在 JavaScript 中等待 X 秒
Moataz Farid
2023年10月12日
-
在 JavaScript 中使用
setTimeout()
来等待 X 秒 -
在 JavaScript 中使用
promises
和async/await
等待 X 秒 -
使用
for
循环来实现 JavaScript 中的同步delay
函数
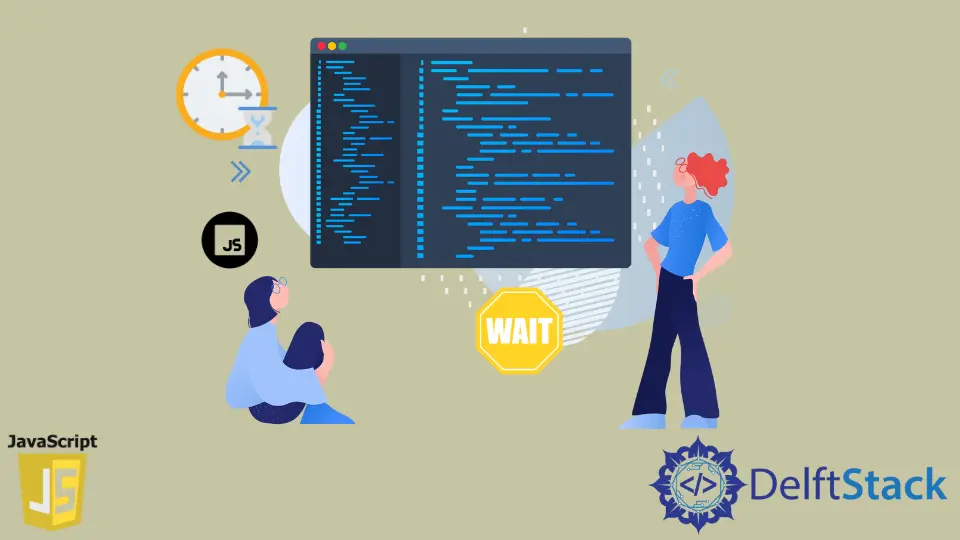
本教程将讲解如何在 JavaScript 中等待 x 秒后再继续执行。我们将实现一个 delay(seconds)
函数,它将阻塞线程 x 秒。我们还将解释这个延迟的多种实现技术。
当我们谈到实现延迟函数时,使用最广泛的方法是异步的 setTimeout()
。
在 JavaScript 中使用 setTimeout()
来等待 X 秒
异步 setTimeout()
方法是高阶函数之一,它以回调函数为参数,在输入时间结束后执行该函数。参数中给出的时间是以 ms
为单位的。
console.log('I am the first log');
setTimeout(function() {
console.log('I am the third log after 5 seconds');
}, 5000);
console.log('I am the second log');
输出:
I am the first log
I am the second log
I am the third log after 5 seconds
在 JavaScript 中使用 promises
和 async/await
等待 X 秒
在异步上下文中实现延迟函数的一种方法是结合 async/await
概念和 promises
概念。我们可以创建一个延迟函数,在里面返回一个新的 promise
,我们将调用 setTimeout()
方法与我们所需的等待时间。之后,我们可以在任何异步上下文里面随意调用那个 delay
函数。
function delay(n) {
return new Promise(function(resolve) {
setTimeout(resolve, n * 1000);
});
}
async function myAsyncFunction() {
// Do what you want here
console.log('Before the delay')
await delay(5);
console.log('After the delay')
// Do what you want here too
}
myAsyncFunction();
输出:
Before the delay
After the delay
延迟函数也可以使用 ECMAScript 6 中的箭头语法进行优化,如下例。
const delay = (n) => new Promise(r => setTimeout(r, n * 1000));
使用 for
循环来实现 JavaScript 中的同步 delay
函数
假设我们没有任何异步上下文来使用上面的 delay()
函数,或者不想使用 setTimeout()
函数。在这种情况下,我们可以用普通的 for
循环和 Date()
类实现一个同步延迟函数来计算时间。
这个函数的实现非常简单。就是让 CPU 在所需时间内保持忙碌。这是通过计算经过的时间,并将其与等待时间进行比较,这样我们就可以在它之后继续执行。
function syncDelay(milliseconds) {
var start = new Date().getTime();
var end = 0;
while ((end - start) < milliseconds) {
end = new Date().getTime();
}
}
console.log('Before the delay')
syncDelay(5000);
console.log('After the delay')
输出:
Before the delay
After the delay