如何在 C# 函数中传递一个方法作为参数
Minahil Noor
2024年2月16日
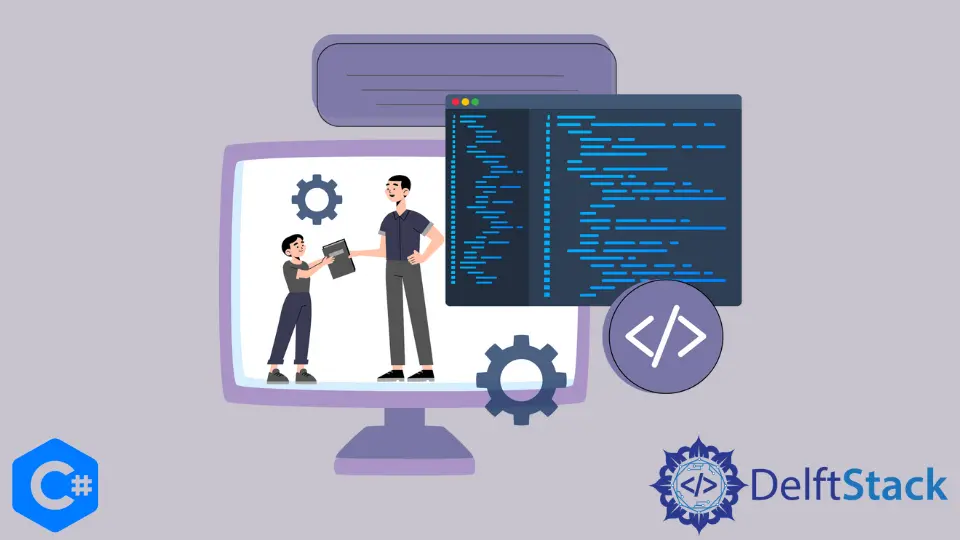
本文将介绍在 C# 函数中传递一个方法作为参数的不同方法。
- 使用
Func
委托 - 使用
Action
代表
在 C# 中使用 Func
代表传递一个方法作为参数
我们将使用内置的 Func
delegate 来传递一个方法作为参数。delegate
的作用就像一个函数指针。使用这个委托的正确语法如下。
public delegate returnType Func<in inputType, out returnType>(InputType arg);
内置的委派 Func
有 N 个参数。它的参数详情如下。
参数 | 说明 | |
---|---|---|
inputType |
强制 | 它是这个委托的输入值的类型,可以有 N 个输入。 |
returnType |
强制 | 它是返回值的类型。该委托的最后一个值被视为是返回类型。 |
下面的程序显示了我们如何使用 Func
委托来传递一个方法作为参数。
public class MethodasParameter {
public int Method1(string input) {
return 0;
}
public int Method2(string input) {
return 1;
}
public bool RunMethod(Func<string, int> MethodName) {
int i = MethodName("This is a string");
return true;
}
public bool Test() {
return RunMethod(Method1);
}
}
在 C# 中使用 Action
委派来传递一个方法作为参数
我们也可以使用内置的委托 Action
来传递一个方法作为参数。使用这个委托的正确语法如下。
public delegate void Action<in T>(T obj);
内置的委托人 Action
可以有 16 个参数作为输入。它的详细参数如下。
参数 | 说明 | |
---|---|---|
T |
强制 | 它是这个委托的输入值的类型。可以有 16 个输入值。 |
下面的程序显示了我们如何使用 Action
委托来传递一个方法作为参数。
public class MethodasParameter {
public int Method1(string input) {
return 0;
}
public int Method2(string input) {
return 1;
}
public bool RunTheMethod(Action myMethodName) {
myMethodName();
return true;
}
RunTheMethod(() => Method1("MyString1"));
}