Pandas DataFrame 删除某行
Suraj Joshi
2023年1月30日
2021年1月22日
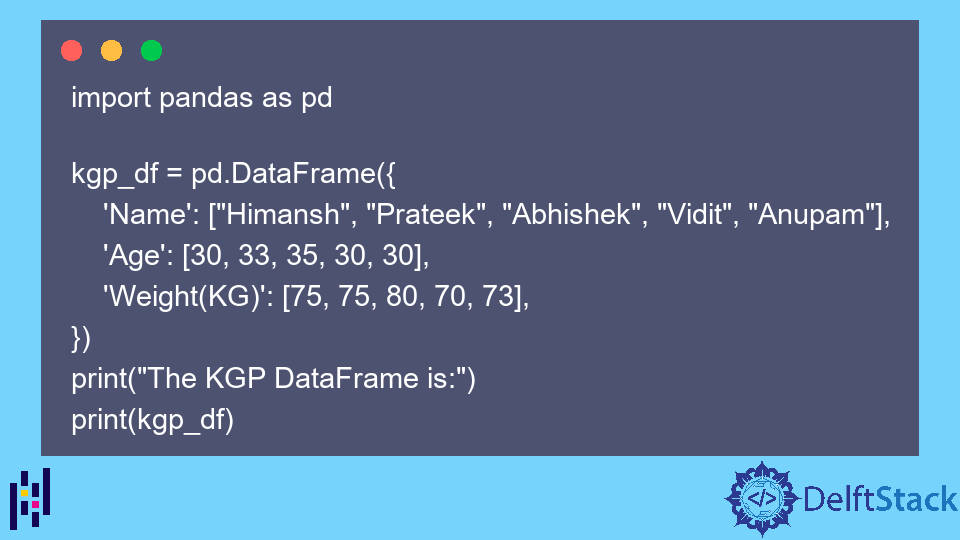
本教程说明了如何使用 pandas.DataFrame.drop()
方法在 Pandas 中删除行。
import pandas as pd
kgp_df = pd.DataFrame({
'Name': ["Himansh", "Prateek", "Abhishek", "Vidit", "Anupam"],
'Age': [30, 33, 35, 30, 30],
'Weight(KG)': [75, 75, 80, 70, 73],
})
print("The KGP DataFrame is:")
print(kgp_df)
输出:
The KGP DataFrame is:
Name Age Weight(KG)
0 Himansh 30 75
1 Prateek 33 75
2 Abhishek 35 80
3 Vidit 30 70
4 Anupam 30 73
我们将使用 kgp_df
DataFrame 来解释如何从 Pandas DataFrame 中删除行。
在 pandas.DataFrame.drop()
方法中按索引删除行
import pandas as pd
kgp_df = pd.DataFrame({
'Name': ["Himansh", "Prateek", "Abhishek", "Vidit", "Anupam"],
'Age': [30, 33, 35, 30, 30],
'Weight(KG)': [75, 75, 80, 70, 73],
})
rows_dropped_df=kgp_df.drop(kgp_df.index[[0,2]])
print("The KGP DataFrame is:")
print(kgp_df,"\n")
print("The KGP DataFrame after dropping 1st and 3rd DataFrame is:")
print(rows_dropped_df)
输出:
The KGP DataFrame is:
Name Age Weight(KG)
0 Himansh 30 75
1 Prateek 33 75
2 Abhishek 35 80
3 Vidit 30 70
4 Anupam 30 73
The KGP DataFrame after dropping 1st and 3rd DataFrame is:
Name Age Weight(KG)
1 Prateek 33 75
3 Vidit 30 70
4 Anupam 30 73
从 kgp_df
DataFrame 中删除索引为 0 和 2 的行。索引 0 和 2 的行对应 DataFrame 中的第一行和第三行,因为索引是从 0 开始的。
我们也可以使用 DataFrame 的索引来删除这些行,而不是使用默认的索引。
import pandas as pd
kgp_idx=["A","B","C","D","E"]
kgp_df = pd.DataFrame({
'Name': ["Himansh", "Prateek", "Abhishek", "Vidit", "Anupam"],
'Age': [30, 33, 35, 30, 30],
'Weight(KG)': [75, 75, 80, 70, 73],
},index=kgp_idx)
rows_dropped_df=kgp_df.drop(["A","C"])
print("The KGP DataFrame is:")
print(kgp_df,"\n")
print("The KGP DataFrame after dropping 1st and 3rd DataFrame is:")
print(rows_dropped_df)
输出:
The KGP DataFrame is:
Name Age Weight(KG)
A Himansh 30 75
B Prateek 33 75
C Abhishek 35 80
D Vidit 30 70
E Anupam 30 73
The KGP DataFrame after dropping 1st and 3rd DataFrame is:
Name Age Weight(KG)
B Prateek 33 75
D Vidit 30 70
E Anupam 30 73
它从 DataFrame 中删除索引 A
和 C
的行,或者第一行和第三行。
我们将要删除的行的索引列表传递给 drop()
方法来删除相应的行。
根据 Pandas DataFrame 中某一列的值来删除行
import pandas as pd
kgp_idx=["A","B","C","D","E"]
kgp_df = pd.DataFrame({
'Name': ["Himansh", "Prateek", "Abhishek", "Vidit", "Anupam"],
'Age': [31, 33, 35, 36, 34],
'Weight(KG)': [75, 75, 80, 70, 73],
},index=kgp_idx)
young_df_idx=kgp_df[kgp_df["Age"]<=33].index
young_folks=kgp_df.drop(young_df_idx)
print("The KGP DataFrame is:")
print(kgp_df,"\n")
print("The DataFrame of folks with age less than or equal to 33 are:")
print(young_folks)
输出:
The KGP DataFrame is:
Name Age Weight(KG)
A Himansh 31 75
B Prateek 33 75
C Abhishek 35 80
D Vidit 36 70
E Anupam 34 73
The DataFrame of folks with age less than or equal to 33 are:
Name Age Weight(KG)
C Abhishek 35 80
D Vidit 36 70
E Anupam 34 73
它将删除所有年龄小于或等于 33 岁的行。
我们首先找到所有年龄小于或等于 33 岁的行的索引,然后使用 drop()
方法删除这些行。
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn