如何使用索引为 Pandas DataFrame 中的特定单元格设置值
Puneet Dobhal
2023年1月30日
-
使用
pandas.dataframe.at
方法为 pandas DataFrame 中的特定单元格设置值 -
使用
Dataframe.set_value()
方法为 pandas DataFrame 中的特定单元格设置值 -
使用
Dataframe.loc
方法为 pandas DataFrame 中的特定单元格设置值
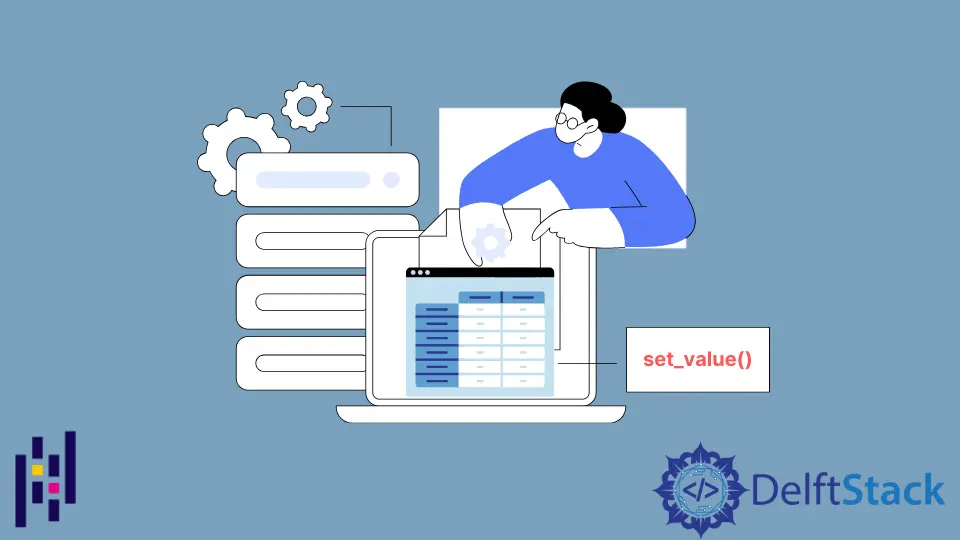
Pandas 是一个以数据为中心的 python 软件包,它使 python 中的数据分析变得容易且一致。在本文中,我们将研究使用索引访问和设置 pandas DataFrame 数据结构中特定单元格值的不同方法。
使用 pandas.dataframe.at
方法为 pandas DataFrame 中的特定单元格设置值
当需要在 DataFrame 中设置单个值时,主要使用 pandas.dataframe.at
方法。
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.at[0, "Col1"] = 99
sample_df.at[1, "Col2"] = 99
sample_df.at[2, "Col3"] = 99
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
输出:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 99 20 30
1 11 99 31
2 15 25 99
你可能会注意到,在访问单元格时,我们已将索引和列指定为 .at[0, 'Col1']
,其中第一个参数是索引,第二个参数是列。
如果你省略列而仅指定索引,则该索引的所有值都将被修改。
使用 Dataframe.set_value()
方法为 pandas DataFrame 中的特定单元格设置值
另一种选择是 Dataframe.set_value()
方法。这与以前的方法非常相似,一次访问一个值,但是语法略有不同。
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.set_value(0, "Col1", 99)
sample_df.set_value(1, "Col2", 99)
sample_df.set_value(2, "Col3", 99)
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
输出:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 99 20 30
1 11 99 31
2 15 25 99
使用 Dataframe.loc
方法为 pandas DataFrame 中的特定单元格设置值
设置特定单元格的语法略有不同的另一种可行方法是 dataframe.loc
方法。
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.loc[0, "Col3"] = 99
sample_df.loc[1, "Col2"] = 99
sample_df.loc[2, "Col1"] = 99
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
输出:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 10 20 99
1 11 99 31
2 99 25 35
本文中所有上述方法都是在 Pandas DataFrame
中修改或设置特定单元格的便捷方法,语法和规范上有微小差异。