在 Go 的 POST 请求中发送 JSON 字符串
Jay Singh
2023年1月30日
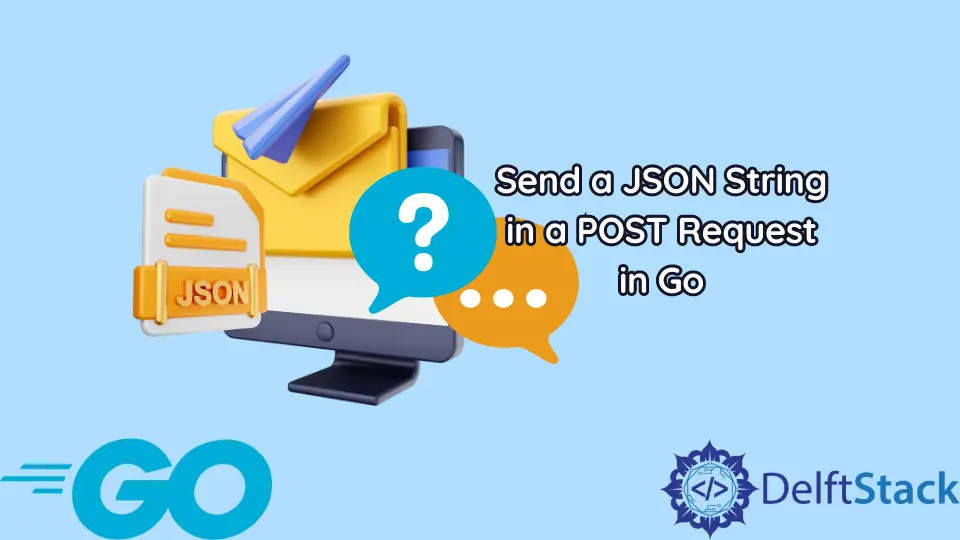
JavaScript Object Notation (JSON) 是 Web 开发中常用的数据传输格式。它易于使用和理解。
你可以使用 Go 语言创建 JSON POST
请求,但你需要导入多个 Go 包。net/HTTP
包包括良好的 HTTP 客户端和服务器支持。
Go 中的 JSON
包还提供 JSON 编码和解码。
在本教程中,你将学习如何使用 Go 语言执行 JSON POST 请求。在本教程中,你将学习如何使用 Go 语言将 JSON 字符串作为 POST 请求传递。
在 Go 的 POST 请求中发送 JSON 字符串
下面显示了一个包含课程和路径列表的基本 JSON 文件。
{
"Courses": [
"Golang",
"Python"
],
"Paths": [
"Coding Interviews",
"Data Structure"
]
}
下面的代码显示了如何将 USER JSON 对象提交给服务 reqres.in
以构造用户请求。
package main
import (
"bytes"
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
)
type User struct {
Name string `json:"name"`
Job string `json:"job"`
}
func main(){
user := User{
Name: "Jay Singh",
Job: "Golang Developer",
}
body, _ := json.Marshal(user)
resp, err := http.Post("https://reqres.in/api/users", "application/json", bytes.NewBuffer(body) )
if err != nil {
panic(err)
}
defer resp.Body.Close()
if resp.StatusCode == http.StatusCreated {
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
jsonStr := string(body)
fmt.Println("Response: ", jsonStr)
} else {
fmt.Println("Get failed with error: ", resp.Status)
}
}
输出:
Response: {
"name":"Jay Singh",
"job":"Golang Developer",
"id":"895",
"createdAt":"2022-04-04T10:46:36.892Z"
}
在 Golang 的 POST 请求中发送 JSON 字符串
这是简单的 JSON 代码。
{
"StudentName": "Jay Singh",
"StudentId" : "192865782",
"StudentGroup": "Computer Science"
}
package main
import (
"bytes"
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
)
type StudentDetails struct {
StudentName string `json:"StudentName"`
StudentId string `json:"StudentId"`
StudentGroup string `json:"StudentGroup"`
}
func main() {
studentDetails := StudentDetails{
StudentName: "Jay Singh",
StudentId: "192865782",
StudentGroup: "Computer Science",
}
body, _ := json.Marshal(studentDetails)
resp, err := http.Post("<https://reqres.in/api/users>", "application/json", bytes.NewBuffer(body))
if err != nil {
panic(err)
}
defer resp.Body.Close()
if resp.StatusCode == http.StatusCreated {
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
jsonStr := string(body)
fmt.Println("Response: ", jsonStr)
} else {
fmt.Println("Get failed with error: ", resp.Status)
}
}
输出:
Response: {
"StudentName":"Deven Rathore",
"StudentId":"170203065",
"StudentGroup":"Computer Science",
"id":"868",
"createdAt":"2022-04-04T12:35:03.092Z"
}