在 C# 中下载图片
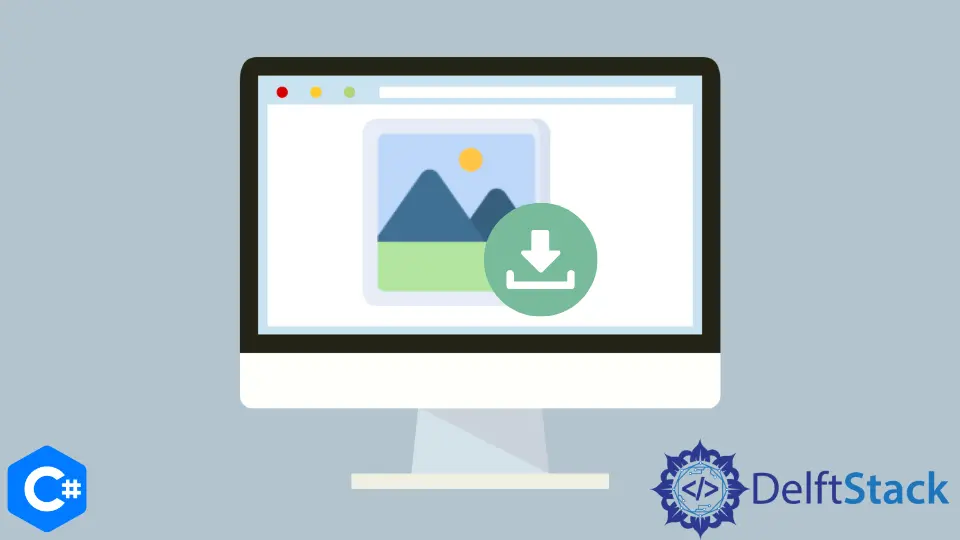
本教程将讨论使用 C# 下载图片的方法。
在 C# 中使用 WebClient
类下载图片
WebClient
类提供了用于向 C# 中的 URL 发送数据和从 URL 接收数据的功能。WebClient.DownloadFile(url, path)
函数从特定的 URL url
下载文件,并将其保存到 path
。我们可以使用 WebClient.DownloadFile()
函数从 URL 下载图片。
using System;
using System.Net;
using System.Runtime.InteropServices;
namespace download_image {
class Program {
static void download() {
string url =
"https://upload.wikimedia.org/wikipedia/commons/thumb/e/ea/Breathe-face-smile.svg/1200px-Breathe-face-smile.svg.png";
using (WebClient client = new WebClient()) {
client.DownloadFile(new Uri(url), "Image.png");
}
}
static void Main(string[] args) {
try {
download();
} catch (ExternalException e) {
Console.WriteLine(e.Message);
} catch (ArgumentNullException e) {
Console.WriteLine(e.Message);
}
}
}
}
在上面的代码中,我们使用 C# 中的 client.DownloadFile(new Uri(url), "Image.png")
函数从 URL url
下载图片并将其保存到路径 Image.png
。
使用 C# 中的 Bitmap
类在不知道格式的情况下下载图片
在上面的示例中,我们必须知道要下载的图片文件格式;然后,我们可以从 URL 下载并保存。但是,如果我们不知道图片文件格式,则可以使用 Bitmap
类。Bitmap
类提供了使用 C# 处理图片的方法。该方法将以 Bitmap
类可以处理的所有格式下载和保存文件。Bitmap.Save(path, format)
函数将我们位图的内容写入格式为 format
的 path
中的文件。ImageFormat
类可以在 Bitmap.Save()
函数内部使用,以手动指定要保存的图片格式。以下代码示例向我们展示了如何在不使用 C# 中的 Bitmap.Save()
函数知道格式的情况下从 URL 下载图片。
using System;
using System.Drawing;
using System.Drawing.Imaging;
using System.IO;
using System.Net;
using System.Runtime.InteropServices;
namespace download_image {
class Program {
static void SaveImage() {
string url =
"https://upload.wikimedia.org/wikipedia/commons/thumb/e/ea/Breathe-face-smile.svg/1200px-Breathe-face-smile.svg.png";
WebClient client = new WebClient();
Stream stream = client.OpenRead(url);
Bitmap bitmap = new Bitmap(stream);
if (bitmap != null) {
bitmap.Save("Image1.png", ImageFormat.Png);
}
stream.Flush();
stream.Close();
client.Dispose();
}
static void Main(string[] args) {
try {
SaveImage();
} catch (ExternalException e) {
Console.WriteLine(e.Message);
} catch (ArgumentNullException e) {
Console.WriteLine(e.Message);
}
}
}
}
我们定义了 SaveImage()
函数,该函数可以下载并保存图片。我们使用 WebClient
类向 url
发出 Web 请求。我们使用了 Stream
类从 client.OpenRead(url)
函数中读取数据。我们使用了 Bitmap
类将流转换为位图格式。然后,我们使用 bitmap.Save()
函数将 bitmap
保存在路径 Image1.png
中,格式为 ImageFormat.Png
。
使用 C# 中的 Image.FromStream()
函数下载不知道格式的图片
我们还可以使用 C# 中的 Image.FromStream()
函数来完成与上一个示例相同的操作。Image.FromStream()
函数从 C# 的内存流中读取图片文件。为此,我们可以先将 URL 中的所有数据下载到字节数组中。然后,我们可以将该数组加载到 MemoryStream
类的对象中。然后,我们可以使用 Image.FromStream()
函数从 MemoryStream
类的对象读取图片。然后,我们可以使用 C# 中的 Image.Save(path, format)
函数将该图片保存为特定格式的路径。以下代码示例向我们展示了如何在不使用 C# 中的 Image.FromStream()
函数知道其格式的情况下,从 URL 下载图片。
using System;
using System.Drawing;
using System.Drawing.Imaging;
using System.IO;
using System.Net;
using System.Runtime.InteropServices;
namespace download_image {
class Program {
static void SaveImage() {
string url =
"https://upload.wikimedia.org/wikipedia/commons/thumb/e/ea/Breathe-face-smile.svg/1200px-Breathe-face-smile.svg.png";
using (WebClient webClient = new WebClient()) {
byte[] data = webClient.DownloadData(url);
using (MemoryStream mem = new MemoryStream(data)) {
using (var yourImage = Image.FromStream(mem)) {
yourImage.Save("Image2.png", ImageFormat.Png);
}
}
}
}
static void Main(string[] args) {
try {
SaveImage();
} catch (ExternalException e) {
Console.WriteLine(e.Message);
} catch (ArgumentNullException e) {
Console.WriteLine(e.Message);
}
}
}
}
我们定义了 SaveImage()
函数,该函数可以下载并保存图片。我们使用了字节数组数据
来存储 webClient.DownloadData(url)
函数返回的数据。然后,我们用 data
初始化了 MemoryStream
类的实例 mem
。然后,使用 Image.FromStream(mem)
函数从 mem
中读取图片 yourImage
。最后,通过使用 yourImage.Save("Image2.png", ImageFormat.Png)
函数,以 ImageFormat.Png
格式将图片 yourImage
保存到 Image2.png
路径。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn