在 Python 中将 CSV 文件转换为 JSON 文件
-
在 Python 中使用
json.dump()
方法将 CSV 文件转换为 JSON 文件 -
在 Python 中使用
Dataframe.to_json()
方法将 CSV 文件转换为 JSON 文件
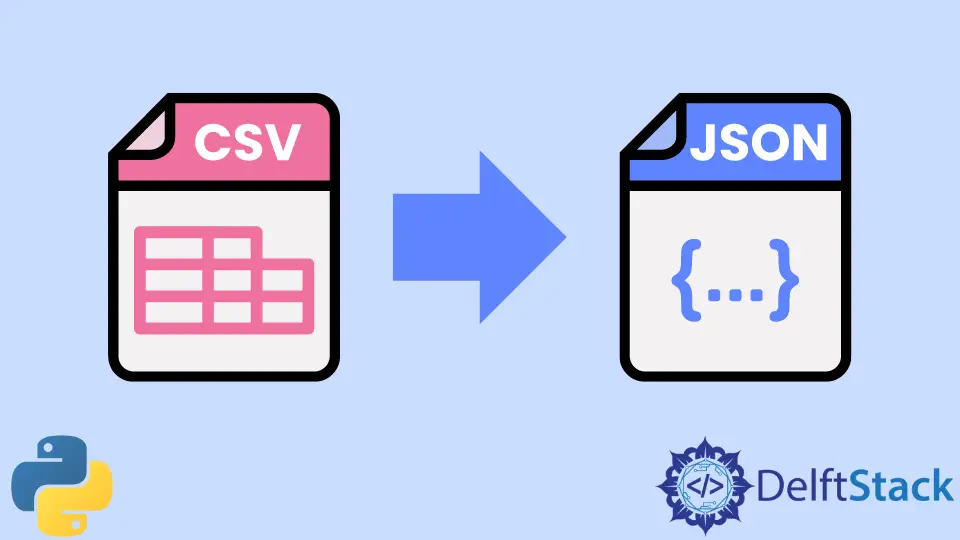
本教程将演示用 Python 从 CSV 文件中读取数据并保存为 JSON 文件的各种方法。在 Web 应用程序中,用于保存和传输数据的格式是 JSON 格式。假设我们已将数据保存为 CSV(逗号分隔值)格式,并且需要将其转换为 JSON 格式。
因此,我们需要一些方法将 CSV 格式的数据转换为 JSON 格式。我们可以使用以下方法在 Python 中将 CSV 文件转换为 JSON 文件。
在 Python 中使用 json.dump()
方法将 CSV 文件转换为 JSON 文件
json.dump(obj, fp, indent=None, Seperator=None)
方法将数据 obj
用作输入,并将 obj
序列化为 JSON 格式的流,并将其写入类似文件的对象 fp
中。
如果我们想将缩进添加到数据中以使其更易于阅读,则可以使用 indent
关键字参数。对于 indent
参数值等于 0
时,方法在每个值后增加一个新行,并在每行开始时增加 indent
的数量,即 indent
等于 1
时增加一个\t
,以此类推。
如果 indent
参数是 None
,则 separator
参数等于 (', ', ': ')
,否则等于 (', ', ': ')
。
以下示例代码演示了如何使用 json.dump()
方法在 Python 中将数据另存为 JSON 文件。
with open("file.csv", "r") as file_csv:
fieldnames = ("field1", "field2")
reader = csv.DictReader(file_csv, fieldnames)
with open("myfile.json", "w") as file_json:
for row in reader:
json.dump(row, file_json)
在 Python 中使用 Dataframe.to_json()
方法将 CSV 文件转换为 JSON 文件
Pandas
模块的 Dataframe.to_json(path, orient)
方法,以 DataFrame
和 path
作为输入并将其转换为 JSON 字符串,并保存在提供的 path
中。如果未提供 path
,则该方法返回 JSON 字符串作为输出,如果提供 path
,则该方法不返回任何内容。
orient
参数对于指定我们如何格式化 JSON 字符串非常有用,并且对于 Series
和 DataFrame
输入都有各种选项。
由于 Dataframe.to_json()
方法将 DataFrame
作为输入,因此我们将使用 pandas.readcsv()
方法首先将 CSV 文件读取为 DataFrame
。以下示例代码演示了如何使用 Dataframe.to_json()
方法在 Python 中将 CSV 文件转换为 JSON 文件。
import pandas as pd
csv_data = pd.read_csv("test.csv", sep=",")
csv_data.to_json("test.json", orient="records")
相关文章 - Python CSV
- Python 逐行写入 CSV
- 使用 Python 将 XML 转换为 CSV
- 在 Python 中将列表写入 CSV 列
- 在 Python 中逐行读取 CSV
- 在 Python 中读取带有标题的 CSV
- 在 Python 中合并 CSV 文件