JavaScript 字符串 startsWith
-
在 JavaScript 中使用字符串
startsWith()
方法 -
在 JavaScript 中使用
indexOf()
函数来检查字符串是否以另一个字符串开头 -
在 JavaScript 中使用
lastIndexOf()
函数检查字符串是否以另一个字符串开头 -
在 JavaScript 中使用
substring()
函数检查字符串是否以另一个字符串开头 -
使用 Regex 的
test()
方法检查字符串是否以 JavaScript 中的另一个字符串开头 - 在 JavaScript 中使用带有循环的自定义函数来检查字符串是否以另一个字符串开头
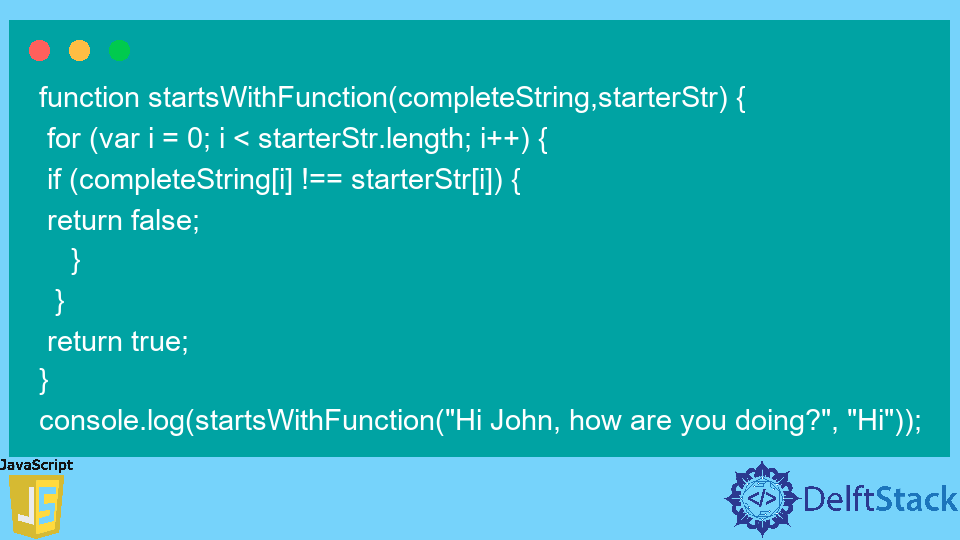
当我们想要确保字符串是否以特定字符串开头时,在编码时会有一些情况。
例如,我想检查给定的字符串是否以 Me
字符串开头。
今天,我们将学习如何使用 startsWith()
、indexOf()
、lastIndexOf()
、substring()
函数以及正则表达式的 test()
方法来检查字符串是否以特定字符串开头和一个带循环的自定义函数。
在 JavaScript 中使用字符串 startsWith()
方法
ECMAScript 2015(也称为 ES6)引入了 startsWith()
方法,该方法检查字符串是否以指定字符串开头。如果找到匹配,则输出 true
;否则,假
。
startsWith()
方法有两个参数:searchString
,第二个是 position
,它是可选的,默认为零。如果指定了位置,则函数从该位置搜索字符串。
在下面的例子中,我们没有指定 position
。因此,它从索引零开始区分大小写搜索。
var name = "Hi John, how are you doing?"
console.log(name.startsWith("Hi John"));
输出:
true
示例代码:
var name = "Hi John, how are you doing?"
console.log(name.startsWith("Hi john"));
输出:
false
示例代码:
var name = "Hi John, how are you doing?"
console.log(name.startsWith("how", 9)); //it starts searching and matching from index 9
输出:
true
在 JavaScript 中使用 indexOf()
函数来检查字符串是否以另一个字符串开头
indexOf()
与数组一起使用来定位元素并返回找到它的第一个索引,但我们也可以通过以下方式使用字符串。
如果匹配元素的索引为零,则表示字符串以指定字符串开头。
示例代码:
var name = "Hi John, how are you doing?"
if(name.indexOf("Hi") === 0)
console.log(true);
else
console.log(false);
输出:
true
它还区分大小写搜索。看看下面的示例代码。
var name = "Hi John, how are you doing?"
if(name.indexOf("hi") === 0)
console.log(true);
else
console.log(false);
输出:
false
如果我们想让 indexOf()
从指定位置定位元素怎么办?
var name = "Hi John, how are you doing?"
if(name.indexOf("how") === 9)
console.log(true);
else
console.log(false);
输出:
true
在 JavaScript 中使用 lastIndexOf()
函数检查字符串是否以另一个字符串开头
此函数返回字符串中特定值的最后一次出现的位置/索引。
它从末尾开始搜索并移向字符串的开头。如果值不存在,则返回 -1
。
我们可以稍微修改一下,通过下面的方式使用 lastIndexOf()
方法来查看字符串是否以另一个字符串开头。
示例代码:
var name = "Hi John, how are you doing?"
if(name.lastIndexOf("Hi", 0) === 0)
console.log(true);
else
console.log(false);
输出:
true
虽然,它从头开始搜索。但是我们在索引 0 处指定它的结尾。
请记住,对于此功能,hi
和 Hi
是不同的。
你可以这里详细了解它。
在 JavaScript 中使用 substring()
函数检查字符串是否以另一个字符串开头
除了指定另一个字符串来检查字符串是否以它开头,我们还可以根据字符串的长度输入索引范围。
例如,我们要检查字符串是否以 Hi
开头,因此索引如下。
var name = "Hi John, how are you doing?"
//here the end index is not included means the end index
//would be endIndex-1
console.log(name.substring(0,2));
输出:
Hi
如果我们寻找 Hi John
,我们可以修改它,如下所示。
var name = "Hi John, how are you doing?"
console.log(name.substring(0,7));
输出:
Hi John
如果你要在中间某处寻找特定的字符串部分,则使用此函数不是一个好的选择。原因是你可能在给定索引上找不到预期的字符串。
使用 Regex 的 test()
方法检查字符串是否以 JavaScript 中的另一个字符串开头
var name = "Hi John, how are you doing?"
console.log(/^Hi John/.test(name));
输出:
true
它也区分大小写。请参阅以下示例。
var name = "Hi John, how are you doing?"
console.log(/^Hi john/.test(name));
输出:
false
你还可以使用具有不同参数和属性的 RegExp
对象的方法。你可以在此处详细了解它。
你是否考虑过使用自定义函数来完成此功能?让我们看一下以下部分。
在 JavaScript 中使用带有循环的自定义函数来检查字符串是否以另一个字符串开头
示例代码:
function startsWithFunction(completeString,starterStr) {
for (var i = 0; i < starterStr.length; i++) {
if (completeString[i] !== starterStr[i]) {
return false;
}
}
return true;
}
console.log(startsWithFunction("Hi John, how are you doing?", "Hi"));
输出:
true
startsWithFunction()
采用主字符串 Hi John, how are you doing?
和另一个字符串 Hi
,以检查主字符串是否以它开头。
此函数匹配每个字符并在成功时返回 true
;否则返回 false
。