C# 将字符串转换为日期时间
-
C# 使用
Convert.ToDateTime()
将字符串转换为DateTime
-
C# 使用
DateTime.Parse()
将字符串转换为DateTime
-
C# 使用
DateTime.ParseExact()
将string
转换为DateTime
- 结论
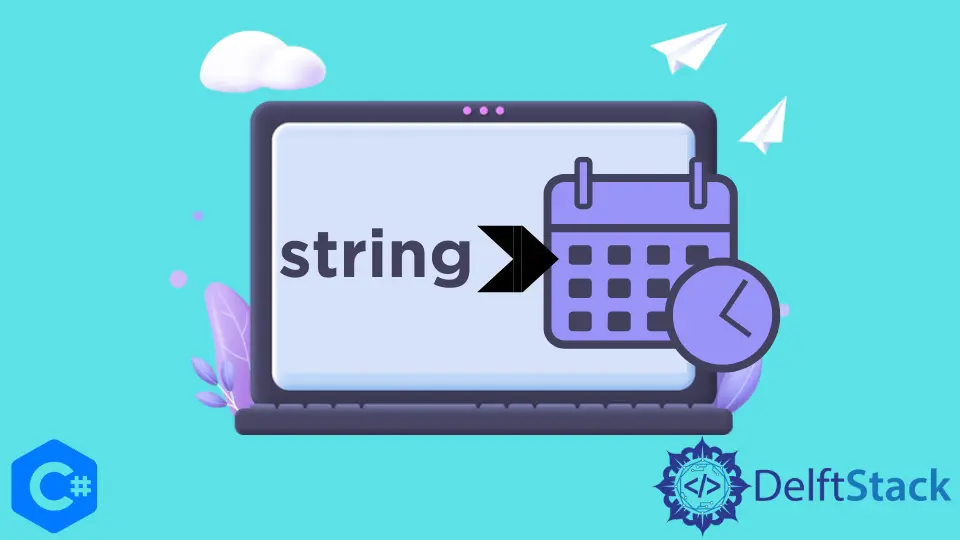
在大多数情况下,我们以字符串形式获取日期,并且希望分别使用日,月和年。不用担心,在 C# 中,为了将字符串转换为 DateTime
对象,我们使用了一个名为 DateTime
的预定义类。
有几种在 C# 中将字符串转换为 DateTime
的方法,但是在这里,我们仅通过运行示例详细说明三种方法。这些方法是 Convert.ToDateTime()
,DateTime.Parse()
和 DateTime.ParseExact()
。
C# 使用 Convert.ToDateTime()
将字符串转换为 DateTime
Convert.ToDateTime()
的正确语法是
Convert.ToDateTime(dateTobeConverted);
Convert.ToDateTime(dateTobeConverted, cultureInfo);
这里应该注意,如果你不提供区域性信息,那么默认情况下,编译器会将我们的日期字符串视为月/日/年。CultureInfo
是 System.Globalization
命名空间中的一个 C# 类,有关特定的 CultureInfo
。
using System;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Use of Convert.ToDateTime()
DateTime DateObject = Convert.ToDateTime(CurrentDate);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
输出:
The Date is: 4 6 2020
现在,我们将通过传递 CultureInfo
对象作为参数来实现它。
using System;
using System.Globalization;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Creating new CultureInfo Object
// You can use different cultures like French, Spanish etc.
CultureInfo Culture = new CultureInfo("en-US");
// Use of Convert.ToDateTime()
DateTime DateObject = Convert.ToDateTime(CurrentDate, Culture);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
输出:
The Date is: 4 6 2020
如果我们将 CultureInfo
更改为 nl-NL
,则将交换月份和日期。
using System;
using System.Globalization;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
CultureInfo Culture = new CultureInfo("nl-nl");
DateTime DateObject = Convert.ToDateTime(CurrentDate, Culture);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
输出:
The Date is: 6 4 2020
C# 使用 DateTime.Parse()
将字符串转换为 DateTime
DateTime.Parse()
的语法是,
DateTime.Parse(dateTobeConverted);
DateTime.Parse(dateTobeConverted, cultureInfo);
DateTime.Parse()
方法也是如此,如果我们不提供文化信息作为参数,那么默认情况下,我们的系统会将其视为月/日/年。
如果要转换的字符串的值为 null,则它将返回 ArgumentNullException
,应使用 try-catch
模块进行处理。
using System;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Use of DateTime.Parse()
DateTime DateObject = DateTime.Parse(CurrentDate);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
输出:
The Date is: 4 6 2020
C# 使用 DateTime.ParseExact()
将 string
转换为 DateTime
DateTime.ParseExact()
的语法是,
DateTime.ParseExact(dateTobeConverted, dateFormat, cultureInfo);
DateTime.ParseExact()
是将字符串转换为 DateTime
的最佳方法。在此方法中,我们将日期的格式作为参数传递。这使用户易于准确执行转换。
在这里,我们通过传递 null
作为参数来代替文化信息,因为它是一个全新的主题,并且需要花费一些时间来理解。
using System;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Use of DateTime.ParseExact()
// culture information is null here
DateTime DateObject = DateTime.ParseExact(CurrentDate, "dd/MM/yyyy", null);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
输出:
The Date is: 6 4 2020
结论
有许多方法可以在 C# 中将字符串转换为 DateTime
。将字符串转换为 DateTime
的最佳方法是 DateTime.ParseExact()
。
相关文章 - Csharp String
- C# 将字符串转换为枚举类型
- C# 中将整形 Int 转换为字符串 String
- 在 C# 中的 Switch 语句中使用字符串
- 如何在 C# 中把一个字符串转换为布尔值
- 如何在 C# 中把一个字符串转换为浮点数
- 如何在 C# 中编写多行字符串文字