在 Java 中删除一个对象
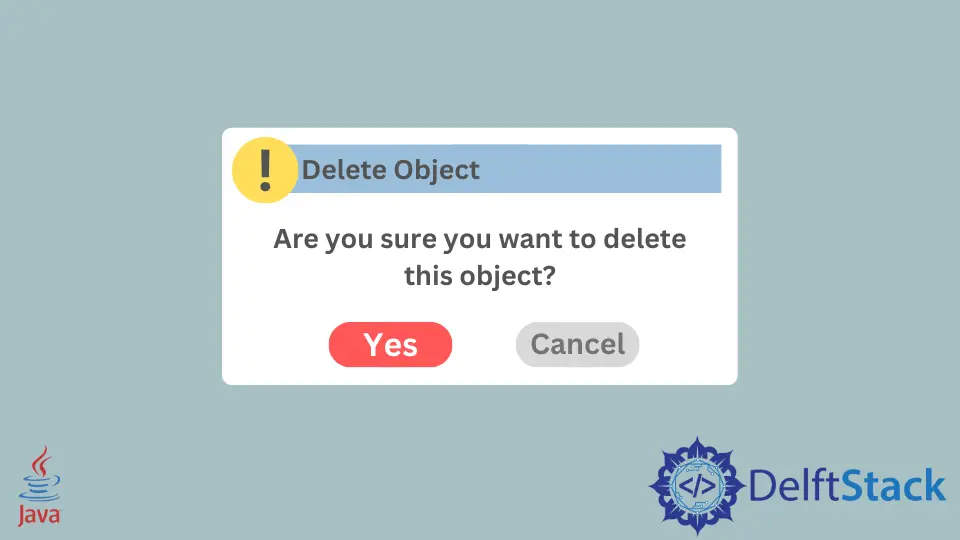
我们将在下面的例子中学习如何在 Java 中删除一个对象和使用垃圾回收器。
Java 通过引用 null
来删除对象
在第一个删除 Java 对象的例子中,我们创建了一个带有构造函数的类,该构造函数初始化了变量 name
和 age
。在 main
方法中,创建了一个 User
类的对象,并传递了 name
和 age
的值。现在,object
已经被初始化,我们可以用它来获取 age
,并进行比较来显示一条消息。但是在这之后,我们要删除 myObject
,以便不再使用。
要做到这一点,我们再次初始化 myObject
,但需要使用 null
。一旦 myObject
是 null
,我们就不能使用它来调用它的任何方法或变量,如果我们这样做,它将抛出一个异常。System.gc()
用于收集任何剩余的垃圾,这样就不会有空字段或对象剩下来释放内存。
我们可以看到,在 User
类中有一个 finalize()
方法。它是一个重载方法,在收集垃圾之前对对象进行调用。
public class JavaDeleteObject {
public static void main(String[] args) {
User myObject = new User("John", 30);
int userAge = myObject.age;
if (userAge > 25) {
System.out.println("User age is : " + userAge);
} else {
System.out.println("User age is less than 25 : " + userAge);
}
myObject = null;
System.gc();
System.out.println(myObject.age);
}
}
class User {
String name;
int age;
User(String n, int a) {
name = n;
age = a;
}
protected void finalize() {
System.out.println("Object is garbage collected.");
}
}
输出:
User age is : 30
Object is garbage collected.
Exception in thread "main" java.lang.NullPointerException
at com.company.JavaDeleteObject.main(JavaDeleteObject.java:18)
Java 通过将对象赋值为 null
来删除一个在限制范围内的对象
在下面的代码中,我们有一个 Student
类,它有三个数据成员,这些数据成员使用该类的参数化构造函数赋值。它有一个 showDetails()
方法和 showNextIndex()
方法。它还有 finalize()
方法来显示下一个学生的索引。
Student
类的新对象是用 new
关键字创建的。Student
类的方法在 student1
和 student2
对象上被调用。在后面的代码中,我们已经在一个范围内创建了更多的 Student
类对象。在使用它们之后,我们调用 System.gc()
,要求 JVM
对这个作用域内创建的两个对象进行垃圾回收。
在进入作用域之前,nextIndex
值会递增到 3
,在离开作用域之前是 5
。为了减少 nextIndex
值,在对象被垃圾收集之前,使用 System.runFinalization()
方法对 5
值调用两次。
然后显示正确的 nextIndex
值,不包括范围内的值。
class Student {
private int index;
private String name;
private int age;
private static int nextIndex = 1;
Student(String name, int age) {
this.name = name;
this.age = age;
this.index = nextIndex++;
}
public void showDetails() {
System.out.println("Student at Index : " + index + ", Student name: " + name + ", age: " + age);
}
public void showNextIndex() {
System.out.println("Next Index: " + nextIndex);
}
protected void finalize() {
--nextIndex;
}
}
class UseStudent {
public static void main(String[] args) {
Student student1 = new Student("John", 23);
Student student2 = new Student("Doe", 20);
student1.showDetails();
student2.showDetails();
student1.showNextIndex();
student2.showNextIndex();
{
Student student3 = new Student("Sam", 22);
Student student4 = new Student("Ben", 21);
student3.showDetails();
student4.showDetails();
student3.showNextIndex();
student4.showNextIndex();
student3 = student4 = null;
System.gc();
System.runFinalization();
}
student2.showNextIndex();
}
}
输出:
Student at Index : 1, Student name: John, age: 23
Student at Index : 2, Student name: Doe, age: 20
Next Index: 3
Next Index: 3
Student at Index : 3, Student name: Sam, age: 22
Student at Index : 4, Student name: Ben, age: 21
Next Index: 5
Next Index: 5
Next Index: 3
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn