Python 计算目录中的文件数
-
在 Python 中使用
pathlib
模块的pathlib.Path.iterdir()
函数计算目录中的文件数 -
在 Python 中使用
os
模块的listdir()
方法计算目录中的文件数
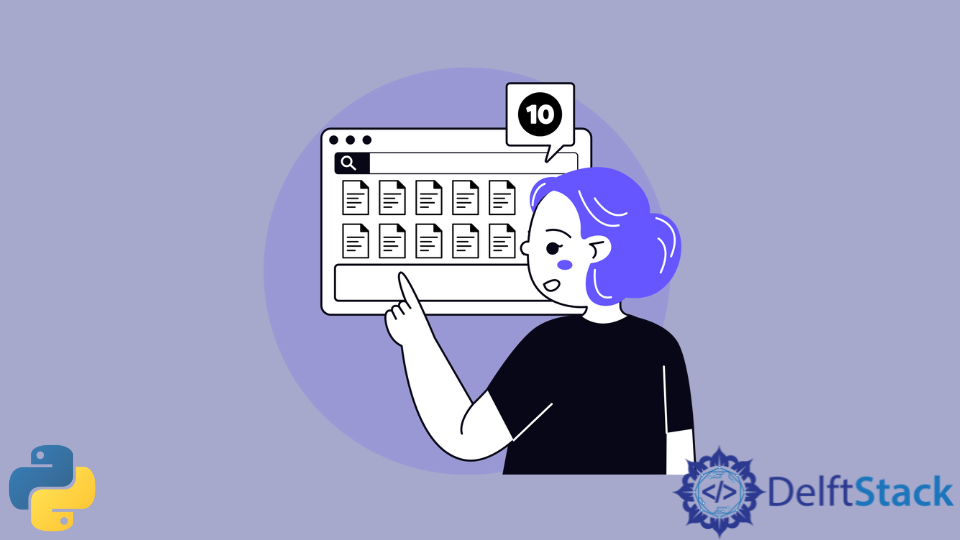
在 Python 中,每当有人需要处理文件并对其执行外部操作时,工作目录总是牢记在心。如果没有设置所需文件所在的正确工作目录,用户将无法对该文件执行任何操作。可能存在用户需要知道特定目录中存在多少文件的情况。
本教程向你展示了如何在 Python 中计算目录中文件数的方法。
在 Python 中使用 pathlib
模块的 pathlib.Path.iterdir()
函数计算目录中的文件数
pathlib
模块属于 Python 的标准实用程序模块。该模块通过提供各种表示外部文件路径的类和对象来帮助用户,并以适当的方法与操作系统交互。
pathlib
模块的 pathlib.Path.iterdir()
用于在 Python 中获取目录内容的路径对象;只要目录的路径已知,就会执行此操作。
import pathlib
initial_count = 0
for path in pathlib.Path(".").iterdir():
if path.is_file():
initial_count += 1
print(initial_count)
在上面的示例中,还使用了 path.is_file()
函数。它也是 pathlib
模块的一个命令,用于检查路径是否以文件结尾。
单独使用时,此函数返回一个布尔值。所以在这里,如果路径指向一个文件,initial_count
增加一。
在 Python 中使用 os
模块的 listdir()
方法计算目录中的文件数
os
模块也属于 Python 的标准实用程序模块。它提供了各种方法或功能,在用户与操作系统交互时非常有用。
os
模块的方法之一是 listdir()
方法。此方法返回所提到的特定目录中存在的所有文件的列表。默认情况下,如果用户未提及目录,则返回当前工作目录中的文件和目录列表。
import os
initial_count = 0
dir = "RandomDirectory"
for path in os.listdir(dir):
if os.path.isfile(os.path.join(dir, path)):
initial_count += 1
print(initial_count)
请注意,在上面的代码中,指定了一个目录。因此,返回的输出将是该特定目录中存在的文件和目录的数量,而没有其他目录。
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn