在 C# 中调整图像大小
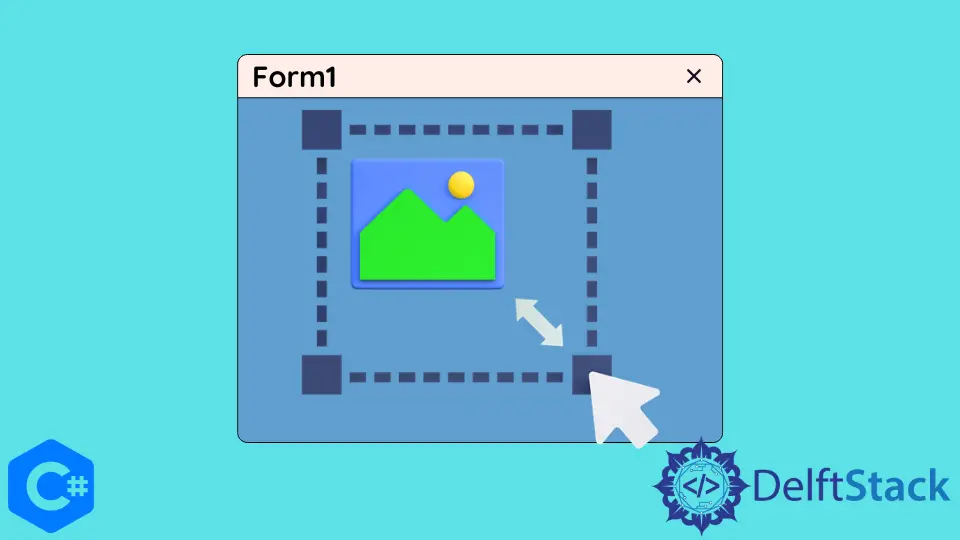
在本教程中,我们将讨论在C#中调整图像大小的方法。
我们将带您完成整个过程,从加载原始图像到保存调整大小后的版本。
使用C#
中的Bitmap
类调整图像大小
Bitmap
类提供了许多在C#中使用图像的方法。Bitmap
类获取图像的像素数据。我们可以通过在Bitmap
类的构造函数中初始化Size
参数来调整图像的大小。
以下代码示例展示了如何在C#中使用Bitmap
类的构造函数调整图像大小。
using System;
using System.Drawing;
namespace resize_image {
class Program {
public static Image resizeImage(Image imgToResize, Size size) {
return (Image)(new Bitmap(imgToResize, size));
}
static void Main(string[] args) {
string path = "C:\\Images\\img1.jpg";
Image img = Image.FromFile(path);
Bitmap imgbitmap = new Bitmap(img);
Image resizedImage = resizeImage(imgbitmap, new Size(200, 200));
}
}
}
这段代码演示了使用C#
中的Bitmap
类调整图像大小的完整过程。让我们来分解一下它是如何工作的。
步骤1:加载原始图像
首先,您需要使用Image.FromFile
方法加载原始图像。请确保将"C:\\Images\\img1.jpg"
替换为您自己图像文件的路径。
string path = "C:\\Images\\img1.jpg";
Image img = Image.FromFile(path);
步骤2:将图像转换为位图
接下来,您将加载的图像转换为Bitmap
对象。这一步是执行调整大小操作所必需的。
Bitmap imgBitmap = new Bitmap(img);
步骤3:调整图像大小
现在是调整大小的部分。ResizeImage
函数接受Bitmap
对象和所需的大小(new Size(200, 200)
),并返回调整大小后的图像。
Image resizedImage = ResizeImage(imgBitmap, new Size(200, 200));
步骤4:保存调整大小后的图像
最后,您可以使用Save
方法将调整大小后的图像保存到文件中。在此示例中,调整大小后的图像保存为"resized.jpg"
。您可以通过更改文件扩展名(例如.jpg
、.png
、.bmp
)选择保存图像的格式。
resizedImage.Save("resized.jpg");
使用C#
中的Graphics.DrawImage()
函数调整图像大小
Graphics.DrawImage()
函数在C#中指定位置和指定尺寸内绘制图像。使用此方法,我们可以消除调整图像大小的许多缺点。以下代码示例展示了如何在C#中使用Graphics.DrawImage()
函数调整图像大小。
using System;
using System.Drawing;
namespace resize_image {
class Program {
public static Image resizeImage(Image image, int width, int height) {
var destinationRect = new Rectangle(0, 0, width, height);
var destinationImage = new Bitmap(width, height);
destinationImage.SetResolution(image.HorizontalResolution, image.VerticalResolution);
using (var graphics = Graphics.FromImage(destinationImage)) {
graphics.CompositingMode = CompositingMode.SourceCopy;
graphics.CompositingQuality = CompositingQuality.HighQuality;
using (var wrapMode = new ImageAttributes()) {
wrapMode.SetWrapMode(WrapMode.TileFlipXY);
graphics.DrawImage(image, destinationRect, 0, 0, image.Width, image.Height,
GraphicsUnit.Pixel, wrapMode);
}
}
return (Image)destinationImage;
}
static void Main(string[] args) {
string path = "C:\\Images\\img1.jpg";
Image img = Image.FromFile(path);
Bitmap imgbitmap = new Bitmap(img);
Image resizedImage = resizeImage(imgbitmap, new Size(200, 200));
}
}
}
让我们来分解一下它是如何工作的。
步骤1:加载原始图像
首先,您需要使用Image.FromFile
加载原始图像。请确保将"C:\\Images\\img1.jpg"
替换为您自己图像文件的路径。
string path = "C:\\Images\\img1.jpg";
Image img = Image.FromFile(path);
步骤2:创建目标位图
创建一个目标Bitmap
对象,用于保存调整大小后的图像。使用SetResolution
将其分辨率设置为与原始图像相匹配。
destinationImage.SetResolution()
函数 会保持图像的 DPI,无论其实际大小如何。
var destinationRect = new Rectangle(0, 0, width, height);
var destinationImage = new Bitmap(width, height);
destinationImage.SetResolution(image.HorizontalResolution, image.VerticalResolution);
第三步:调整图像大小
现在,我们使用Graphics.FromImage
来获得与目标Bitmap
相关联的Graphics
对象。我们设置合成模式和质量以获得最佳结果。
using (var graphics = Graphics.FromImage(destinationImage)) {
graphics.CompositingMode = CompositingMode.SourceCopy;
graphics.CompositingQuality = CompositingQuality.HighQuality;
using (var wrapMode = new ImageAttributes()) {
wrapMode.SetWrapMode(WrapMode.TileFlipXY);
graphics.DrawImage(image, destinationRect, 0, 0, image.Width, image.Height, GraphicsUnit.Pixel,
wrapMode);
}
}
graphics.CompositingMode = CompositingMode.SourceCopy
属性 指定当呈现颜色时,其会覆盖背景颜色。
graphics.CompositingQuality = CompositingQuality.HighQuality
属性 指定我们只希望呈现高质量的图像。
wrapMode.SetWrapMode(WrapMode.TileFlipXY)
函数 避免图像边框周围出现残影。
第四步:保存调整大小后的图像
最后,您可以使用 Save
方法将调整大小后的图像保存到文件中。在这个例子中,调整大小后的图像保存为 "resized.jpg"
。您可以通过更改文件扩展名(例如 .jpg
, .png
, .bmp
)来选择保存图像的格式。
resizedImage.Save("resized.jpg");
结论
调整图像大小是软件开发中的常见任务,C# 提供了多种方法来实现此目的。在本文中,我们重点介绍了使用 Bitmap
类调整图像大小的方法。我们逐步讲解了从加载原始图像到保存调整大小版本的整个过程。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn