如何在 Java 中遍历 Map 的每个元素
Mohammad Irfan
2023年1月30日
2020年9月26日
-
在 Java 中如何遍历
Map
元素 -
在 Java 中使用
for
循环遍历Map
元素 -
在 Java 中使用
foreach
遍历Map
元素 -
使用 Java 中的
Entry
和Iterator
遍历Map
元素 -
使用 Java 中的
for-each
和keySet()
遍历Map
元素 -
在 Java 中使用
while
循环遍历Map
元素 -
使用 Java 中的
Stream
和forEach
对Map
元素进行遍历 -
在 Java 中使用
forEach
和lambda
遍历Map
元素
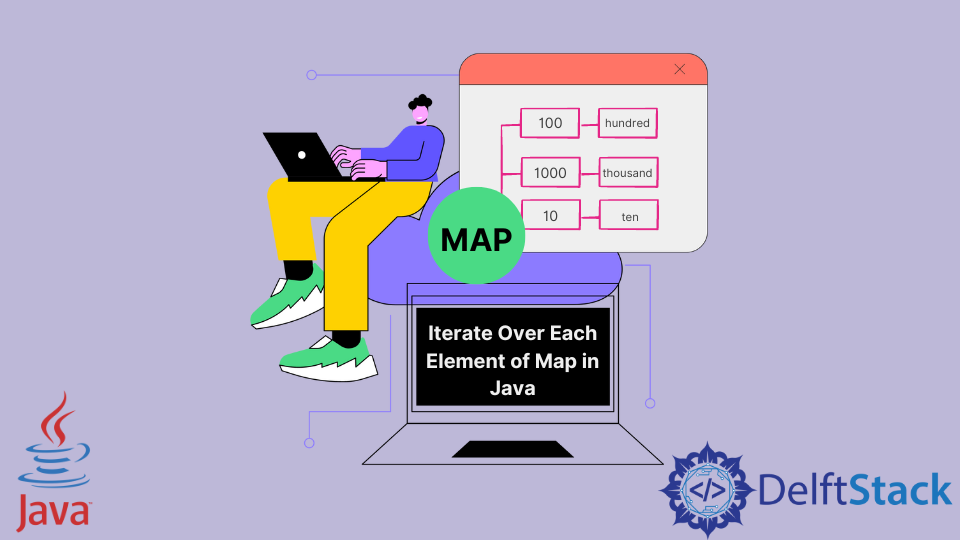
本教程介绍了如何对 map 的各个元素进行遍历,并列举了一些示例代码来理解。
在 Java 中如何遍历 Map
元素
Map 是一个接口,用来收集键值对形式的数据。Java 提供了几种遍历 map 元素的方法,如 for
循环、for-each
循环、while
循环、forEach()
方法等。我们来看看例子。
在 Java 中使用 for
循环遍历 Map
元素
我们使用简单的 for
循环来遍历 Map
元素。在这里,在循环中使用 iterator()
方法来获取元素。
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting{
public static void main(String[] args){
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
for (Iterator<Map.Entry<Integer, String>> entries = map.entrySet().iterator(); entries.hasNext(); ) {
Map.Entry<Integer, String> entry = entries.next();
System.out.println(entry.getKey()+" : "+entry.getValue());
}
}
}
输出:
100 : Hundred
1000 : Thousand
10 : Ten
在 Java 中使用 foreach
遍历 Map
元素
我们使用 for-each
循环和 entrySet()
方法来遍历 Map
的每个条目。entrySet()
返回 Map
的元素集合。
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting{
public static void main(String[] args){
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
for (Map.Entry<Integer, String> entry : map.entrySet()) {
System.out.println(entry.getKey() + ":" + entry.getValue());
}
}
}
输出:
100 : Hundred
1000 : Thousand
10 : Ten
使用 Java 中的 Entry
和 Iterator
遍历 Map
元素
iterator()
方法返回一个 Iterator
来遍历元素,而 Entry
用于收集 Map
的元素。
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting{
public static void main(String[] args){
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
Iterator<Map.Entry<Integer, String>> entries = map.entrySet().iterator();
while (entries.hasNext()) {
Map.Entry<Integer, String> entry = entries.next();
System.out.println(entry.getKey() + ":" + entry.getValue());
}
}
}
输出:
100 : Hundred
1000 : Thousand
10 : Ten
使用 Java 中的 for-each
和 keySet()
遍历 Map
元素
keySet()
方法用于收集 Map
的键集,进一步使用 for-each
循环进行遍历。
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting{
public static void main(String[] args){
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
for (Integer key : map.keySet()) {
System.out.println(key +" : "+map.get(key));
}
}
}
输出:
100 : Hundred
1000 : Thousand
10 : Ten
在 Java 中使用 while
循环遍历 Map
元素
在这里,我们使用 iterator()
方法来获取键的遍历器,然后使用 while
循环来遍历这些键。为了获得键的值,我们使用 get()
方法。
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting{
public static void main(String[] args){
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
Iterator<Integer> itr = map.keySet().iterator();
while (itr.hasNext()) {
Integer key = itr.next();
System.out.println(key +" : "+map.get(key));
}
}
}
输出:
100 : Hundred
1000 : Thousand
10 : Ten
使用 Java 中的 Stream
和 forEach
对 Map
元素进行遍历
我们可以使用流来遍历元素。在这里,我们使用 entrySet()
来收集 Map
元素,并通过流的 forEach()
方法进一步遍历。
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting{
public static void main(String[] args){
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
map.entrySet()
.stream()
.forEach(System.out::println);
}
}
输出:
100=Hundred
1000=Thousand
10=Ten
在 Java 中使用 forEach
和 lambda
遍历 Map
元素
我们也可以使用 lambda 表达式来遍历 Map
元素。这里,我们在 forEach()
方法中使用了 lambda
表达式。
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting{
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
map.forEach((key, value) -> System.out.println(key + " : " + value));
}
}
输出:
100 : Hundred
1000 : Thousand
10 : Ten
相关文章 - Java Map
- Java 中的映射过滤
- 在 Java 中将 Stream 元素转换为映射
- 在 Java 中将列表转换为 map
- 在 Java 中将 map 值转换为列表
- 用 Java 创建 map
- 在 Java 中创建有序映射