在 Python 中计算累积分布函数
Najwa Riyaz
2023年1月30日
2021年7月9日
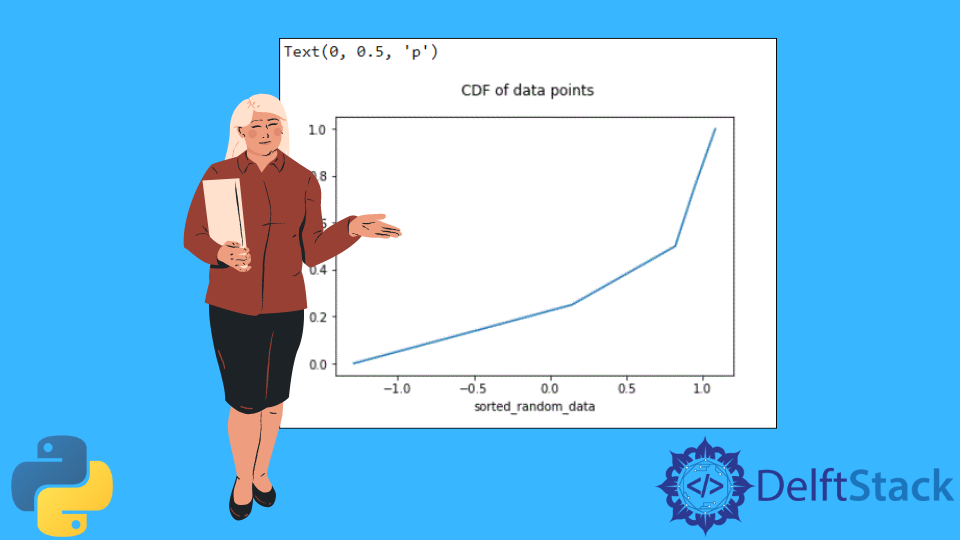
术语累积分布函数或 CDF
是一个函数 y=f(x)
,其中 y
表示整数 x
或任何低于 x
的数字从分布中随机选择的概率。
它是通过使用 NumPy
库中的以下函数在 Python 中计算的。
numpy.arange()
函数返回一个ndarray
的均匀间隔值。numpy.linspace()
函数返回给定间隔内均匀间隔值的ndarray
。
在 Python 中使用 numpy.arange()
计算 CDF
NumPy
标准库包含用于在 Python 中确定 CDF 的 arange()
函数。
为此,首先导入 NumPy
库。
arange()
函数返回一个由均匀间隔的值组成的 ndarray
。
下面的示例演示了使用 Python 中的 numpy.arange()
函数实现 CDF 函数。
import matplotlib.pyplot as plt
import numpy
data = numpy.random.randn(5)
print("The data is-",data)
sorted_random_data = numpy.sort(data)
p = 1. * numpy.arange(len(sorted_random_data)) / float(len(sorted_random_data) - 1)
print("The CDF result is-",p)
fig = plt.figure()
fig.suptitle('CDF of data points')
ax2 = fig.add_subplot(111)
ax2.plot(sorted_random_data, p)
ax2.set_xlabel('sorted_random_data')
ax2.set_ylabel('p')
在这里,randn()
函数用于返回使用标准正态分布的数据样本。由于提到了 randn(5)
,因此使用 5 个随机值构建了一个 1Darray。
接下来,使用 sort()
函数对数据进行排序,然后使用 arange()
函数计算 CDF。
输出 :
The data is- [ 0.14213322 -1.28760908 0.94533922 0.82004319 1.08232731]
The CDF result is- [0. 0.25 0.5 0.75 1. ]
图形按照 CDF 函数显示。
在 Python 中使用 numpy.linspace()
计算 CDF
NumPy
标准库包含用于在 Python 中确定 CDF 的 linspace()
函数。为此,首先导入 NumPy
库。
linspace()
函数返回指定间隔内均匀间隔数字的 ndarray
。
这是一个示例,演示了在 Python 中使用 numpy.linspace()
实现 CDF 函数。
import matplotlib.pyplot as plt
import numpy as np
data = np.random.randn(5)
print("The data is-",data)
sorted_random_data = np.sort(data)
np.linspace(0, 1, len(data), endpoint=False)
print("The CDF result using linspace =\n",p)
fig = plt.figure()
fig.suptitle('CDF of data points')
ax2 = fig.add_subplot(111)
ax2.plot(sorted_random_data, p)
ax2.set_xlabel('sorted_random_data')
ax2.set_ylabel('p')
在这里,randn()
函数用于返回使用标准正态分布的数据样本。接下来,使用 sort()
函数对数据进行排序,然后使用 arange()
函数计算 CDF。
输出:
The data is- [-0.92106668 -0.05998132 0.02102705 -0.84778184 0.90815869]
The CDF result using linspace =
[0. 0.25 0.5 0.75 1. ]
图表按照 CDF 函数显示,如下所示。