在 C# 中将 XML 反序列化为对象
Abdullahi Salawudeen
2023年1月30日
2022年4月20日
- 使用手动类型的类将 XML 文件反序列化为 C# 对象
-
使用 Visual Studio 的
Paste Special
功能将Xml
反序列化为 C# 对象 -
使用
XSD 工具
将XML
反序列化为 C# 对象
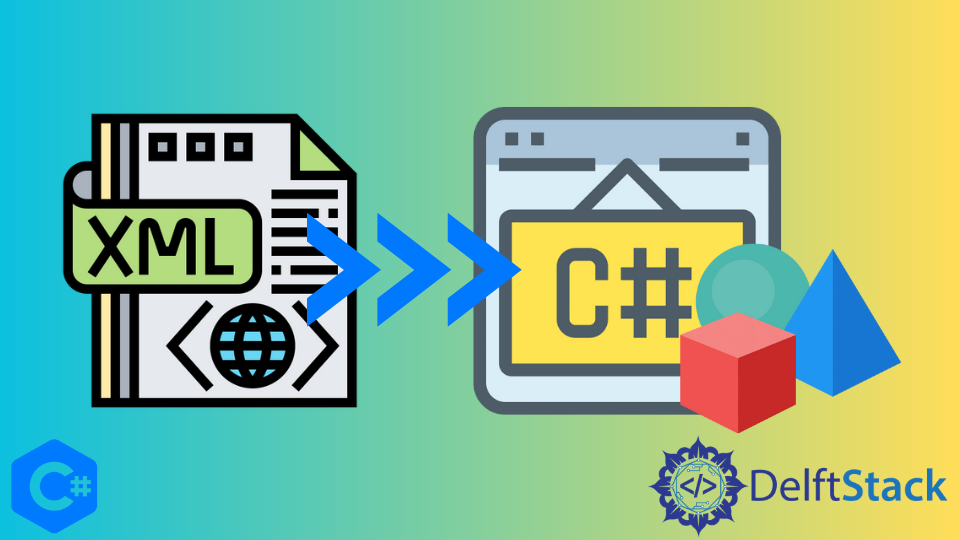
本文将演示将 XML
文件转换或反序列化为 C# 对象。
使用手动类型的类将 XML 文件反序列化为 C# 对象
- C# 与
classes
和objects
以及attributes
和methods
相关联。 Objects
以编程方式用类表示,例如John
或James
。属性
是对象的特征,例如汽车的颜色
、生产年份
、人的年龄
或建筑物的颜色
。XML
是允许解析XML
数据而不考虑传输XML
文件的媒介的标准化格式。
进一步的讨论可通过此参考网页获得。
下面是一个将转换为 C# 对象的 XML
代码示例。
1. <?xml version="1.0" encoding="utf-8"?>
2. <Company xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
3. <Employee name="x" age="30" />
4. <Employee name="y" age="32" />
5. </Company>
将在 C# 中创建具有类似结构的类来转换 XML 代码示例。
using System.Xml.Serialization;
[XmlRoot(ElementName = "Company")]
public class Company
{
public Company()
{
Employees = new List<Employee>();
}
[XmlElement(ElementName = "Employee")]
public List<Employee> Employees { get; set; }
public Employee this[string name]
{
get { return Employees.FirstOrDefault(s => string.Equals(s.Name, name, StringComparison.OrdinalIgnoreCase)); }
}
}
public class Employee
{
[XmlAttribute("name")]
public string Name { get; set; }
[XmlAttribute("age")]
public string Age { get; set; }
}
将 XML
对象转换为 C# 的最后一步是使用 System.Xml.Serialization.XmlSerializer
函数来序列化对象。
public T DeserializeToObject<T>(string filepath) where T : class
{
System.Xml.Serialization.XmlSerializer ser = new System.Xml.Serialization.XmlSerializer(typeof(T));
using (StreamReader sr = new StreamReader(filepath))
{
return (T)ser.Deserialize(sr);
}
}
使用 Visual Studio 的 Paste Special
功能将 Xml
反序列化为 C# 对象
此方法需要使用 Microsoft Visual Studio 2012 及更高版本和 .Net Framework 4.5 及更高版本。还必须安装 Visual Studio 的 WCF 工作负载。
XML
文档的内容必须复制到剪贴板。- 在项目解决方案中添加一个新的
empty
类。 - 打开新的类文件。
- 单击 IDE 菜单栏上的
Edit
按钮。 - 从下拉列表中选择
Paste Special
。 - 单击
Paste XML As Classes
。
要使用 Visual Studio 生成的类,请创建 Helpers
类。
using System;
using System.IO;
using System.Web.Script.Serialization; // Add reference: System.Web.Extensions
using System.Xml;
using System.Xml.Serialization;
namespace Helpers
{
internal static class ParseHelpers
{
private static JavaScriptSerializer json;
private static JavaScriptSerializer JSON { get { return json ?? (json = new JavaScriptSerializer()); } }
public static Stream ToStream(this string @this)
{
var stream = new MemoryStream();
var writer = new StreamWriter(stream);
writer.Write(@this);
writer.Flush();
stream.Position = 0;
return stream;
}
public static T ParseXML<T>(this string @this) where T : class
{
var reader = XmlReader.Create(@this.Trim().ToStream(), new XmlReaderSettings() { ConformanceLevel = ConformanceLevel.Document });
return new XmlSerializer(typeof(T)).Deserialize(reader) as T;
}
public static T ParseJSON<T>(this string @this) where T : class
{
return JSON.Deserialize<T>(@this.Trim());
}
}
}
使用 XSD 工具
将 XML
反序列化为 C# 对象
XSD
用于自动生成与 XML
文件或文档中定义的模式等效的 classes
或 objects
。
XSD.exe
通常在以下路径中找到:C:\Program Files (x86)\Microsoft SDKs\Windows\{version}\bin\NETFX {version} Tools\
。进一步的讨论可以通过这个参考获得。
假设 XML 文件保存在此路径中:C:\X\test.XML
。
以下是将 XML
自动反序列化为 C# 类的步骤:
- 在搜索栏中键入
开发人员命令提示符
并单击它以打开。 - 键入
cd C:\X
导航到XML
文件路径。 - 删除
XML
文件中的行号和任何不必要的字符。 - 键入
xsd test.XML
从 test.XML 创建一个等效的XSD 文件
。 - 在同一文件路径中创建一个
test.XSD
文件。 - 键入
XSD /c test.XSD
创建与XML
文件等效的C# classes
。 - 创建一个
test.cs
文件,一个具有XML
文件的精确模式的 C# 类。
输出:
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated by a tool.
// Runtime Version:4.0.30319.42000
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
using System.Xml.Serialization;
//
// This source code was auto-generated by xsd, Version=4.8.3928.0.
//
/// <remarks/>
[System.CodeDom.Compiler.GeneratedCodeAttribute("xsd", "4.8.3928.0")]
[System.SerializableAttribute()]
[System.Diagnostics.DebuggerStepThroughAttribute()]
[System.ComponentModel.DesignerCategoryAttribute("code")]
[System.Xml.Serialization.XmlTypeAttribute(AnonymousType=true)]
[System.Xml.Serialization.XmlRootAttribute(Namespace="", IsNullable=false)]
public partial class Company {
private CompanyEmployee[] itemsField;
/// <remarks/>
[System.Xml.Serialization.XmlElementAttribute("Employee", Form=System.Xml.Schema.XmlSchemaForm.Unqualified)]
public CompanyEmployee[] Items {
get {
return this.itemsField;
}
set {
this.itemsField = value;
}
}
}
/// <remarks/>
[System.CodeDom.Compiler.GeneratedCodeAttribute("xsd", "4.8.3928.0")]
[System.SerializableAttribute()]
[System.Diagnostics.DebuggerStepThroughAttribute()]
[System.ComponentModel.DesignerCategoryAttribute("code")]
[System.Xml.Serialization.XmlTypeAttribute(AnonymousType=true)]
public partial class CompanyEmployee {
private string nameField;
private string ageField;
/// <remarks/>
[System.Xml.Serialization.XmlAttributeAttribute()]
public string name {
get {
return this.nameField;
}
set {
this.nameField = value;
}
}
/// <remarks/>
[System.Xml.Serialization.XmlAttributeAttribute()]
public string age {
get {
return this.ageField;
}
set {
this.ageField = value;
}
}
}
Author: Abdullahi Salawudeen