获取 Java 中文件的行数
Farkhanda Athar
2023年10月12日
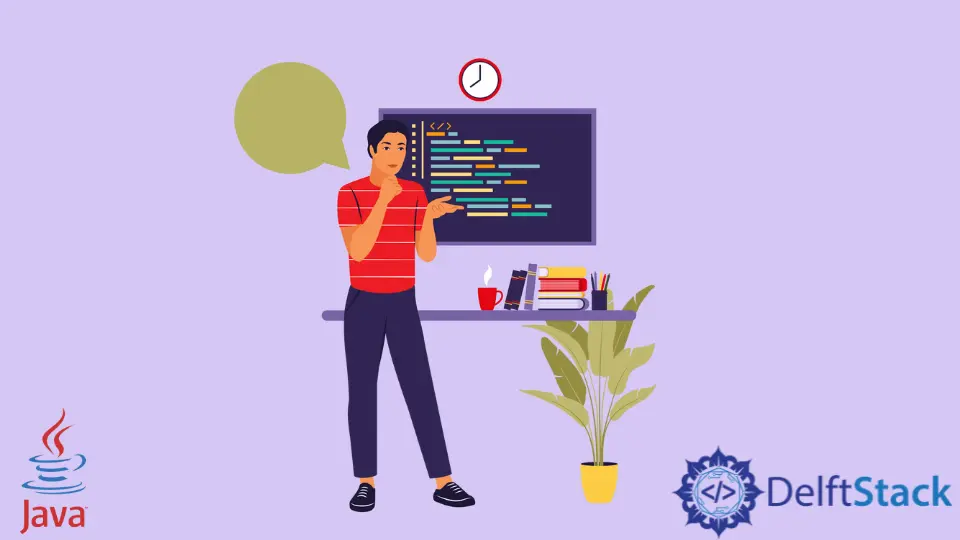
本文将解释计算文件中总行数的各种方法。
计算某文件中的行数的过程包括四个步骤:
- 打开文件。
- 逐行读取并在每行后将计数加一。
- 关闭文件。
- 读取计数。
这里我们使用了两种方法来计算文件中的行数。这些方法是 Java File
和 Scanner
类。
使用 Java 中的 Scanner
类计算文件中的行数
在这种方法中,使用了 Scanner
类的 nextLine()
方法,该方法访问文件的每一行。行数取决于 input.txt
文件中的行数。该程序还打印文件内容。
示例代码:
import java.io.File;
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int count = 0;
try {
File file = new File("input.txt");
Scanner sc = new Scanner(file);
while (sc.hasNextLine()) {
sc.nextLine();
count++;
}
System.out.println("Total Number of Lines: " + count);
sc.close();
} catch (Exception e) {
e.getStackTrace();
}
}
}
如果文件由三行组成,如下所示。
This is the first line.This is the second line.This is the third line.
然后输出将是,
输出:
Total Number of Lines: 3
使用 java.nio.file
包计算文件中的行数
为此,lines()
方法会将文件的所有行作为流读取,而 count()
方法将返回流中的元素数。
示例代码:
import java.nio.file.*;
class Main {
public static void main(String[] args) {
try {
Path file = Paths.get("input.txt");
long count = Files.lines(file).count();
System.out.println("Total Lines: " + count);
} catch (Exception e) {
e.getStackTrace();
}
}
}
输出:
Total Lines: 3