Java 消息格式
-
利用
MessageFormat
使用双引号格式化消息 -
利用
MessageFormat
使用Unicode
字符格式化消息 -
利用
MessageFormat
使用转义序列格式化消息 -
通过使用
MessageFormat
替换字符来格式化消息
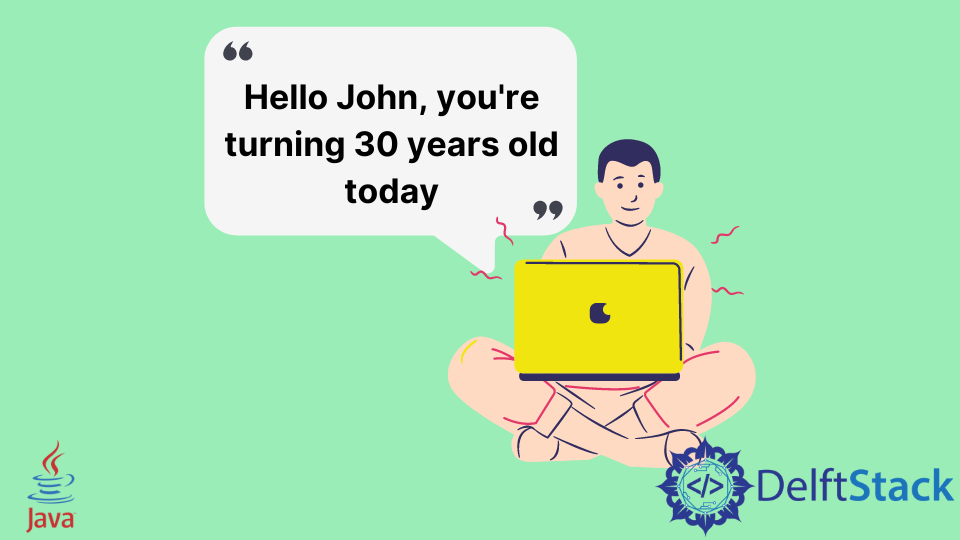
在本教程中,我们将学习如何使用 Java API 提供的 Java MessageFormat
类来格式化消息。格式化是将字符和字符串动态插入消息的过程,而 MessageFormat
为我们提供了此功能。
MessageFormat
使用对象参数来形成格式化字符串,该字符串使用显示对象参数插入位置的模式。
对象参数根据它们在 MessageFormat
对象参数中传递的位置插入到模式中。我们将使用 MessageFormat
的静态 format()
方法来学习如何格式化消息。
传递给 format()
方法的第一个参数是我们的模式,然后是基于我们想要动态插入字符串中的字符串说明符的对象参数。当向我们的模式中插入一个元素时,我们可能会使用 MessageFormat
以不同方式解释的某些字符,这可能会导致与预期不同的结果。
例如,在使用 MessageFormat
格式化的消息中使用诸如 you're
的字符串中的单引号会产生字符串 youre
。单引号用于表示不会格式化的部分,请注意以下示例中消息的剩余模式未格式化。
import java.text.MessageFormat;
public class FormatString {
public static void main(String[] args) {
String name = "John";
long age = 30;
String message = MessageFormat.format(
"Hello {0}, you're turning {1} years old today",
name,
age);
System.out.println(message);
}
}
输出:
Hello John, youre turning {1} years old today
在接下来的部分中,我们将介绍四种不同的方法,我们可以使用这些方法在消息中插入单引号,以确保我们的模式被成功格式化。
利用 MessageFormat
使用双引号格式化消息
要在使用 MessageFormat
格式化的消息中添加单引号,我们应该在字符串中使用两个单引号''
而不是单引号'
,如下所示。
import java.text.MessageFormat;
public class FormatString {
public static void main(String[] args) {
String name = "John";
long age = 30;
String message = MessageFormat.format(
"Hello {0}, you''re turning {1} years old today",
name,
age);
System.out.println(message);
}
}
输出:
Hello John, you're turning 30 years old today
请注意,添加双引号后插入单引号,并将剩余的模式格式化为正确的值。
利用 MessageFormat
使用 Unicode
字符格式化消息
每个字符都有一个 Unicode 表示。由于 Java 可以读取 Unicode 字符,我们可以通过使用单引号的 Unicode 表示在字符串中插入单引号。
import java.text.MessageFormat;
public class FormatString {
public static void main(String[] args) {
String name = "John";
long age = 30;
String message = MessageFormat.format(
"Hello {0}, you\u2019re turning {1} years old today",
name,
age);
System.out.println(message);
}
}
输出:
Hello John, you're turning 30 years old today
Unicode 字符 \u2019
在我们的字符串中添加了一个单引号,并且我们消息的其余模式被格式化为正确的值。
利用 MessageFormat
使用转义序列格式化消息
此方法类似于使用双引号的方法,但使用转义序列\
,它是一个前面带有反斜杠的字符,用于插入一系列字符。我们可以通过在我们的模式中插入两个引号转义序列来插入一个单引号,如下所示。
import java.text.MessageFormat;
public class FormatString {
public static void main(String[] args) {
String name = "John";
long age = 30;
String message = MessageFormat.format(
"Hello {0}, you\'\'re turning {1} years old today",
name,
age);
System.out.println(message);
}
}
输出:
Hello John, you're turning 30 years old today
通过使用 MessageFormat
替换字符来格式化消息
由于我们使用的是字符串,我们可以让我们的字符串保持不变并使用 replaceAll()
方法将单引号替换为双引号,最终在我们的字符串中添加一个单引号。
import java.text.MessageFormat;
public class FormatString {
public static void main(String[] args) {
String name = "John";
long age = 30;
String message = MessageFormat.format(
"Hello {0}, you're turning {1} years old today"
.replaceAll("'","''"),
name,
age);
System.out.println(message);
}
}
输出:
Hello John, you're turning 30 years old today
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub