在 Java 中将 JSON 转换为 XML
Mohammad Irfan
2023年10月12日
- 使用 Java 中的 org.json 库将 JSON 转换为 XML
-
使用 Java 中的
underscore
库将 JSON 转换为 XML - 使用 Java 中的 Jackson 库将 JSON 转换为 XML
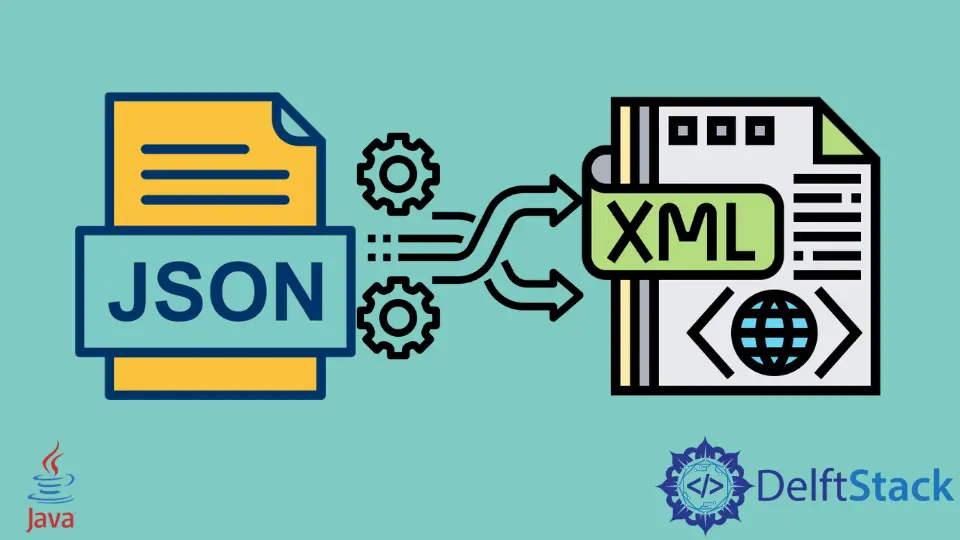
本教程介绍如何在 Java 中将 JSON 转换为 XML。
要将 JSON 转换为 XML,我们可以使用多个库,例如 org.json、下划线和 Jackson。在本文中,我们将学习使用这些库并查看 JSON 到 XML 的转换过程。
JSON 是应用程序用于数据交换的数据格式。由于轻量级和简单,它被应用程序使用,而 XML 是一种标记语言,也用于传输数据。
让我们从一些在 Java 中将 JSON 转换为 XML 的示例开始。
使用 Java 中的 org.json 库将 JSON 转换为 XML
在这个例子中,我们使用了 org.json 库,它提供了 JSONObject
和一个 XML 类。JSONObject 类用于将 JSON 字符串转换为 JSON 对象,然后通过使用 XML 类将 JSON 转换为 XML。
XML 类提供了一个静态的 toString() 方法,该方法将结果作为字符串返回。请参阅下面的示例。
import java.io.IOException;
import org.json.*;
public class SimpleTesting {
public static void main(String[] args) throws IOException, JSONException {
String jsonStr = "{student : { age:30, name : Kumar, technology : Java } }";
JSONObject json = new JSONObject(jsonStr);
String xml = XML.toString(json);
System.out.println(xml);
}
}
输出:
<student>
<name>Kumar</name>
<technology>Java</technology>
<age>30</age>
</student>
使用 Java 中的 underscore
库将 JSON 转换为 XML
在这里,我们使用 underscore
库将 JSON
转换为 XML
。我们使用了 U
类及其静态方法 jsonToXml()
,它以字符串形式返回 XML
。请参阅下面的示例。
import com.github.underscore.lodash.U;
import java.io.IOException;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
String jsonStr =
"{\"name\":\"JSON\",\"integer\":1,\"double\":2.0,\"boolean\":true,\"nested\":{\"id\":42},\"array\":[1,2,3]}";
System.out.println(jsonStr);
String xml = U.jsonToXml(jsonStr);
System.out.println(xml);
}
}
输出:
{"name":"JSON","integer":1,"double":2.0,"boolean":true,"nested":{"id":42},"array":[1,2,3]}
<?xml version="1.0" encoding="UTF-8"?>
<root>
<name>JSON</name>
<integer number="true">1</integer>
<double number="true">2.0</double>
<boolean boolean="true">true</boolean>
<nested>
<id number="true">42</id>
</nested>
<array number="true">1</array>
<array number="true">2</array>
<array number="true">3</array>
</root>
使用 Java 中的 Jackson 库将 JSON 转换为 XML
在这里,我们使用 ObjectMapper
类读取 JSON,然后使用 XmlMapper
类获取 XML 格式的数据。在这里,我们使用了两个主要的包,jackson.databind
和 jackson.dataformat
;第一个包含与 JSON 相关的类,第二个包含与 XML 相关的类。请参阅下面的示例。
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.dataformat.xml.ser.ToXmlGenerator;
import java.io.IOException;
import java.io.StringWriter;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
final String jsonStr =
"{\"name\":\"JSON\",\"integer\":1,\"double\":2.0,\"boolean\":true,\"nested\":{\"id\":42},\"array\":[1,2,3]}";
ObjectMapper jsonMapper = new ObjectMapper();
JsonNode node = jsonMapper.readValue(jsonStr, JsonNode.class);
XmlMapper xmlMapper = new XmlMapper();
xmlMapper.configure(SerializationFeature.INDENT_OUTPUT, true);
xmlMapper.configure(ToXmlGenerator.Feature.WRITE_XML_DECLARATION, true);
xmlMapper.configure(ToXmlGenerator.Feature.WRITE_XML_1_1, true);
StringWriter sw = new StringWriter();
xmlMapper.writeValue(sw, node);
System.out.println(sw.toString());
}
}
输出:
{"name":"JSON","integer":1,"double":2.0,"boolean":true,"nested":{"id":42},"array":[1,2,3]}
<?xml version="1.0" encoding="UTF-8"?>
<root>
<name>JSON</name>
<integer number="true">1</integer>
<double number="true">2.0</double>
<boolean boolean="true">true</boolean>
<nested>
<id number="true">42</id>
</nested>
<array number="true">1</array>
<array number="true">2</array>
<array number="true">3</array>
</root>