Java 中 size 和 length 的区别
-
Java 中数组的
length
属性 -
Java 数组中
.length
的示例 -
Java 数组中
size()
方法的示例 -
使用 Java 中的
length()
方法查找长度 -
Java Collections
size()
方法 - Java 中大小和长度的区别
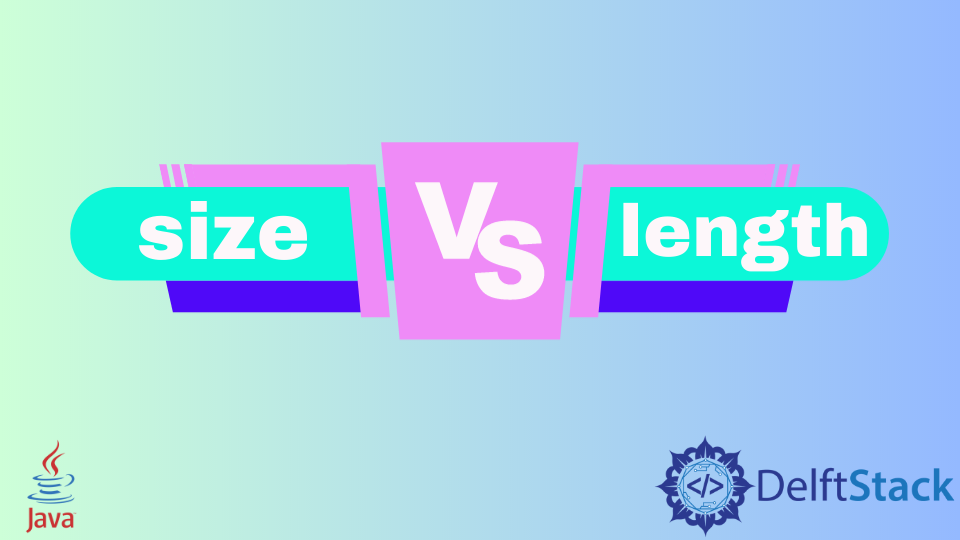
本教程介绍了 Java 中大小和长度之间的区别。我们还列出了一些示例代码以帮助你理解该主题。
Java 有一个 size()
方法和一个 length
属性。初学者可能认为它们是可以互换的并执行相同的任务,因为它们听起来有些相同。在 Java 中,大小和长度是两个不同的东西。在这里,我们将了解两者之间的差异。
Java 中数组的 length
属性
数组以有序的方式存储固定数量的相同类型的数据。Java 中的所有数组都有一个长度字段,用于存储为该数组的元素分配的空间。它是一个常数值,用于找出数组的最大容量。
- 请记住,该字段不会为我们提供数组中存在的元素数,而是可以存储的最大元素数(无论元素是否存在)。
Java 数组中 .length
的示例
在下面的代码中,我们首先初始化一个长度为 7
的数组。即使我们没有添加任何元素,该数组的长度字段仍显示 7
。这个 7
只是表示最大容量。
public class Main
{
public static void main(String[] args)
{
int[] intArr = new int[7];
System.out.print("Length of the Array is: " + intArr.length);
}
}
输出:
Length of the Array is: 7
现在,让我们使用它们的索引将 3 个元素添加到数组中,然后打印长度字段。还是显示 7。
public class Main
{
public static void main(String[] args)
{
int[] intArr = new int[7];
intArr[0] = 20;
intArr[1] = 25;
intArr[2] = 30;
System.out.print("Length of the Array is: " + intArr.length);
}
}
输出:
Length of the Array is: 7
长度字段是常量,因为数组的大小是固定的。我们必须定义在初始化期间我们将存储在数组中的最大元素数(数组的容量),我们不能超过这个限制。
Java 数组中 size()
方法的示例
数组没有 size()
方法;它会返回一个编译错误。请参考下面的示例。
public class SimpleTesting
{
public static void main(String[] args)
{
int[] intArr = new int[7];
System.out.print("Length of the Array is: " + intArr.size());
}
}
输出:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Cannot invoke size() on the array type int[]
at SimpleTesting.main(SimpleTesting.java:7)
使用 Java 中的 length()
方法查找长度
Java 字符串只是字符的有序集合,与数组不同,它们具有 length()
方法而不是 length
字段。此方法返回字符串中存在的字符数。
请参考下面的示例。
public class Main
{
public static void main(String[] args)
{
String str1 = "This is a string";
String str2 = "Another String";
System.out.println("The String is: " + str1);
System.out.println("The length of the string is: " + str1.length());
System.out.println("\nThe String is: " + str2);
System.out.println("The length of the string is: " + str2.length());
}
}
输出:
The String is: This is a string
The length of the string is: 16
The String is: Another String
The length of the string is: 14
请注意,我们不能在字符串上使用 length
属性,并且 length()
方法不适用于数组。下面的代码显示了我们滥用它们时的错误。
public class SimpleTesting
{
public static void main(String[] args)
{
String str1 = "This is a string";
System.out.println("The length of the string is: " + str1.length);
}
}
输出:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
length cannot be resolved or is not a field
at SimpleTesting.main(SimpleTesting.java:7)
同样,我们不能在数组上使用字符串 length()
方法。
public class SimpleTesting
{
public static void main(String[] args)
{
int[] intArray = {1, 2, 3};
System.out.println("The length of the string is: " + intArray.length());
}
}
输出:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Cannot invoke length() on the array type int[]
at SimpleTesting.main(SimpleTesting.java:7)
Java Collections size()
方法
size()
是 java.util.Collections
类的一个方法。Collections
类被许多不同的集合(或数据结构)使用,例如 ArrayList
、LinkedList
、HashSet
和 HashMap
。
size()
方法返回集合中当前存在的元素数。与数组的 length
属性不同,size()
方法返回的值不是常数,而是根据元素的数量而变化。
Java 中 Collection Framework
的所有集合都是动态分配的,因此元素的数量可能会有所不同。size()
方法用于跟踪元素的数量。
在下面的代码中,很明显,当我们创建一个没有任何元素的新 ArrayList
时,size()
方法返回 0。
import java.util.ArrayList;
import java.util.List;
public class Main
{
public static void main(String[] args)
{
List<Integer> list = new ArrayList<Integer>();
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
}
}
输出:
The ArrayList is: []
The size of the ArrayList is: 0
但是这个值会随着我们添加或删除元素而改变。添加三个元素后,大小增加到 3。接下来,我们删除了两个元素,列表的大小变为 1。
import java.util.ArrayList;
import java.util.List;
public class Main
{
public static void main(String[] args)
{
List<Integer> list = new ArrayList<Integer>();
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
list.add(20);
list.add(40);
list.add(60);
System.out.println("\nAfter adding three new elements:");
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
list.remove(0);
list.remove(1);
System.out.println("\nAfter removing two elements:");
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
}
}
输出:
The ArrayList is: []
The size of the ArrayList is: 0
After adding three new elements:
The ArrayList is: [20, 40, 60]
The size of the ArrayList is: 3
After removing two elements:
The ArrayList is: [40]
The size of the ArrayList is: 1
Java 中大小和长度的区别
尽管 size 和 length 有时可以在同一上下文中使用,但它们在 Java 中具有完全不同的含义。
数组的 length
字段用于表示数组的最大容量。最大容量是指可以存储在其中的最大元素数。此字段不考虑数组中存在的元素数量并保持不变。
字符串的 length()
方法用于表示字符串中出现的字符数。Collections Framework
的 size()
方法用于查看该集合中当前存在的元素数量。Collections
具有动态大小,因此 size()
的返回值可能会有所不同。