在 C# 中删除文件名中的非法字符
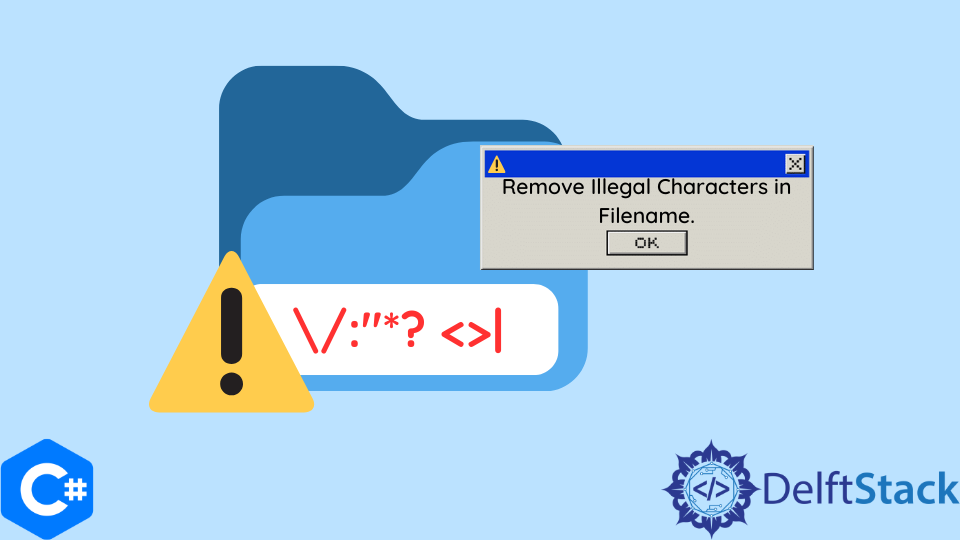
本文是关于使用 C# 从路径中获取文件名的简短教程。它进一步讨论了从文件名中删除非法字符的方法。
在 C#
中获取文件名
C# 库中提供的一些方法用于从完整路径中提取文件名。完整路径可能包含驱动器名称、文件夹名称层次结构以及带有扩展名的实际文件名。
在许多情况下,我们可能需要路径中的文件名。因此,我们可以使用 C# 的 Path
类中的 GetFileName()
方法来提取文件名。
C#
中的 GetFileName()
函数
函数 getFileName()
的语法是:
public static string GetFileName (string completePath);
其中 completePath
是一个字符串,其中包含我们需要从中提取文件名的完整路径,该函数在 string
变量中返回文件名及其扩展名。
让我们看一下 GetFileName()
的工作示例。
using System;
using System.IO;
using System.Text;
namespace mynamespace {
class FileNameExample {
static void Main(string[] args)
{
string stringPath = "C:// files//textFiles//myfile1.txt";
string filename = Path.GetFileName(stringPath);
Console.WriteLine("Filename = " + filename);
Console.ReadLine();
}
} }
输出:
Filename = myfile1.txt
在 C#
中从文件名中删除无效字符
如果在文件名中发现一些非法字符,上述函数可能会给出 ArgumentException
。这些非法字符在函数 GetInvalidPathChars()
和 GetInvalidFilenameChars()
中定义。
我们可以使用以下正则表达式和 Replace
函数从文件名中删除无效或非法字符。
using System;
using System.IO;
using System.Text;
using System.Text.RegularExpressions;
namespace mynamespace
{
class FileNameExample
{
static void Main(string[] args)
{
string invalidFilename = "\"M\"\\y/Ne/ w**Fi:>> l\\/:*?\"| eN*a|m|| e\"ee.?";
string regSearch = new string(Path.GetInvalidFileNameChars()) + new string(Path.GetInvalidPathChars());
Regex rg = new Regex(string.Format("[{0}]", Regex.Escape(regSearch)));
invalidFilename = rg.Replace(invalidFilename, "");
Console.WriteLine(invalidFilename);
}
}
}
输出:
MyNe wFi l eNam eee.
在上面的代码片段中,我们将两个函数(即 GetInvalidPathChars()
和 GetInvalidFilenameChars()
)中的无效字符连接起来,并对结果创建一个正则表达式。
之后,我们从指定的文件名(包含多个无效字符)中搜索所有无效字符,并使用 Replace
函数将它们替换为空白。
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn