在 Python 中连接字符串和整数值
-
在 Python 中使用
str()
函数实现字符串和整数连接 -
在 Python 中使用带有模
%
符号的字符串格式进行字符串和整数连接 -
在 Python 中将字符串格式与
str.format()
函数用于字符串和整数连接 -
在 Python 中使用
f-string
进行字符串格式化
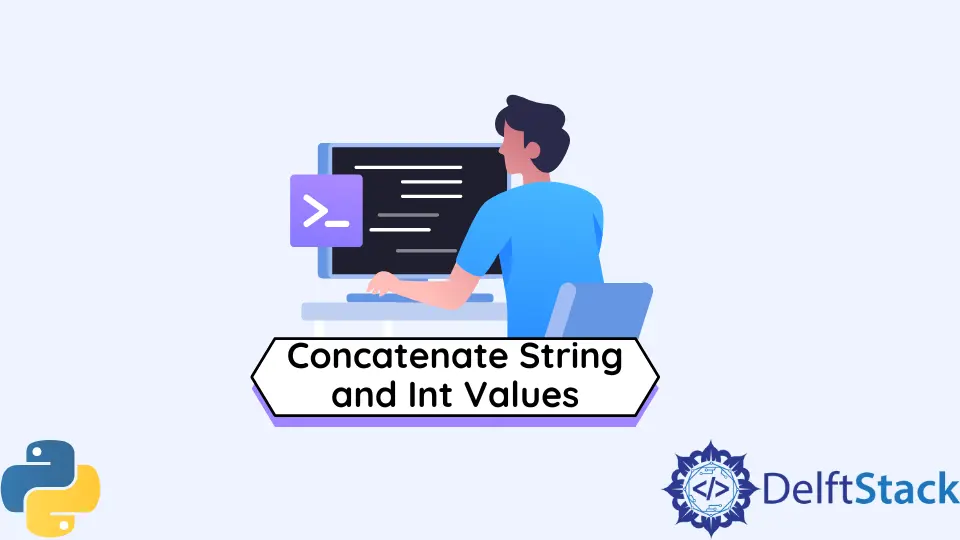
连接可以定义为将两个字符串集成到一个对象中。在 Python 中,你可以使用 +
运算符执行连接。在这里,我们将讨论如何在 Python 中成功实现字符串和整数连接。
在大多数编程语言中,你经常会遇到这样的操作:如果要在字符串和整数之间进行连接过程,语言会自动先将整数值转换为字符串值,然后再继续进行字符串连接过程。
Python 是此操作的一个例外,如果将字符串与整数连接,则会引发错误。
以下代码尝试在 Python 中实现字符串和整数连接。
x = "My crypto portfolio amount in dollars is "
y = 5000
print(x + y)
输出:
Traceback (most recent call last):
File "<string>", line 3, in <module>
TypeError: can only concatenate str (not "int") to str
从上面的代码可以看出,在 Python 编程语言中,字符串和整数的直接连接是不可能的。
在本指南的以下部分中,我们将重点介绍可以成功实现整数和字符串连接的不同方法。
在 Python 中使用 str()
函数实现字符串和整数连接
成功实现字符串和整数之间的连接的最简单和最简单的方法是使用 str()
函数手动将整数值转换为字符串值。
以下代码使用 str()
函数在 Python 中实现字符串和整数连接。
x = "My crypto portfolio amount in dollars is "
y = 5000
print(x + str(y))
输出:
My crypto portfolio amount in dollars is 5000
在 Python 中使用带有模 %
符号的字符串格式进行字符串和整数连接
字符串格式提供了多种自定义选项供用户在 print
语句中进行选择。%
符号有时也称为插值或字符串格式化运算符。
有很多方法可以实现字符串格式化,%
符号是最古老的可用方法,几乎适用于所有版本的 Python。
%
符号和代表转换类型的字母被标记为变量的占位符。以下代码使用模 %
符号在 Python 中实现字符串和整数连接。
x = "My crypto portfolio amount in dollars is "
y = 5000
print("%s%s" % (x, y))
输出:
My crypto portfolio amount in dollars is 5000
在 Python 中将字符串格式与 str.format()
函数用于字符串和整数连接
这种方法是另一种实现字符串格式化的方法,其中括号 {}
标记了 print
语句中需要替换变量的位置。
str.format()
函数是在 Python 2.6 中引入的,可用于 Python 2.6 到 Python 3.5 之后发布的所有 Python 版本。
以下代码使用 str.format()
函数在 Python 中实现字符串和整数连接。
x = "My crypto portfolio amount in dollars is "
y = 5000
print("{}{}".format(x, y))
输出:
My crypto portfolio amount in dollars is 5000
在 Python 中使用 f-string
进行字符串格式化
这种方法是 Python 中实现字符串格式化的最新方法。它是在 Python 3.6 中引入的,可用于更新和最新版本的 Python。
它比其他两个同行,%
符号和 str.format()
更快更容易,在 Python 中实现字符串格式时效率更高并且具有速度优势。
以下代码使用 fstring
格式在 Python 中实现字符串和整数连接。
x = "My crypto portfolio amount in dollars is "
y = 5000
print(f"{x}{y}")
输出:
My crypto portfolio amount in dollars is 5000
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn相关文章 - Python String
- 在 Python 中从字符串中删除逗号
- 如何用 Pythonic 的方式来检查字符串是否为空
- 在 Python 中将字符串转换为变量名
- Python 如何去掉字符串中的空格/空白符
- 如何在 Python 中从字符串中提取数字
- Python 如何将字符串转换为时间日期 datetime 格式