如何在 PHP 中解析 JSON 文件
Minahil Noor
2021年4月29日
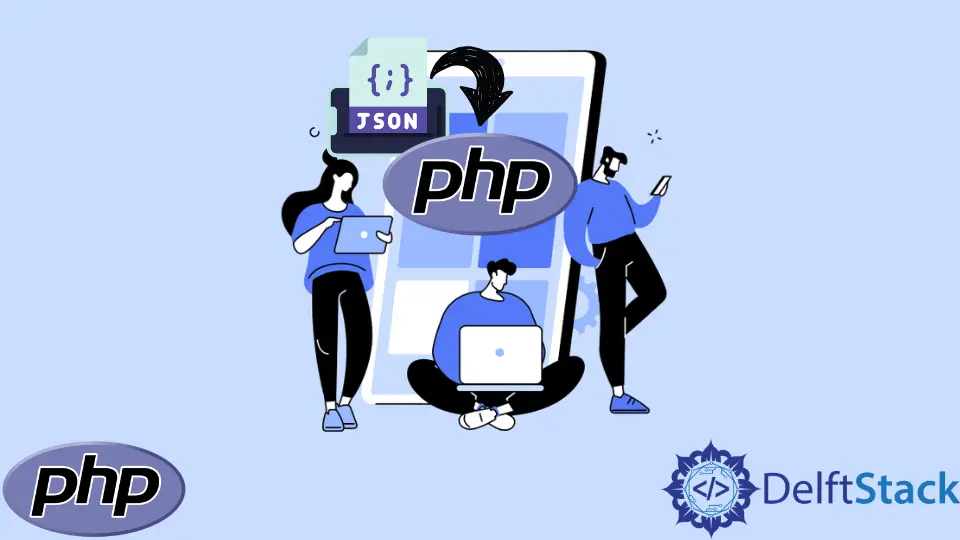
在本文中,我们将介绍在 PHP 中解析 JSON
文件的方法。
- 使用
file_get_contents()
函数
示例代码中使用的 JSON
文件的内容如下。
[
{
"id": "01",
"name": "Olivia Mason",
"designation": "System Architect"
},
{
"id": "02",
"name": "Jennifer Laurence",
"designation": "Senior Programmer"
},
{
"id": "03",
"name": "Medona Oliver",
"designation": "Office Manager"
}
]
使用 file_get_contents()
函数解析 PHP 中的 JSON 文件
内置函数 file_get_contents()
用于读取文件并将其存储为字符串。通过使用此函数,我们可以将 JSON
文件解析为字符串。使用此函数的正确语法如下。
file_get_contents($pathOfFile, $searchPath, $customContext, $startingPoint, $length);
此函数接受五个参数。这些参数的详细信息如下。
参数 | 描述 | |
---|---|---|
$pathOfFile |
强制性的 | 它指定文件的路径 |
$searchPath |
可选的 | 它指定搜索文件的路径 |
$customContext |
可选的 | 它用于指定自定义上下文 |
$startingPoint |
可选的 | 它指定读取文件的起点 |
$length |
可选的 | 它是要读取的文件的最大长度(以字节为单位) |
以下程序显示了如何解析 JSON
文件。
<?php
$JsonParser = file_get_contents("myfile.json");
var_dump($JsonParser);
?>
函数 file_get_contents()
仅解析存储在 JSON 文件中的 JSON 数据。我们无法直接使用此数据。
输出:
string(328) "[
{
"id": "01",
"name": "Olivia Mason",
"designation": "System Architect"
},
{
"id": "02",
"name": "Jennifer Laurence",
"designation": "Senior Programmer"
},
{
"id": "03",
"name": "Medona Oliver",
"designation": "Office Manager"
}
]"
为了使这些数据有用,我们可以使用 json_decode()
将 JSON 字符串转换为数组。在以下程序中使用此函数。
<?php
$Json = file_get_contents("myfile.json");
// Converts to an array
$myarray = json_decode($Json, true);
var_dump($myarray); // prints array
?>
输出:
array(3) {
[0]=>
array(3) {
["id"]=>
string(2) "01"
["name"]=>
string(12) "Olivia Mason"
["designation"]=>
string(16) "System Architect"
}
[1]=>
array(3) {
["id"]=>
string(2) "02"
["name"]=>
string(17) "Jennifer Laurence"
["designation"]=>
string(17) "Senior Programmer"
}
[2]=>
array(3) {
["id"]=>
string(2) "03"
["name"]=>
string(13) "Medona Oliver"
["designation"]=>
string(14) "Office Manager"
}
}