在 C# 中将 IEnumerable 转换为列表
Abdullahi Salawudeen
2023年10月12日
-
在 C# 中使用
ToList()
将数据从 IEnumerable 转换为列表 -
在 C# 中使用
ToList()
将数据从数组转换为列表 -
在 C# 中使用
ToArray()
将数据从列表转换为数组 -
在 C# 中使用
AsEnumerable()
将数据从 List 转换为 IEnumerable
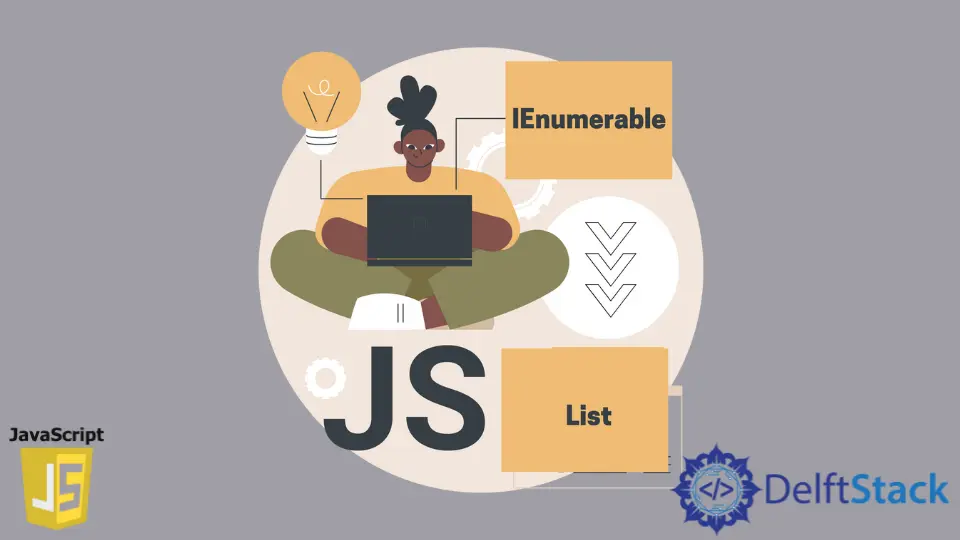
本文将说明将数据从 IEnumerable 转换为 C# 中的列表。
在 C# 中使用 ToList()
将数据从 IEnumerable 转换为列表
IEnumerable 是一个包含在 System.Collections.Generic
命名空间中的接口。像所有其他接口一样,它公开了一个方法。
此案例公开了 enumerator
方法,该方法支持迭代或循环遍历泛型和非泛型列表,包括 LINQ 查询和数组。
IEnumerable 仅包含返回 IEnumerator 对象的 GetEnumerator
方法。
public interface IEnumerable<out T> : System.Collections.IEnumerable
IEnumerable 接口返回的值是只读的。这意味着操作仅限于这些数据。
C# 中的 ToList()
方法是操作这些数据的替代方法。C# 列表中的元素可以添加、删除、排序和重新排列。
与 IEnumerable 值相比,可以对列表执行的操作太多了。
C# List
类表示可以通过索引访问的强类型对象的集合。ToList()
函数或方法位于 System.Linq
命名空间(语言集成查询)中。
LINQ 中的 ToList
运算符从给定源获取元素并返回一个新列表。输入将被转换为类型列表。
ToList()
方法返回字符串实例的列表。ToList()
函数可以在数组引用或 IEnumerable 值上调用,反之亦然。
可通过此参考进行进一步讨论。
可以按如下方式访问或操作列表元素:
// an array of 4 strings
string[] animals = { "Cow", "Camel", "Elephant" };
// creating a new instance of a list
List<string> animalsList = new List<string>();
// use AddRange to add elements
animalsList.AddRange(animals);
// declaring a list and passing array elements into the list
List<string> animalsList = new List<string>(animals);
// Adding an element to the collection
animalsList.Add("Goat");
Console.WriteLine(animalsList[2]); // Output Elephant, Accessing elements of a list
// Collection of new animals
string[] newAnimals = { "Sheep", "Bull", "Camel" };
// Insert array at position 3
animalsList.InsertRange(3, newAnimals);
// delete 2 elements at starting with element at position 3
animalsList.RemoveRange(3, 2);
必须导入命名空间才能使用 ToList
函数,如下所示:
using System.Collections.Generic;
using System.Linq;
下面是 ToList()
方法的语法。
List<string> result = countries.ToList();
下面是在 C# 中将 IEnumerable 转换为列表的示例代码。
using System;
using System.Collections.Generic;
using System.Linq;
namespace TestDomain {
class Program {
public static void Main(String[] args) {
IEnumerable<int> testValues = from value in Enumerable.Range(1, 10) select value;
List<int> result = testValues.ToList();
foreach (int a in result) {
Console.WriteLine(a);
}
}
}
}
输出:
1
2
3
4
5
6
7
8
9
10
在 C# 中使用 ToList()
将数据从数组转换为列表
下面是在 C# 中将数组转换为列表的示例代码。
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ArrayToListApp {
class Program {
static void Main(string[] args) {
// create an array of African countries of type string containing the collection of data
string[] countries = { "Nigeria", "Ghana", "Egypt", "Liberia",
"The Gambia", "Morocco", "Senegal" };
// countries.ToList() convert the data collection into the list.
List<string> result = countries.ToList();
// foreach loop is used to print the countries
foreach (string s in result) {
Console.WriteLine(s);
}
}
}
}
输出:
Nigeria
Ghana
Egypt
Liberia
The Gambia
Morocco
Senegal
在 C# 中使用 ToArray()
将数据从列表转换为数组
下面是在 C# 中从列表转换为数组的示例代码。
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ListToArrayApp {
class Program {
static void Main(string[] args) {
// create an array of African countries of type string containing the collection of data
IEnumerable<int> testValues = from value in Enumerable.Range(1, 10) select value;
// countries.ToList() convert the data collection into the list.
List<int> result = testValues.ToList();
int[] array = result.ToArray(); // convert string to array.
// foreach loop is used to print the countries
foreach (int i in array) {
Console.WriteLine(i);
}
}
}
}
输出:
1
2
3
4
5
6
7
8
9
10
在 C# 中使用 AsEnumerable()
将数据从 List 转换为 IEnumerable
下面是将 IEnumerable 转换为列表并返回 IEnumerable 的示例代码。
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ListToArrayApp {
class Program {
static void Main(string[] args) {
List<int> list = new List<int>();
IEnumerable enumerable = Enumerable.Range(1, 5);
foreach (int item in enumerable) {
list.Add(item);
}
Console.WriteLine("Output as List");
foreach (var item in list) {
Console.WriteLine(item);
}
Console.WriteLine("Output as Enumerable");
// using the AsEnumerable to convert list back to Enumerable
IEnumerable resultAsEnumerable = list.AsEnumerable();
foreach (var item in resultAsEnumerable) {
Console.WriteLine(item);
}
}
}
}
输出:
Output as List
1
2
3
4
5
Output as Enumerable
1
2
3
4
5