修复 Python 字典中的 KeyError
Vaibhav Vaibhav
2022年5月17日
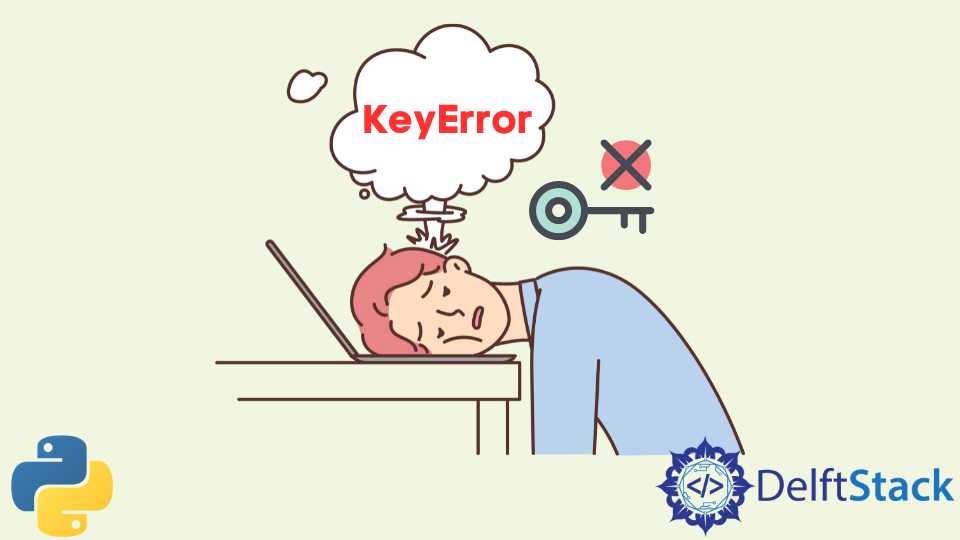
字典是 Python 中可用的可扩展数据结构。它以键值对的形式存储数据,其中键可以是任何可散列和不可变的对象,值可以是任何东西;一个列表、一个元组、一个字典、一个对象列表等等。
使用键,我们可以访问这些键指向的值。如果给字典一个不存在的键,它会抛出一个 KeyError
异常。在本文中,我们将学习如何在 Python 中处理此异常。
修复 Python 字典中的 KeyError
异常
要解决 KeyError
异常,可以在访问之前检查字典中是否存在该键。keys()
方法返回字典中的键列表。在访问某个键的值之前,如果你不确定该键是否存在,建议你检查该键是否存在于此列表中。以下 Python 代码描述了相同的内容。
data = {
"a": 101,
"b": 201,
"c": 301,
"d": 401,
"e": 501,
}
keys = ["a", "e", "r", "f", "c"]
for key in keys:
if key in data.keys():
print(data[key])
else:
print(f"'{key}' not found.")
输出:
101
501
'r' not found.
'f' not found.
301
除了上面讨论的方法之外,还可以使用 try...except
块来捕获 KeyError
异常或任何异常。请参阅以下 Python 代码。
data = {
"a": 101,
"b": 201,
"c": 301,
"d": 401,
"e": 501,
}
keys = ["a", "e", "r", "f", "c"]
for key in keys:
try:
print(data[key])
except:
print(f"'{key}' not found.")
输出:
101
501
'r' not found.
'f' not found.
301
如果发生 KeyError
异常,except
块下的代码将被执行。
Author: Vaibhav Vaibhav