在 C# 中检查一个字符串是否为空或 null
Muhammad Maisam Abbas
2023年1月30日
2021年4月29日
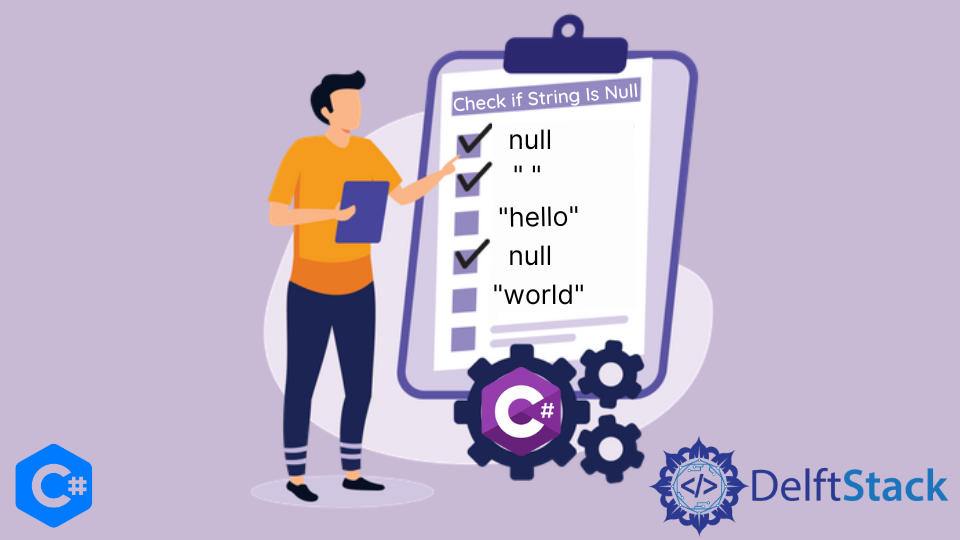
本教程将讨论在 C# 中检查字符串是否为空或 null
的方法。
检查 C# 中的字符串是空或者 null
如果我们要检查其中包含 null
值或""
值的字符串,可以在 C# 中使用 string.IsNullOrEmpty()
方法。string.IsNullOrEmpty()
方法具有布尔返回类型。如果字符串为空或 null
,则返回 true
。请参见以下代码示例。
using System;
namespace check_string
{
class Program
{
static void Main(string[] args)
{
string s = null;
if (string.IsNullOrEmpty(s))
{
Console.WriteLine("String is either null or empty");
}
}
}
}
输出:
String is either null or empty
在上面的代码中,我们将 null
值分配给了字符串变量 s
,并使用 C# 中的 string.IsNullOrEmpty()
方法检查了该值是空还是 null
。
在 C# 中检查一个字符串是否为空
在上一节中,我们检查 null
值和 ""
值的组合。如果要单独检查字符串是否为 null
,则可以使用 ==
比较运算符。请参见以下代码示例。
using System;
namespace check_string
{
class Program
{
static void Main(string[] args)
{
string s = null;
if(s == null)
{
Console.WriteLine("String is null");
}
}
}
}
输出:
String is null
在上面的代码中,我们使用 C# 中的 ==
比较运算符检查字符串变量 s
是否为 null
。
在 C# 中检查一个字符串变量是否是空
与前面的示例一样,我们还可以使用 C# 中的 string.Empty
字段单独检查字符串是否为空。string.Empty
字段代表 C# 中的空白。请参见以下代码示例。
using System;
namespace check_string
{
class Program
{
static void Main(string[] args)
{
string s = "";
if(s == string.Empty)
{
Console.WriteLine("String is empty");
}
}
}
}
输出:
String is empty
在上面的代码中,我们使用 C# 中的 string.Empty
字段检查字符串是否为空。
Author: Muhammad Maisam Abbas
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn