在 Java 中转换字符串为输入流
-
在 Java 中使用
ByteArrayInputStream()
把一个字符串转换为InputStream
-
使用
StringReader
和ReaderInputStream
将字符串转换为InputStream
-
使用
org.apache.commons.io.IOUtils
将字符串转换为InputStream
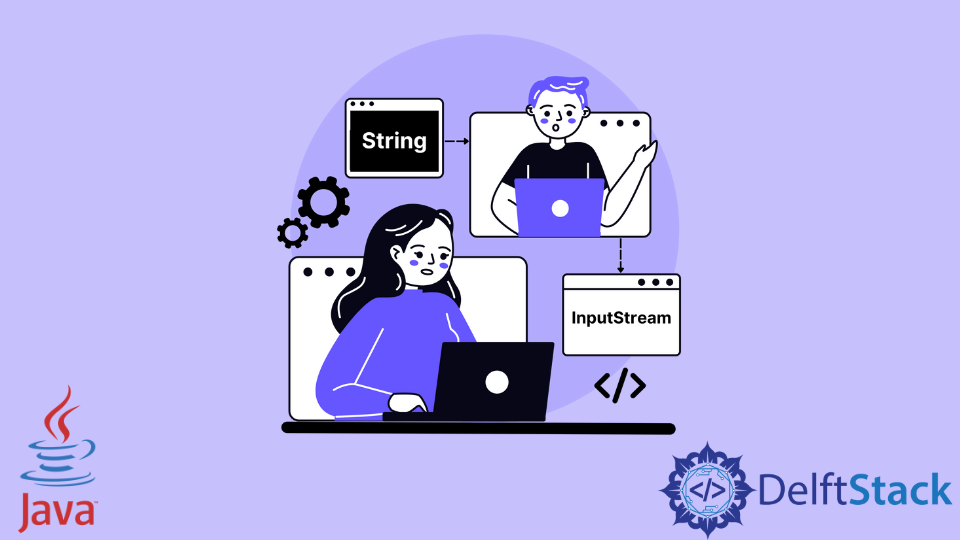
我们将讨论如何在 Java 中使用几种方法将一个字符串转换为一个 InputStream
。字符串是一组字符,而 InputStream 是一组字节。让我们看看如何在 Java 中把字符串转换为 InputStream
。
在 Java 中使用 ByteArrayInputStream()
把一个字符串转换为 InputStream
Java 的 Input/Output 包中有一个类 ByteArrayInputStream
,可以将字节数组读取为 InputStream
。首先,我们使用 getBytes()
从 exampleString
中获取字符集为 UTF_8
的字节,然后传递给 ByteArrayInputStream
。
为了检查我们的目标是否成功,我们可以使用 read()
读取 inputStream
,并将每个字节转换为 char
。这将返回我们的原始字符串。
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream inputStream = new ByteArrayInputStream(exampleString.getBytes(StandardCharsets.UTF_8));
//To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1){
char getSingleChar = (char)i;
System.out.print(getSingleChar);
}
}
}
输出:
This is a sample string
使用 StringReader
和 ReaderInputStream
将字符串转换为 InputStream
将字符串转换为 InputStream
的第二种技术使用两个方法,即 StringReader
和 ReaderInputStream
。前者用于读取字符串并将其包入 reader
中,而后者则需要两个参数,一个 reader
和 charsets。最后,我们得到 InputStream
。
import org.apache.commons.io.input.ReaderInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
StringReader stringReader = new StringReader(exampleString);
InputStream inputStream = new ReaderInputStream(stringReader, StandardCharsets.UTF_8);
//To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1){
char getSingleChar = (char)i;
System.out.print(getSingleChar);
}
}
}
输出:
This is a sample string
使用 org.apache.commons.io.IOUtils
将字符串转换为 InputStream
我们还可以使用 Apache Commons 库来使我们的任务变得更简单。这个 Apache Commons 库的 IOUtls
类有一个 toInputStream()
方法,它接受一个字符串和要使用的 charset
。这个方法是最简单的,因为我们只需要调用一个方法就可以将 Java 字符串转换为 InputStream
。
import org.apache.commons.io.IOUtils;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream is = IOUtils.toInputStream(exampleString, StandardCharsets.UTF_8);
//To check if we can read the string back from the inputstream
int i;
while ((i = is.read()) != -1){
char getSingleChar = (char)i;
System.out.print(getSingleChar);
}
}
}
输出:
This is a sample string
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn