在 Python 字典中寻找最大值
-
使用
operator.itemgetter()
方法获取具有最大值的键 -
在 Python 3.x 中使用
dict.items()
方法来获取字典中的最大值的键 - 获取字典中最大值的键的通用和高内存效率的解决方案
-
使用
max()
和dict.get()
方法来获取字典中最大值的键
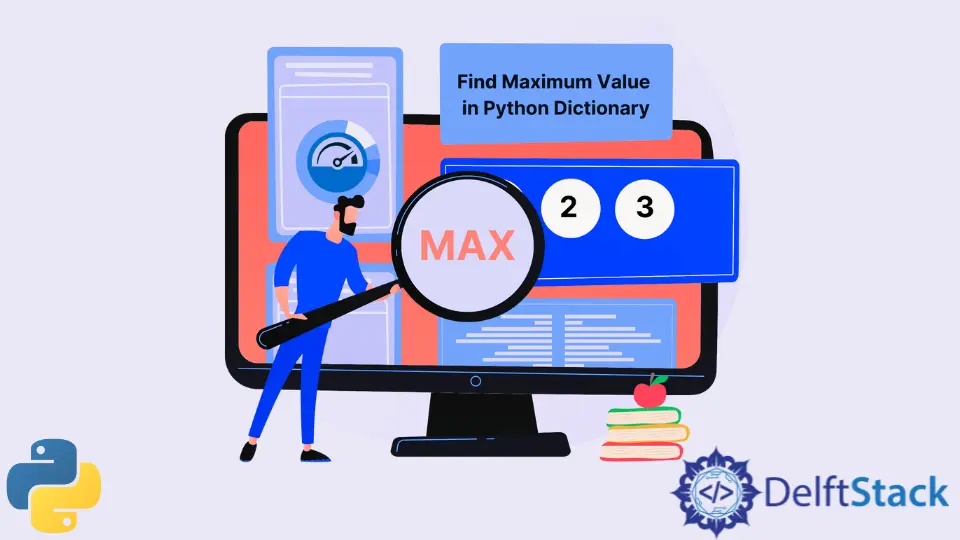
本教程介绍了如何在 Python 中获取一个具有最大值的键。由于该方法与以前的 Python 版本相比已经发生了变化,因此它还列出了一些示例代码来澄清概念。
使用 operator.itemgetter()
方法获取具有最大值的键
我们不需要创建一个新的列表来迭代字典的 (key, value)
对。我们可以使用 stats.iteritems()
来实现这个目的。它在字典的 (key, value)
对上返回一个迭代器。
你可以使用 operator.itemgetter(x)
方法来获得可调用的对象,它将从对象中返回索引为 x
的元素。这里因为对象是 (key, value)
对,所以 operator.itemgetter(1)
指的是 1
索引处的元素,也就是 value
。
由于我们想要一个具有最大值的键,所以我们将把方法封装在 max
函数中。
下面给出了这个方法的基本示例代码。
import operator
stats = {"key1": 20, "key2": 35, "key3": 44}
max_key = max(stats.iteritems(), key=operator.itemgetter(1))[0]
print(max_key)
输出:
key3
另外,请注意,iteritems()
在从字典中添加或删除项时可能会引发 RunTimeException
,而 dict.iteritems
在 Python 3 中被删除。
在 Python 3.x 中使用 dict.items()
方法来获取字典中的最大值的键
在 Python 3.x 中,你可以使用 dict.items()
方法来迭代字典中的 key-value
对。它与 Python 2 中的 dict.iteritems()
方法相同。
下面给出了这个方法的示例代码。
import operator
stats = {"key1": 20, "key2": 35, "key3": 44}
max_key = max(stats.items(), key=operator.itemgetter(1))[0]
print(max_key)
输出:
key3
获取字典中最大值的键的通用和高内存效率的解决方案
有趣的是,有另一种解决方案对 Python 2 和 Python 3 都适用。该方案使用 lambda
函数来获取 key,并使用 max
方法来确保获取的 key
是最大的。
下面给出了这种方法的代码。
import operator
stats = {"key1": 20, "key2": 35, "key3": 44}
max_key = max(stats, key=lambda key: stats[key])
print(max_key)
输出:
key3
使用 max()
和 dict.get()
方法来获取字典中最大值的键
解决这个问题的另一个方法可以简单地使用内置的 max()
方法。它提供了 stats
来获取最大值,为了返回具有最大值的 key
,使用 dict.get()
方法。
下面给出一个示例代码。
import operator
stats = {"key1": 20, "key2": 35, "key3": 44}
max_key = max(stats, key=stats.get)
print(max_key)
输出:
key3
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn