessing
def sumall(value):
return sum(range(1, value + 1))
pool_obj = multiprocessing.Pool()
answer = pool_obj.map(sumall,range(0,5))
print(answer)
输出:
0, 1, 3, 6, 10
在 Python 中使用 joblib
模块并行化 for
循环
joblib
模块使用多处理来运行多个 CPU 内核来执行 for
循环的并行化。它提供了一个轻量级的管道,可以记住模式以进行简单直接的并行计算。
要执行并行处理,我们必须设置作业数,而作业数受限于 CPU 中的内核数或当前可用或空闲的内核数。
delayed()
函数允许我们告诉 Python 在一段时间后调用特定的提到的方法。
Parallel()
函数创建一个具有指定内核的并行实例(在本例中为 2)。
我们需要为代码的执行创建一个列表。然后将列表传递给并行,并行开发两个线程并将任务列表分发给它们。
请参考下面的代码。
from joblib import Parallel, delayed
import math
def sqrt_func(i, j):
time.sleep(1)
return math.sqrt(i**j)
Parallel(n_jobs=2)(delayed(sqrt_func)(i, j) for i in range(5) for j in range(2))
输出:
[1.0,
0.0,
1.0,
1.0,
1.0,
1.4142135623730951,
1.0,
1.7320508075688772,
1.0,
2.0]
在 Python 中使用 asyncio
模块并行化 for
循环
asyncio
模块是单线程的,通过使用 yield from
或 await
方法暂时挂起协程来运行事件循环。
下面的代码会在被调用时并行执行,而不会影响要等待的主函数。该循环还与主函数并行运行。
import asyncio
import time
def background(f):
def wrapped(*args, **kwargs):
return asyncio.get_event_loop().run_in_executor(None, f, *args, **kwargs)
return wrapped
@background
def your_function(argument):
time.sleep(2)
print('function finished for '+str(argument))
for i in range(10):
your_function(i)
print('loop finished')
输出:
ended execution for 4
ended execution for 8
ended execution for 0
ended execution for 3
ended execution for 6
ended execution for 2
ended execution for 5
ended execution for 7
ended execution for 9
ended execution for 1