Java 中的守护线程
Mohammad Irfan
2023年1月30日
2021年3月21日
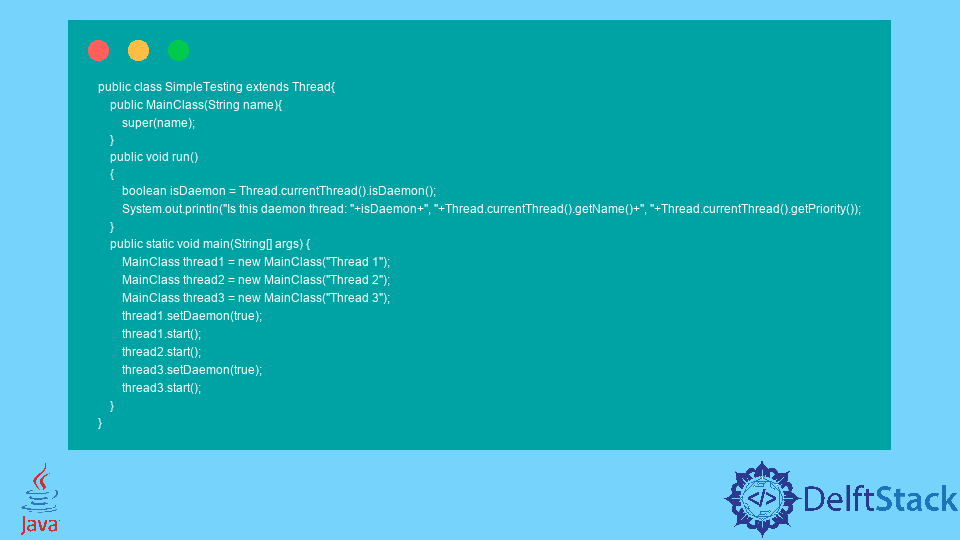
本教程将介绍什么是守护线程以及如何在 Java 中创建守护线程。
在 Java 中,守护线程是一种特殊线程,支持其他线程的后台线程。Java 使用这些线程来为用户线程和垃圾回收等提供特殊用途。
为了创建守护线程,Java 提供了带有布尔参数的 setDaemon()
方法。isDaemon()
方法可以检查当前正在运行的线程是否是 Daemon 线程。
在 Java 中使用 setDaemon()
方法创建守护线程
在此示例中,我们使用 Thread
类的 setDaemon()
方法创建守护线程。我们创建了三个线程,其中两个被设置为守护线程。我们使用 isDaemon()
方法检查当前正在运行的线程是否是守护线程。请参见下面的示例。
public class SimpleTesting extends Thread{
public MainClass(String name){
super(name);
}
public void run()
{
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: "+isDaemon);
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.setDaemon(true);
thread1.start();
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
输出:
Is this daemon thread: true
Is this daemon thread: false
Is this daemon thread: true
使用 Java 中的 setDaemon()
方法创建守护线程
守护线程是低优先级线程,并且始终在用户线程之后运行。通过使用返回整数值的 getPriority()
方法,我们可以看到线程的优先级。我们使用 getName()
方法来获取当前正在运行的线程的名称。请参见以下示例。
public class SimpleTesting extends Thread{
public MainClass(String name){
super(name);
}
public void run()
{
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: "+isDaemon+", "+Thread.currentThread().getName()+", "+Thread.currentThread().getPriority());
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.setDaemon(true);
thread1.start();
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
输出:
Is this daemon thread: true, Thread 1, 5
Is this daemon thread: false, Thread 2, 5
Is this daemon thread: true, Thread 3, 5
使用 Java 中的 setDaemon()
方法创建守护线程
我们在创建守护线程时将值设置为 setDaemon()
方法后启动线程。这一点是非常必要的,因为如果我们将一个已经在运行的线程设置为守护线程,它将抛出异常。我们用 thread1
调用 start()
方法,然后调用 setDaemon()
,这是不允许的。请参见以下示例。
public class SimpleTesting extends Thread{
public MainClass(String name){
super(name);
}
public void run()
{
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: "+isDaemon+", "+Thread.currentThread().getName()+", "+Thread.currentThread().getPriority());
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.start();
thread1.setDaemon(true);
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
输出:
Is this daemon thread: false, Thread 1, 5
Exception in thread "main" java.lang.IllegalThreadStateException