在 JavaScript 中从字符串中删除字符
Kirill Ibrahim
2023年1月30日
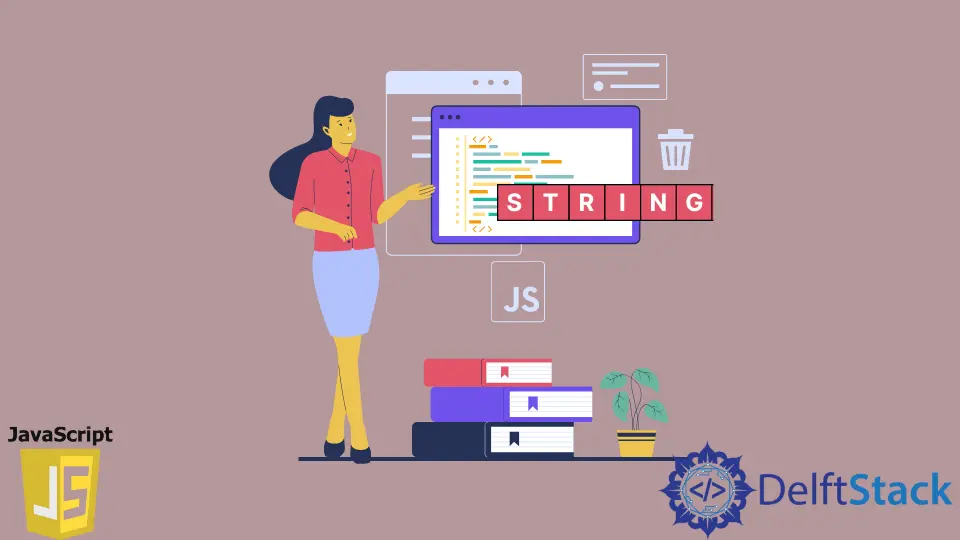
JavaScript 有不同的方法来删除字符串中的特定字符。我们将介绍如何在 JavaScript 中删除字符串中的字符。
在 JavaScript 中使用正则表达式的 replace()
方法
在 JavaScript 中,我们使用正则表达式的 replace()
方法来删除字符串中指定字符的所有实例。
JavaScript replace()
正则表达式语法
replace(/regExp/g, '');
例子:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove all instances of the specified character in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove all instances of the specified character in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString()">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = () => {
originalWord = 'DelftStack';
newWord = originalWord.replace(/t/g, '');
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>
输出:
The original string is DelftStack
New Output is: DelfSack
在 JavaScript 中删除指定索引处的指定字符
当我们需要删除一个字符时,当一个字符串中有多个该字符的实例时,例如,从字符串 DelftStack
中删除字符 t
,我们可以使用 slice()
方法得到给定索引前后的两个字符串,并将其连接起来。
例子:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove Specified Character at a Given Index in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove Specified Character at a Given Index in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString(5)">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = (position) => {
originalWord = 'DelftStack';
newWord = originalWord.slice(0, position - 1)
+ originalWord.slice(position, originalWord.length);
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>
在 JavaScript 中删除字符串中字符的第一个实例
我们可以使用 replace()
方法,但不使用正则表达式,在 JavaScript 中只删除字符串中的第一个字符实例。我们把要删除的字符作为第一个参数,把空字符串''
作为第二个参数。
例子:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove First Instance of Character in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove First Instance of Character in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString()">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = () => {
originalWord = 'DelftStack';
newWord = originalWord.replace('t', '');
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>