Java 中 break 和 continue 语句的区别
-
通过 Java 中的
for
循环演示 Break 和 Continue 之间的区别 -
通过 Java 中的
foreach
循环演示 Break 和 Continue 之间的区别 - 通过 Java 中的嵌套循环演示 Break 和 Continue 之间的区别
- 演示 Java 中带标签的 break 和带标签的 continue 语句之间的区别
-
演示 Java 中
switch
条件中break
和continue
的使用
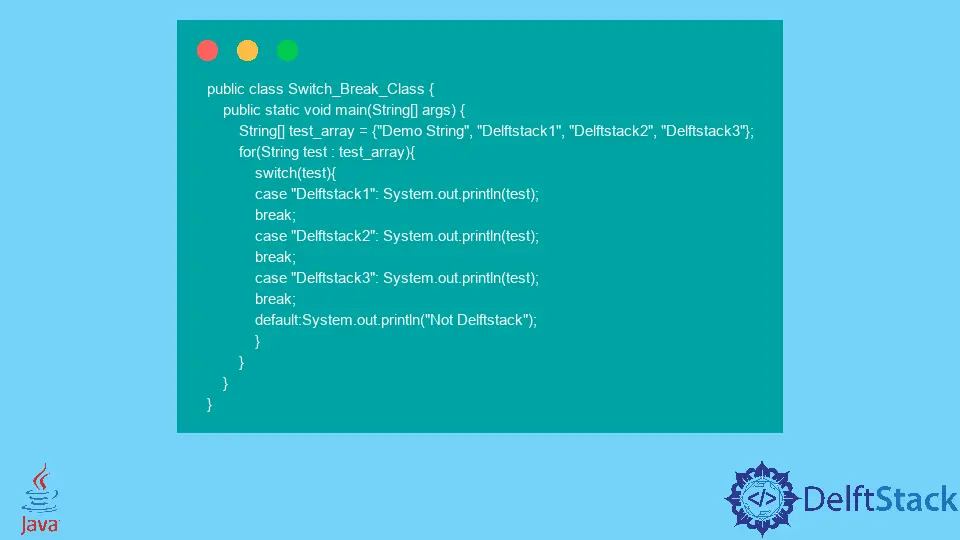
本教程将演示 Java 的 break
和 continue
语句之间的区别。
Java 中的 break
语句用于在给定条件下离开循环,而 continue
语句用于在给定条件下跳转循环的迭代。
通过 Java 中的 for
循环演示 Break 和 Continue 之间的区别
请参阅下面的示例,以使用 for
循环区分 break
和 continue
。
public class Break_Continue_Class {
public static void main(String args[]) {
System.out.println("The Break Statement: \n");
for (int x = 0; x < 15; x++) {
if (x == 8) {
break;
}
System.out.println(x);
}
System.out.println("The Continue Statement: \n");
for (int x = 0; x < 15; x++) {
if (x == 8) {
continue;
}
System.out.println(x);
}
}
}
输出:
The Break Statement:
0
1
2
3
4
5
6
7
The Continue Statement:
0
1
2
3
4
5
6
7
9
10
11
12
13
14
带有 break
语句的循环在 8 处终止,而带有 continue
的循环在 8 处跳转迭代。对于 while
和 do-while
循环的工作方式类似。
通过 Java 中的 foreach
循环演示 Break 和 Continue 之间的区别
请参阅下面的示例以区分 foreach
循环的 break
和 continue
语句。
public class Break_Continue_Class {
public static void main(String args[]) {
int[] test_array = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
System.out.println("The Break statement works in this way: \n");
for (int test : test_array) {
if (test == 5) {
break;
}
System.out.print(test);
System.out.print("\n");
}
System.out.println("The Continue statement works in this way: \n");
for (int test : test_array) {
if (test == 5) {
continue;
}
System.out.print(test);
System.out.print("\n");
}
}
}
输出:
The Break statement works in this way:
0
1
2
3
4
The Continue statement works in this way:
0
1
2
3
4
6
7
8
9
如图所示,带有 break
语句的循环在 5 处终止,带有 continue
的循环在 5 处跳转迭代。
通过 Java 中的嵌套循环演示 Break 和 Continue 之间的区别
下面的示例使用带有 break
和 continue
语句的嵌套 for
循环。
public class Break_Continue_Class {
public static void main(String[] args) {
System.out.println("The Break Statement: \n");
for (int x = 1; x <= 4; x++) {
for (int y = 1; y <= 4; y++) {
if (x == 3 && y == 3) {
break;
}
System.out.println(x + " " + y);
}
}
System.out.println("The Continue Statement: \n");
for (int x = 1; x <= 4; x++) {
for (int y = 1; y <= 4; y++) {
if (x == 3 && y == 3) {
continue;
}
System.out.println(x + " " + y);
}
}
}
}
输出:
The Break Statement:
1 1
1 2
1 3
1 4
2 1
2 2
2 3
2 4
3 1
3 2
4 1
4 2
4 3
4 4
The Continue Statement:
1 1
1 2
1 3
1 4
2 1
2 2
2 3
2 4
3 1
3 2
3 4
4 1
4 2
4 3
4 4
正如我们所见,break
语句仅中断 x 和 y 均为 3 的内部循环,而 continue
语句仅跳过了一次迭代,其中 x 和 y 均为 3。
演示 Java 中带标签的 break 和带标签的 continue 语句之间的区别
未标记的 break
和 continue
语句仅适用于嵌套循环中的最内层循环。这些标签仅用于将声明应用于我们的选择。
class Break_Continue_Labeled {
public static void main(String args[]) {
System.out.println("The Labeled Break Statement: ");
first_break: // First label
for (int x = 0; x < 4; x++) {
second_break: // Second label
for (int y = 0; y < 4; y++) {
if (x == 2 && y == 2) {
// Using break statement with label
break first_break;
}
System.out.println(x + " " + y);
}
}
System.out.println("The Labeled Continue Statement: ");
first_continue: // First label
for (int x = 0; x < 4; x++) {
second_continue: // Second label
for (int y = 0; y < 4; y++) {
if (x == 2 && y == 2) {
// Using break statement with label
continue first_continue;
}
System.out.println(x + " " + y);
}
}
}
}
输出:
The Labeled Break Statement:
0 0
0 1
0 2
0 3
1 0
1 1
1 2
1 3
2 0
2 1
The Labeled Continue Statement:
0 0
0 1
0 2
0 3
1 0
1 1
1 2
1 3
2 0
2 1
3 0
3 1
3 2
3 3
上面的代码使用标签作为第一和第二。如果我们将 first 作为参数传递给语句,它将应用于第一个语句,如果我们传递 second,它将应用于第二个语句。
演示 Java 中 switch
条件中 break
和 continue
的使用
只有 break
语句用于 switch
条件; continue
语句没有用处。
public class Switch_Break_Class {
public static void main(String[] args) {
String[] test_array = {"Demo String", "Delftstack1", "Delftstack2", "Delftstack3"};
for (String test : test_array) {
switch (test) {
case "Delftstack1":
System.out.println(test);
break;
case "Delftstack2":
System.out.println(test);
break;
case "Delftstack3":
System.out.println(test);
break;
default:
System.out.println("Not Delftstack");
}
}
}
}
输出:
Not Delftstack
Delftstack1
Delftstack2
Delftstack3
上面的代码包含三种情况和一种默认情况,它显示了开关条件中的 break
语句。
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook