获取 Dataframe Pandas 的第一行
Suraj Joshi
2023年1月30日
2021年1月22日
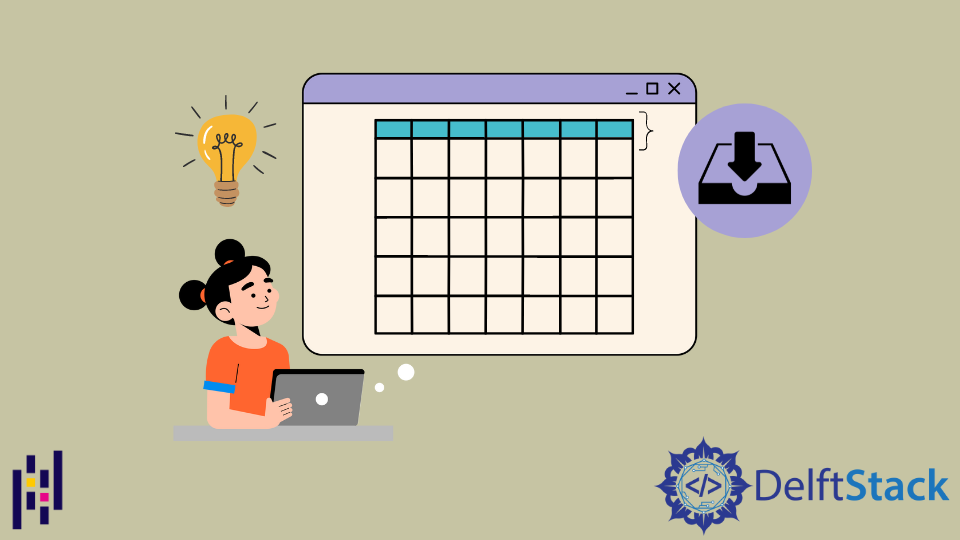
本教程介绍了如何使用 pandas.DataFrame.iloc
属性和 pandas.DataFrame.head()
方法从 Pandas DataFrame 中获取第一行。
我们将在下面的例子中使用以下 DataFrame 来解释如何从 Pandas DataFrame 中获取第一行。
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 200, 350],
})
print(df)
输出:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
使用 pandas.DataFrame.iloc
属性获取 Pandas DataFrame 的第一行
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 200, 350],
})
row_1=df.iloc[0]
print("The DataFrame is:")
print(df,"\n")
print("The First Row of the DataFrame is:")
print(row_1)
输出:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame is:
C_1 A
C_2 40
C_3 430
Name: 0, dtype: object
它显示 DataFrame df
的第一行。为了选择第一行,我们使用第一行的默认索引,即 0
和 DataFrame 的 iloc
属性。
使用 pandas.DataFrame.head()
方法从 Pandas DataFrame 中获取第一行
pandas.DataFrame.head()
方法返回一个 DataFrame,其中包含 DataFrame 中最上面的 5 行。我们也可以传递一个数字作为参数给 pandas.DataFrame.head()
方法,代表要选择的最上面的行数。我们可以传递 1 作为参数到 pandas.DataFrame.head()
方法中,只选择 DataFrame 的第一行。
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 200, 350],
})
row_1=df.head(1)
print("The DataFrame is:")
print(df,"\n")
print("The First Row of the DataFrame is:")
print(row_1)
输出:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame is:
C_1 C_2 C_3
0 A 40 430
根据指定的条件从 Pandas DataFrame 中获取第一行
为了从 DataFrame 中提取满足指定条件的第一行,我们首先过滤满足指定条件的行,然后使用上面讨论的方法从过滤后的 DataFrame 中选择第一行。
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 500, 350],
})
filtered_df=df[(df.C_2 < 40) & (df.C_3 > 450)]
row_1_filtered=filtered_df.head(1)
print("The DataFrame is:")
print(df,"\n")
print("The Filtered DataFrame is:")
print(filtered_df,"\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
输出:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
它将显示第一条列 C_2
值小于 45 且 C_3
列值大于 450 的行。
我们也可以使用 query()
方法来过滤 DataFrame 中的行。
import pandas as pd
df = pd.DataFrame({
'C_1': ["A","B","C","D"],
'C_2': [40,34,38,45],
'C_3': [430, 980, 500, 350],
})
filtered_df=df.query('(C_2 < 40) & (C_3 > 450)')
row_1_filtered=filtered_df.head(1)
print("The DataFrame is:")
print(df,"\n")
print("The Filtered DataFrame is:")
print(filtered_df,"\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
输出:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
它将使用 query()
方法过滤所有列 C_2
值小于 45 和列 C_3
值大于 450 的行,然后使用 head()
方法从 filtered_df
中选择第一行。
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn