在 C# 中重复字符串 X 次
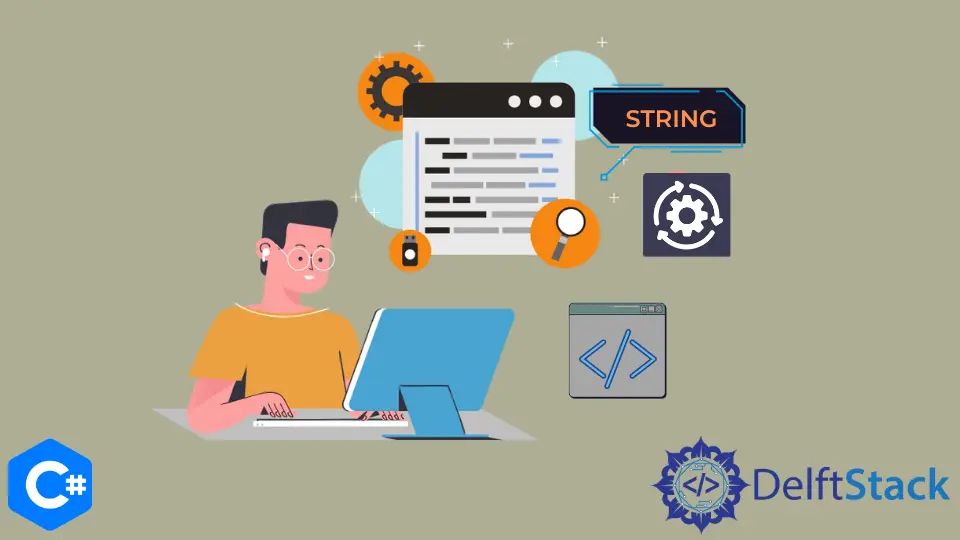
本教程将介绍在 C# 中将字符串重复 x 次的方法。
用 C# 中的 string
类构造函数重复执行 X 次字符串
string
类的构造函数可用于在 C# 的字符串中将特定字符重复指定的次数。我们可以将要重复的字符及其应重复的次数传递给 C# 中的 string
类的构造函数。string(c, x)
构造函数为我们提供了一个字符串,其中字符 c
重复了 x
次。请参见以下代码示例。
using System;
namespace repeat_string_x_times {
class Program {
static void Main(string[] args) {
string str = new string('e', 3);
Console.WriteLine(str);
}
}
}
输出:
eee
在上面的代码中,我们重复了字符 e
3 次,并使用 C# 中的 string('e', 3)
构造函数将其保存在字符串变量 str
中。此方法只能用于在字符串中重复单个字符 x 次。
使用 C# 中的 StringBuilder
类将字符串重复 X 次
StringBuilder
类还可以用于在 C# 中将字符串重复 x 次。StringBuilder
类在 C# 中创建一个可变长度的可变字符串,该字符串具有一定的长度。然后,我们可以使用 StringBuilder.Insert(s,x)
函数插入字符串 s
并重复 x
次。请参见以下代码示例。
using System;
using System.Text;
namespace repeat_string_x_times {
class Program {
static void Main(string[] args) {
string str = new StringBuilder("TEXT".Length * 3).Insert(0, "TEXT", 3).ToString();
Console.WriteLine(str);
}
}
}
输出:
TEXTTEXTTEXT
在上面的代码中,我们重复了字符串 TEXT
3 次,并使用 C# 中的 StringBuilder
类将其保存在字符串变量 str
中。我们首先创建了一个可变字符串,其组合长度为 TEXT.Length * 3
个字符。然后,我们从索引 0
开始,将字符串 TEXT
插入可变字符串内 3 次。然后,使用 C# 中的 ToString()
函数,将可变字符串转换为常规字符串。此方法可用于重复字符串,而不是先前方法中的字符。
在 C# 中使用 LINQ 方法将字符串重复 X 次
LINQ(也称为语言集成查询)用于将 SQL 查询功能与 C# 中的数据结构集成在一起。我们可以使用 LINQ 的 Enumerable.Repeat()
函数在 C# 中重复一个字符串 x 次。Enumerable.Repeat(s, x)
函数有两个参数,字符串变量 s
和整数变量 x
,即字符串变量必须重复的次数。请参见以下代码示例。
using System;
using System.Linq;
namespace repeat_string_x_times {
class Program {
static void Main(string[] args) {
string str = string.Concat(Enumerable.Repeat("TEXT", 3));
Console.WriteLine(str);
}
}
}
输出:
TEXTTEXTTEXT
在上面的代码中,我们重复了字符串 TEXT
3 次,并使用 C# 中 LINQ 的 Enumerable.Repeat("TEXT", 3)
函数将其保存在字符串变量 str
中。我们使用 Enumerable.Repeat()
函数重复该字符串,并使用 String.Concat()
函数将这些值连接为一个字符串。建议使用此方法,因为它与以前的方法具有相同的作用,并且相对简单。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn