在 Python 中使用 fetchall() 从数据库中提取元素
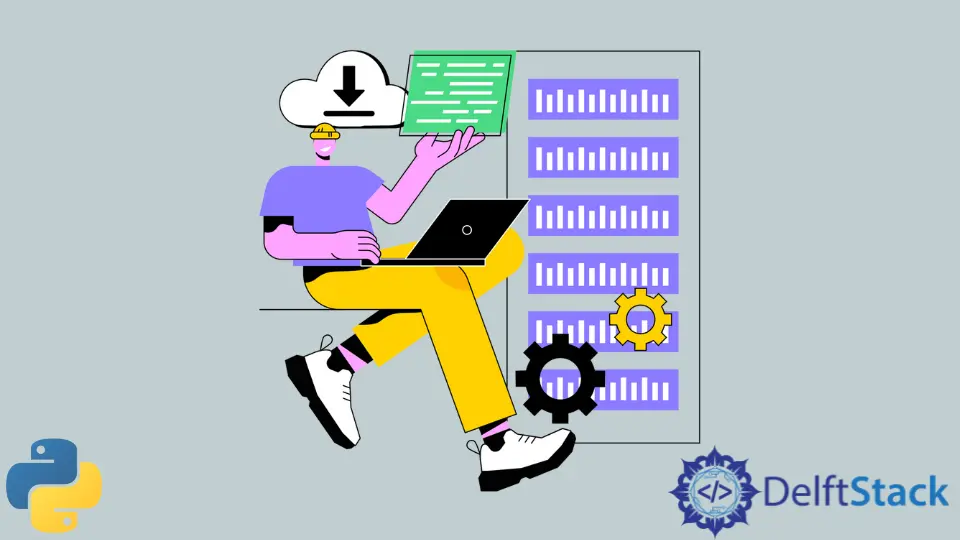
本文旨在介绍使用 fetchall()
从数据库中提取元素的工作方法以及如何正确显示它们。本文还将讨论 list(cursor)
函数如何在程序中使用。
在 Python 中使用 fetchall()
从数据库文件中提取元素
该程序将与扩展名为 .db 的数据库文件建立安全 SQL 连接。建立连接后,程序将获取存储在该数据库表中的数据。
由于它是一个使用 fetchall()
提取元素的程序,因此将使用 for
循环提取和显示数据。
导入 Sqlite3 并与数据库建立连接
sqlite3 是 Python 中用于访问数据库的导入包。它是一个内置包;它不需要安装额外的软件即可使用,可以使用 import sqlite3
直接导入。
该程序在加载数据库时使用 try
块来测试错误,并在使用 exception
块建立连接时抛出错误消息。最后,程序关闭与 finally
块的连接。
但是,在了解如何使用 fetchall()
检索项目之前,首先必须了解 SQLite 是如何建立连接的。该程序声明了一个方法 allrowsfetched()
,在其中插入了 try
块并声明了一个变量 database_connecter
。
该变量将与数据库建立连接并加载其内容,如下面的代码片段所示。
import sqlite3
def allrowsfetched():
try:
database = sqlite3.connect("samplefile.db")
cursorfordatabase = database.cursor()
print("Connection is established")
except Exception as e:
print(e)
建立连接后,需要为数据库创建游标,这是一种帮助使用 Python 为 SQL 数据库执行命令的连接器。
在上面的程序中,游标是使用语法 database.cursor()
创建并存储在变量 cursorfordatabase
中的。如果上述所有步骤都正确执行,程序将打印一条成功消息。
为使用 fetchall()
方法创建游标对象
要使用 fetchall()
提取元素,我们必须确定数据库内容。程序中使用的数据库中存储了多个表。
该程序需要专门提取一个名为 employees
的表。它必须生成一个查询:
- 使用语法
SELECT * from table_name
生成查询。在程序中,查询是为了从数据库中找到一个名为employees
的表,它存储在变量query_for_sqlite
中。 - 生成查询后,
cursor.execute()
方法对数据库执行该查询。 - 最后,
cursor.fetchall()
语法使用fetchall()
提取元素,并将特定表加载到游标内并将数据存储在变量required_records
中。 - 变量
required_records
存储整个表本身,因此返回该变量的长度提供表内的行数。 - 使用
len(required_records)
语法打印行数。
query_for_sqlite = """SELECT * from employees"""
cursorfordatabase.execute(query_for_sqlite)
required_records = cursorfordatabase.fetchall()
print("Rows Present in the database: ", len(required_records))
使用 for
循环显示行元素
在使用 fetchall()
提取元素的步骤启动后,程序使用 for
循环来打印元素。for
循环运行的次数是行在变量 required_records
中出现的次数。
在此内部,使用行的索引打印各个元素。在这个数据库中,有 8 行(索引计数从 0 开始,到 7 结束)。
print("Data in an ordered list")
for row in required_records:
print("Id: ", row[0])
print("Last Name: ", row[1])
print("First Name ", row[2])
print("Title: ", row[3])
print("Reports to: ", row[4])
print("dob: ", row[5])
print("Hire-date: ", row[6])
print("Address: ", row[7])
print("\n")
处理异常
一旦实现了程序的目的,即使用 fetchall()
提取元素,则需要从内存中释放游标和连接变量中加载的数据。
- 首先,我们使用
cursor.close()
语法来释放游标变量cursorfordatabase
中存储的内存。 - 然后程序需要说明异常处理,即程序的
except
和finally
块,紧跟在try
块之后。 except
块用于 sqlite3 错误。因此,当未与数据库建立连接时,程序会显示错误消息,而不是在运行时崩溃。finally
块最后执行,在执行两个块中的一个后,try
或except
。它关闭 SQLite 连接并打印相关消息。
finally
块的执行发生在最后,无论哪个块在它之前执行,为程序提供了一个关闭的姿态。
cursorfordatabase.close()
except sqlite3.Error as error:
print("Failed to read data from table", error)
finally:
if database:
database.close()
print("Connection closed")
用 Python 从数据库文件中提取元素的完整代码
下面提供了该程序的工作代码,以更好地理解这些概念。
import sqlite3
def allrowsfetched():
try:
database = sqlite3.connect("samplefile.db")
cursorfordatabase = database.cursor()
print("Connection established")
query_for_samplefile = """SELECT * from employees"""
cursorfordatabase.execute(query_for_samplefile)
required_records = cursorfordatabase.fetchall()
print("Rows Present in the database: ", len(required_records))
print("Data in an ordered list")
print(required_records)
for row in required_records:
print("Id: ", row[0])
print("Last Name: ", row[1])
print("First Name ", row[2])
print("Title: ", row[3])
print("Reports to: ", row[4])
print("dob: ", row[5])
print("Hired on: ", row[6])
print("Address: ", row[7])
print("\n")
cursorfordatabase.close()
except sqlite3.Error as error:
print("Failed to read data from table,", error)
finally:
if database:
database.close()
print("The Sqlite connection is closed")
allrowsfetched()
输出:当成功找到表时,
"C:/Users/Win 10/main.py"
Connection established
Rows Present in the database: 8
Data in an ordered list
Id: 1
Last Name: Adams
First Name Andrew
Title: General Manager
Reports to: None
Birthdate: 1962-02-18 00:00:00
Hire-date: 2002-08-14 00:00:00
Address: 11120 Jasper Ave NW
.
.
.
Connection closed
Process finished with exit code 0
输出:当所需的表不存在时,
"C:/Users/Win 10/main.py"
Connection established
Failed to read data from table, no such table: salary
Connection closed
Process finished with exit code 0
在这里,错误是通过使用表名 salary
作为查询创建的,例如 query_for_samplefile = """SELECT * from salary"""
。
在 Python 中使用 list(cursor)
作为从数据库中提取元素的替代方法
使用 fetchall()
提取元素的方法已经讨论到现在,尽管还有其他方法,例如 fetchone()
和 fetchmany()
。
我们也可以在不使用 fetch()
方法的情况下提取元素;相反,我们可以使用 list(cursor)
。这个过程就像 fetchall()
一样提取所有元素。
该方法节省了内存占用。与加载整个表的 fetchall()
不同,list(cursor)
运行一个循环,连续提取元素,然后从数据库中打印它们而不将它们存储在任何地方。
下面的代码让我们了解如何使用它。
所有步骤都类似于上面的程序,除了没有使用 fetchall()
初始化新变量来存储表。游标 cursorfordatabase
被放入 for
循环中,并打印该行。
由于游标对象仅存储查询,因此在内存占用中占用的空间最少甚至没有。
query_for_sqlite = """SELECT * from employees"""
cursorfordatabase.execute(query_for_sqlite)
for row in cursorfordatabase:
print("\n", row)
索引还可以获取有序列表,就像上一个程序一样。
for row in cursorfordatabase:
print("id:", row[0])
print("l_name:", row[1])
结论
本文重点展示如何在 Python 程序中使用 fetchall()
提取元素。你已经学习了诸如 cursor()
之类的概念和诸如 cursor.execute()
、sqlite3.connect
之类的语法函数,以及处理异常块。
你还了解了 list(cursor)
方法以及它如何成为从数据库中提取元素的替代方法。