将两个 Pandas 系列合并到一个 DataFrame 中
-
使用
pandas.concat()
方法将两个 Pandas 系列合并为一个DataFrame
-
使用
pandas.merge()
方法将两个 Pandas 系列合并到一个DataFrame
中 -
使用
Series.append()
方法将两个 Pandas 系列合并到一个DataFrame
中 -
使用
DataFrame.join()
方法将两个 Pandas 系列合并为一个DataFrame
- 结论
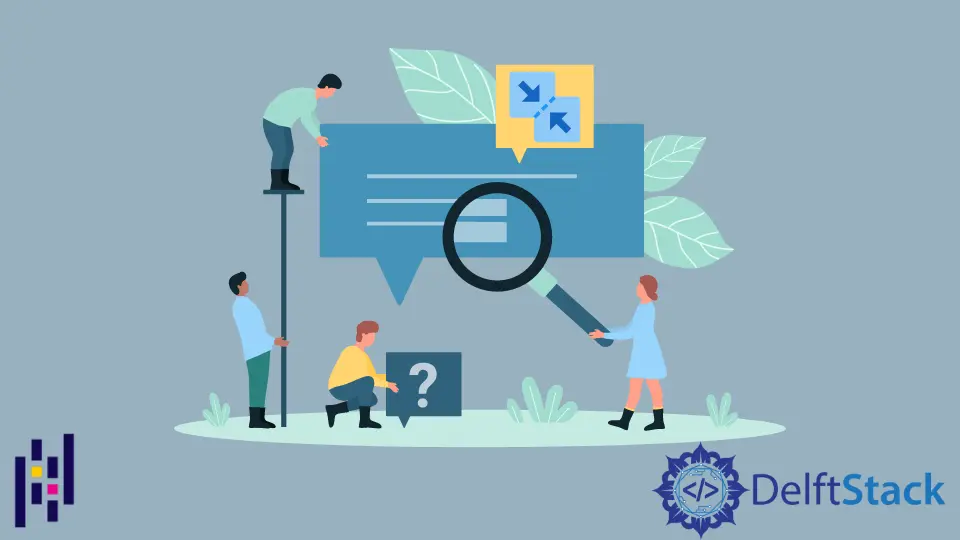
Pandas 是一个非常流行的开源 Python 库,它提供了各种功能或方法来合并或组合 DataFrame
中的两个 Pandas 系列。在 pandas 中,series 是一个单一的一维标签数组,可以处理整数、浮点数、字符串、python 对象等任何数据类型。简单来说,pandas 系列就是 excel 表格中的一列。系列以顺序
顺序存储数据。
本教程将教我们如何将两个或多个 Pandas 系列合并或组合成一个 DataFrame
。
有几种方法可以将两个或多个 Pandas 系列合并到一个 DataFrame
,例如 pandas.concat()
、Series.append()
、pandas.merge()
和 DataFrame.join()
。我们将借助本文中的一些示例简要详细地解释每种方法。
使用 pandas.concat()
方法将两个 Pandas 系列合并为一个 DataFrame
pandas.concat()
方法沿轴(row-wise
或 column-wise
)执行所有连接操作。我们可以沿特定轴合并两个或多个 Pandas 对象或系列以创建 DataFrame
。concat()
方法采用各种参数。
在下面的示例中,我们将 pandas series
传递给 merge 和 axis=1
作为参数。axis=1
表示该系列将合并为一列而不是行。如果我们使用 axis=0
,它会将 pandas 系列附加为一行。
示例代码:
import pandas as pd
# Create Series by assigning names
products = pd.Series(
["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
name="Products",
)
dollar_price = pd.Series([350, 300, 400, 250], name="Price")
percentage_sale = pd.Series([83, 99, 84, 76], name="Sale")
# merge two pandas series using the pandas.concat() method
df = pd.concat([products, dollar_price, percentage_sale], axis=1)
print(df)
输出:
Products Price Sale
0 Intel Dell Laptops 350 83
1 HP Laptops 300 99
2 Lenavo Laptops 400 84
3 Acer Laptops 250 76
使用 pandas.merge()
方法将两个 Pandas 系列合并到一个 DataFrame
中
pandas.merge()
用于合并 DataFrame
的复杂列组合,类似于 SQL join
或 merge
操作。merge()
方法可以执行命名系列对象或 DataFrame
之间的所有数据库连接操作。使用 pandas 时,我们必须向系列传递一个额外的参数
name。合并()
方法。
请参阅以下示例。
示例代码:
import pandas as pd
# Create Series by assigning names
products = pd.Series(
["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
name="Products",
)
dollar_price = pd.Series([350, 300, 400, 250], name="Price")
# using pandas series merge()
df = pd.merge(products, dollar_price, right_index=True, left_index=True)
print(df)
输出:
Products Price
0 Intel Dell Laptops 350
1 HP Laptops 300
2 Lenavo Laptops 400
3 Acer Laptops 250
使用 Series.append()
方法将两个 Pandas 系列合并到一个 DataFrame
中
Series.append()
方法是 concat()
方法的快捷方式。此方法沿 axis=0
或行附加系列。使用这种方法,我们可以通过将系列作为行而不是列附加到另一个系列来创建 DataFrame
。
我们在源代码中以如下方式使用了 series.append()
方法:
示例代码:
import pandas as pd
# Using Series.append()
technical = pd.Series(["Pandas", "Python", "Scala", "Hadoop"])
non_technical = pd.Series(["SEO", "Graphic design", "Content writing", "Marketing"])
# using the appen() method merge series and create dataframe
df = pd.DataFrame(
technical.append(non_technical, ignore_index=True), columns=["Skills"]
)
print(df)
输出:
Skills
0 Pandas
1 Python
2 Scala
3 Hadoop
4 SEO
5 Graphic design
6 Content writing
7 Marketing
使用 DataFrame.join()
方法将两个 Pandas 系列合并为一个 DataFrame
使用 DataFrame.join()
方法,我们可以连接两个系列。当我们使用这种方法时,我们必须将一个系列转换为 DataFrame
对象。然后我们将使用结果与另一个系列组合。
在以下示例中,我们已将第一个系列转换为 DataFrame
对象。然后,我们使用这个 DataFrame
与另一个系列合并。
示例代码:
import pandas as pd
# Create Series by assigning names
products = pd.Series(
["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
name="Products",
)
dollar_price = pd.Series([350, 300, 400, 250], name="Price")
# Merge series using DataFrame.join() method
df = pd.DataFrame(products).join(dollar_price)
print(df)
输出:
Products Price
0 Intel Dell Laptops 350
1 HP Laptops 300
2 Lenavo Laptops 400
3 Acer Laptops 250
结论
我们在本教程中学习了如何使用四种不同的方式将两个 Pandas 系列合并为一个 DataFrame
。此外,我们探索了这四种方法 pandas.concat()
、Series.append()
、pandas.merge()
和 DataFrame.join()
如何帮助我们解决 Pandas 合并系列任务。
相关文章 - Pandas DataFrame
- 如何将 Pandas DataFrame 列标题获取为列表
- 如何删除 Pandas DataFrame 列
- 如何在 Pandas 中将 DataFrame 列转换为日期时间
- 如何在 Pandas DataFrame 中将浮点数转换为整数
- 如何按一列的值对 Pandas DataFrame 进行排序
- 如何用 group-by 和 sum 获得 Pandas 总和