C# 中的 goto 语句
Fil Zjazel Romaeus Villegas
2023年10月12日
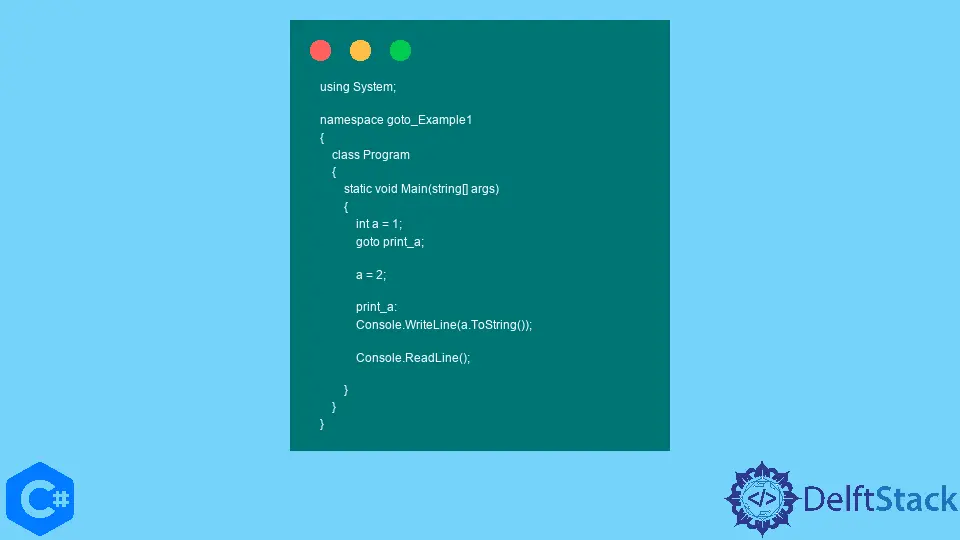
本教程将演示如何在 C# 中使用 goto
语法,并提供一些代码中的实际使用示例。
goto
是一个无条件跳转语句,一旦遇到程序将自动转到代码的新部分。要使用 goto
,你必须同时拥有一个由标签标记的语句和一个调用该标签的实例。
要创建标签,请在调用标签时要运行的语句之前添加其名称和冒号。
例子:
using System;
namespace goto_Example1 {
class Program {
static void Main(string[] args) {
int a = 1;
goto print_a;
a = 2;
print_a:
Console.WriteLine(a.ToString());
Console.ReadLine();
}
}
}
在本例中,我们将整数变量 a
初始化为等于 1。由于我们立即调用 goto
跳转到语句 print_a
,所以 a
永远不会设置为等于 2。因此,当我们将 a
的值打印到控制台时,发布的是 1 而不是 2。
输出:
1
何时在 C#
中使用 goto
然而,goto
现在并不常用,因为它被批评为恶化代码的可读性,因为如果它需要你跳转到完全不同的部分,逻辑流程就不那么清晰了。
然而,仍有一些情况下 goto
可以带来好处并提高可读性。例如,它可以转义嵌套循环和 switch
语句。
例子:
using System;
namespace goto_Example2 {
class Program {
static void Main(string[] args) {
// Intialize the integer variable a
int a = 2;
// Run the function test input
TestInput(a);
// Change the value of a and see how it affects the output from TestInput()
a = 1;
TestInput(a);
a = 3;
TestInput(a);
Console.ReadLine();
}
static void TestInput(int input) {
// In this example function, we only want to accept either 1 or 2 as values.
// If we accept the value 1, we want to add to it and then run case 2;
// Print the original value
Console.WriteLine("Input Being Tested: " + input.ToString());
switch (input) {
case 1:
input++;
// If goto isn't called, the input value will not be modified, and its final value would
// have been unchanged
goto case 2;
case 2:
input = input / 2;
break;
default:
break;
}
Console.WriteLine("Final Value: " + input.ToString() + "\n");
}
}
}
在上面的示例中,我们创建了一个示例函数来根据传递的值执行不同的操作。正在考虑三个案例。第一个是值是否等于 1。如果是这种情况,我们将添加到输入值,然后使用 goto
函数继续到情况 2。如果没有调用 goto
,输入值将保持不变。
在情况 2 中,我们将输入除以 2。最后,传递的任何其他值都将属于默认情况,根本不会被修改。最后,打印出最终值并显示案例 1 和 2 将产生相同的绝对值。由于 goto
,即使最初不符合案例规范,也可以通过跳转到其语句来应用案例。
输出:
Input Being Tested: 2
Final Value: 1
Input Being Tested: 1
Final Value: 1
Input Being Tested: 3
Final Value: 3