在 C# 中连接到 Access 数据库
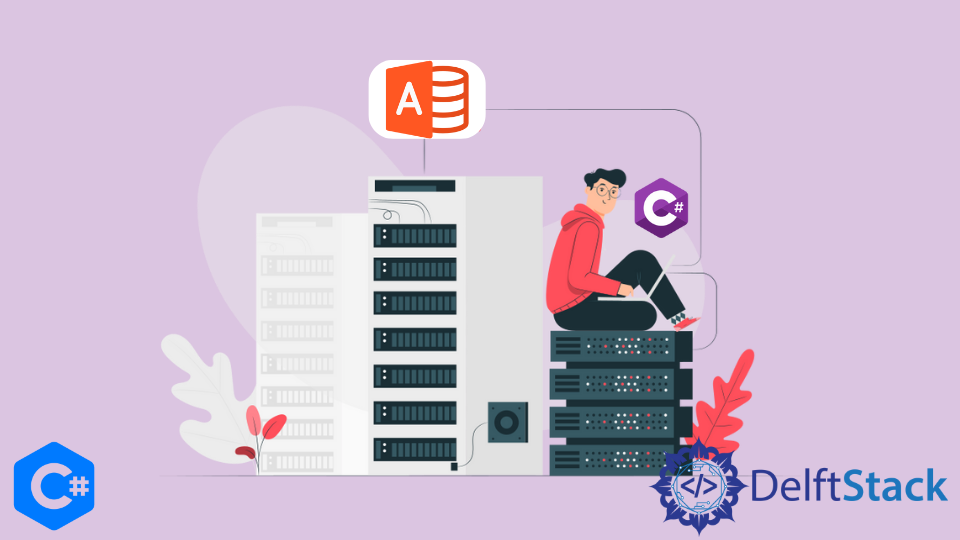
本文将讨论在 C# 中连接到 Access 数据库。
微软访问
Microsoft Access 是一个数据管理程序,允许你保存数据以供将来参考、报告和分析。与 Microsoft Excel 或其他电子表格工具不同,Microsoft Access 使你能够分析大量数据并有效地处理相关数据。
在 C#
中连接到一个 Access 数据库
我们可以按照以下步骤连接到 C# 中的 Access 数据库。
-
首先,打开 Microsoft Access 并选择一个空白桌面数据库。命名数据库,然后单击创建。
-
在数据库中创建一个表,并为其命名。我们将其称为
EmployeeInfo
,有四列:Eid
、Ename
、Edept
和Eaddress
。 -
现在,启动 Microsoft Visual Studio 并创建一个新的 Windows 窗体应用程序。在解决方案资源管理器中,将数据库文件从 Documents 拖放到使用 Microsoft Access 生成的 Project Directory 文件夹中。
-
创建如下表单设计:
-
双击
提交数据
按钮,当你双击1
按钮时,将创建一个事件。 -
现在,为连接添加以下库:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.Data.OleDb;
-
通过转到
工具
并从列表中选择连接到数据库
来生成连接字符串,然后从项目目录中浏览数据库。 -
从列表中选择
高级
,然后选择提供者
。复制文本作为连接字符串。 -
现在,创建一个连接字符串并将其分配给静态字符串类型变量
constr
,如下所示:static string constr = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application .StartupPath + "/employeeinfo.mdb";
-
初始化一个
OleDbConnection
类型变量dbcon
以建立连接并将连接字符串constr
作为参数传递:OleDbConnection dbcon = new OleDbConnection(constr);
-
最后,添加这几行代码,输入员工姓名、部门、地址等员工信息。
OleDbCommand cmd = dbcon.CreateCommand(); dbcon.Open(); cmd.CommandText = "Insert into EmployeeInfo (Ename, Edept,Eaddress)Values('" + txtEmpname.Text + "','" + txtEmpdept.Text + "','" + txtEmpaddress.Text + "')"; cmd.Connection = dbcon; cmd.ExecuteNonQuery(); MessageBox.Show("Data Inserted Successfully"); dbcon.Close();
示例源代码
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.OleDb;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
static string constr = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application.StartupPath + "/employeeinfo.mdb";
OleDbConnection dbcon = new OleDbConnection(constr);
public Form1()
{
InitializeComponent();
}
private void button1_Click_1(object sender, EventArgs e)
{
OleDbCommand cmd = dbcon.CreateCommand();
dbcon.Open();
cmd.CommandText = "Insert into EmployeeInfo (Ename, Edept,Eaddress.)Values('" + txtEmpname.Text + "','" + txtEmpdept.Text + "','" + txtEmpaddress.Text + "')";
cmd.Connection = dbcon;
cmd.ExecuteNonQuery();
MessageBox.Show("Data Inserted", "Congrats");
dbcon.Close();
}
}
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn