使用 PHP 和 MySQL 设置搜索系统
Habdul Hazeez
2023年1月30日
2022年5月13日
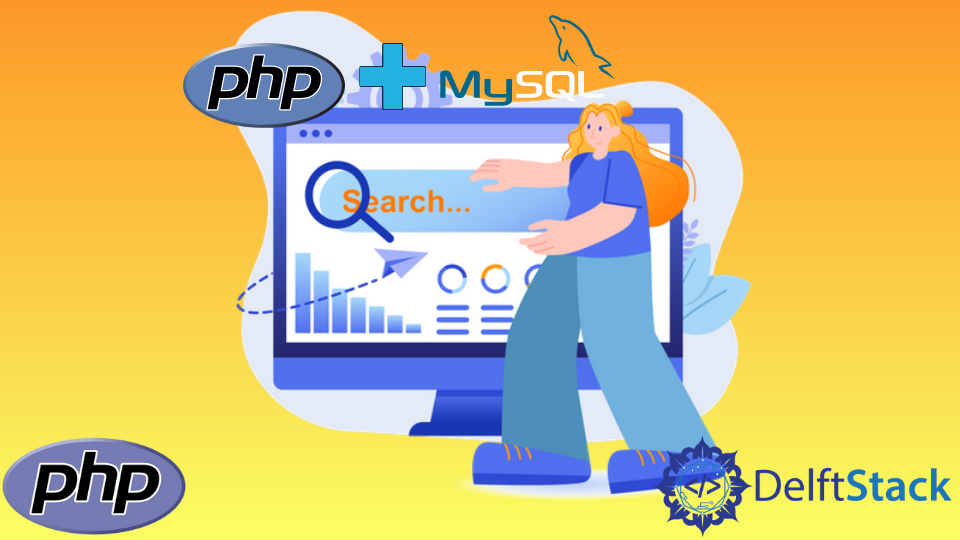
本教程将教你使用 PHP 和 MySQL 创建一个搜索系统。你将学习如何设置 HTML、MySQL 数据库和 PHP 后端。在 PHP 代码中,你将学习在 SQL 中使用带有 LIKE 运算符的准备好的语句。
设置数据库
下载并安装 XAMPP 服务器。它与 MySQL 一起提供。在 XAMPP 控制面板中启动 shell。使用以下命令登录 MySQL shell:
# This login command assumes that the
# password is empty and the user is "root"
mysql -u root -p
使用以下 SQL 查询创建一个名为 fruit_db
的数据库。
CREATE database fruit_db;
输出:
Query OK, 1 row affected (0.001 sec)
你将需要可以使用的示例数据。因此,在 fruit_db
数据库上执行以下 SQL:
CREATE TABLE fruit
(id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(20) NOT NULL,
color VARCHAR(20) NOT NULL,
PRIMARY KEY (id))
ENGINE = InnoDB;
输出:
Query OK, 0 rows affected (0.028 sec)
检查表的结构:
DESC fruit;
输出:
+-------+-------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+----------------+
| id | int(11) | NO | PRI | NULL | auto_increment |
| name | varchar(20) | NO | | NULL | |
| color | varchar(20) | NO | | NULL | |
+-------+-------------+------+-----+---------+----------------+
建立表后,使用以下 SQL 插入示例数据:
INSERT INTO fruit (id, name, color) VALUES (NULL, 'Banana', 'Yellow'), (NULL, 'Pineapple', 'Green')
输出:
Query OK, 2 rows affected (0.330 sec)
Records: 2 Duplicates: 0 Warnings: 0
使用以下 SQL 确认数据存在:
SELECT * FROM fruit;
输出:
+----+-----------+--------+
| id | name | color |
+----+-----------+--------+
| 1 | Banana | Yellow |
| 2 | Pineapple | Green |
+----+-----------+--------+
创建 HTML 代码
搜索系统的 HTML 代码是一个 HTML 表单。该表单有一个表单输入和一个 submit
按钮。表单输入中的 required
属性确保用户在表单中输入内容。
下一个代码块是搜索表单的 HTML 代码。
<main>
<form action="searchdb.php" method="post">
<input
type="text"
placeholder="Enter your search term"
name="search"
required>
<button type="submit" name="submit">Search</button>
</form>
</main>
下面的 CSS 使表单更美观。
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
display: grid;
align-items: center;
place-items: center;
height: 100vh;
}
main {
width: 60%;
border: 2px solid #1560bd;
padding: 2em;
display: flex;
justify-content: center;
}
input,
button {
padding: 0.2em;
}
HTML 应该类似于你的网络浏览器中的下一个图像。
创建 PHP 代码
PHP 代码将处理表单提交。以下是代码工作原理的摘要:
- 检查用户提交的表单。
- 连接数据库。
- 转义搜索字符串并去除所有的空白。
- 检查无效字符,如
<
或-
(不带引号)。 - 通过准备好的语句执行搜索。
- 返回结果。
下一个代码块是执行搜索的完整 PHP 代码。将代码保存在名为 searchdb.php
的文件中。
<?php
if (isset($_POST['submit'])) {
// Connect to the database
$connection_string = new mysqli("localhost", "root", "", "fruit_db");
// Escape the search string and trim
// all whitespace
$searchString = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['search'])));
// If there is a connection error, notify
// the user, and Kill the script.
if ($connection_string->connect_error) {
echo "Failed to connect to Database";
exit();
}
// Check for empty strings and non-alphanumeric
// characters.
// Also, check if the string length is less than
// three. If any of the checks returns "true",
// return "Invalid search string", and
// kill the script.
if ($searchString === "" || !ctype_alnum($searchString) || $searchString < 3) {
echo "Invalid search string";
exit();
}
// We are using a prepared statement with the
// search functionality to prevent SQL injection.
// So, we need to prepend and append the search
// string with percent signs
$searchString = "%$searchString%";
// The prepared statement
$sql = "SELECT * FROM fruit WHERE name LIKE ?";
// Prepare, bind, and execute the query
$prepared_stmt = $connection_string->prepare($sql);
$prepared_stmt->bind_param('s', $searchString);
$prepared_stmt->execute();
// Fetch the result
$result = $prepared_stmt->get_result();
if ($result->num_rows === 0) {
// No match found
echo "No match found";
// Kill the script
exit();
} else {
// Process the result(s)
while ($row = $result->fetch_assoc()) {
echo "<b>Fruit Name</b>: ". $row['name'] . "<br />";
echo "<b>Fruit Color</b>: ". $row['color'] . "<br />";
} // end of while loop
} // end of if($result->num_rows)
} else { // The user accessed the script directly
// Tell them nicely and kill the script.
echo "That is not allowed!";
exit();
}
?>
下一张图片包含搜索字符串 pine
的结果。它返回水果 pine
及其颜色。
Author: Habdul Hazeez
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn