在 C# 中将枚举转换为字符串
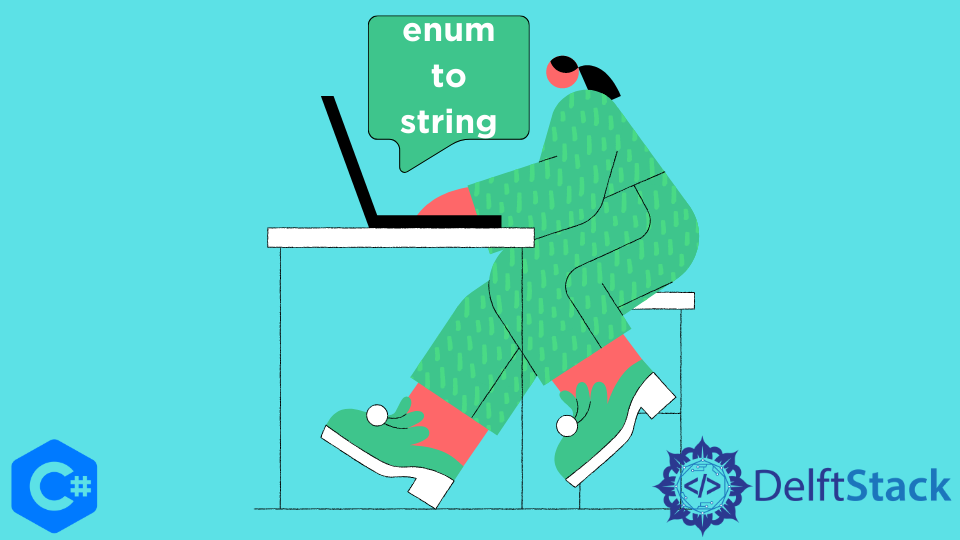
本教程将讨论在 C# 中将枚举转换为字符串的方法。
在 C# 中用 Description
属性将枚举转换为字符串
对于遵循命名约定的简单 Enum 值,我们无需使用任何方法即可将其转换为字符串。可以使用 C# 中的 Console.WriteLine()
函数向用户显示。在下面的编码示例中进行了说明。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace enum_to_string
{
public enum Status
{
InProgress,
Completed
}
class Program
{
static void Main(string[] args)
{
Status complete = Status.Completed;
Console.WriteLine(complete);
}
}
}
输出:
Completed
在上面的代码中,我们直接以 C# 的字符串格式打印 Enum 值 Completed
。这是可能的,因为我们的 Enum 值遵循 C# 中的变量命名约定。但是,如果要显示易于阅读的字符串,则必须在 C# 中使用 Enums 的 Description
属性。Description
属性用于描述枚举的每个值。我们可以通过在 Enum 的 Description
属性中编写字符串,将 Enum 转换为易于阅读的字符串。以下代码示例向我们展示了如何使用 C# 中的 Description
属性将 Enum 值转换为字符串。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Reflection;
namespace enum_to_string
{
public enum Status
{
[Description("The Desired Task is InProgress")]
InProgress,
[Description("The Desired Task is Successfully Completed")]
Completed
}
static class extensionClass
{
public static string getDescription(this Enum e)
{
Type eType = e.GetType();
string eName = Enum.GetName(eType, e);
if (eName != null)
{
FieldInfo fieldInfo = eType.GetField(eName);
if (fieldInfo != null)
{
DescriptionAttribute descriptionAttribute =
Attribute.GetCustomAttribute(fieldInfo,
typeof(DescriptionAttribute)) as DescriptionAttribute;
if (descriptionAttribute != null)
{
return descriptionAttribute.Description;
}
}
}
return null;
}
}
class Program
{
static void Main(string[] args)
{
Status complete = Status.Completed;
string description = complete.getDescription();
Console.WriteLine(description);
}
}
}
输出:
The Desired Task is Successfully Completed
在上面的代码中,我们创建了一个扩展方法 getDescription
,该方法返回 C# 中的 Enum 值描述。该方法可以很好地工作,但是有点复杂。这种复杂性在下一节中得到了简化。
用 C# 中的 switch
语句将 Enum 转为字符串
通过使用 C# 中的 switch
语句,可以简化以前方法的许多复杂性。我们可以使用 C# 中的 switch
语句,为每个 Enum 值的字符串变量分配所需的值。请参见以下代码示例。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Reflection;
namespace enum_to_string
{
public enum Status
{
InProgress,
Completed
}
static class extensionClass
{
public static string getValue(this Status e)
{
switch (e)
{
case Status.InProgress:
return "The Desired Task is InProgress";
case Status.Completed:
return "The Desired Task is Successfully Completed";
}
return String.Empty;
}
}
class Program
{
static void Main(string[] args)
{
Status complete = Status.Completed;
string value = complete.getValue();
Console.WriteLine(value);
}
}
}
输出:
The Desired Task is Successfully Completed
在上面的代码中,我们创建了一个扩展方法 getValue()
,该方法使用 C# 中的 switch
语句返回基于 Enum 值的字符串。getValue()
函数使用 switch
语句,并为我们指定的 Enum 的每个值返回不同的字符串。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn