在 NumPy 中获取两个数组的组合
-
使用 Python 中的
itertools.product()
函数获取 NumPy 数组组合 -
使用 Python 中的
numpy.meshgrid()
函数获取 NumPy 数组组合 -
使用 Python 中的
for-in
方法获取 NumPy 数组组合
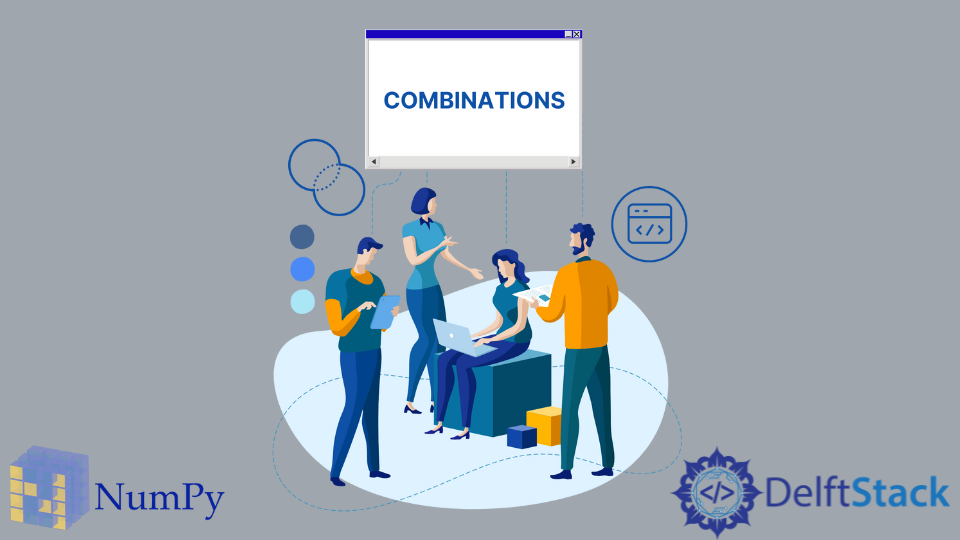
本文将介绍如何在 Python 中求两个 NumPy 数组的笛卡尔积。
使用 Python 中的 itertools.product()
函数获取 NumPy 数组组合
itertools
包提供了许多与组合和排列相关的功能。我们可以使用两个可迭代对象的 itertools.product()
函数 笛卡尔积。itertools.product()
函数将可迭代对象作为输入参数,并返回可迭代对象的笛卡尔积。
import itertools as it
import numpy as np
array = np.array([1, 2, 3])
combinations = it.product(array,array)
for combination in combinations:
print(combination)
输出:
(1, 1)
(1, 2)
(1, 3)
(2, 1)
(2, 2)
(2, 3)
(3, 1)
(3, 2)
(3, 3)
在上面的代码中,我们通过使用 itertools
包内的 product()
函数计算了 array
与其自身的笛卡尔叉积,并将结果存储在 combinations
中。
使用 Python 中的 numpy.meshgrid()
函数获取 NumPy 数组组合
我们还可以使用 NumPy 包中的 meshgrid()
函数 来计算两个 NumPy 数组的笛卡尔积。numpy.meshgrid()
函数将数组作为输入参数并返回两个数组的叉积。
import numpy as np
array = np.array([1,2,3])
combinations = np.array(np.meshgrid(array, array)).T.reshape(-1,2)
print(combinations)
输出:
[[1 1]
[1 2]
[1 3]
[2 1]
[2 2]
[2 3]
[3 1]
[3 2]
[3 3]]
在上面的代码中,我们使用 NumPy 中的 meshgrid()
函数计算了 array
与其自身的笛卡尔叉积。然后,我们使用 np.array()
函数将此操作的结果转换为一个数组,并使用 numpy.reshape()
函数对其进行整形。然后我们将新的重塑结果存储在 combinations
数组中。
使用 Python 中的 for-in
方法获取 NumPy 数组组合
实现与前两个示例相同的目标的另一种更直接的方法是使用 for-in
迭代器。for-in
迭代器用于迭代 Python 中可迭代对象内的每个元素。无需导入任何新包或库即可使用此方法。
import numpy as np
array = np.array([1,2,3])
array2 = np.array([1, 2, 3])
combinations = np.array([(i,j) for i in array for j in array2])
print(combinations)
输出:
[[1 1]
[1 2]
[1 3]
[2 1]
[2 2]
[2 3]
[3 1]
[3 2]
[3 3]]
我们在上面的代码中使用嵌套的 for-in
迭代器计算了两个数组的笛卡尔叉积。我们使用 np.array()
函数将结果保存在 NumPy 数组 combinations
中。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn