在 C# 中向数组中添加字符串
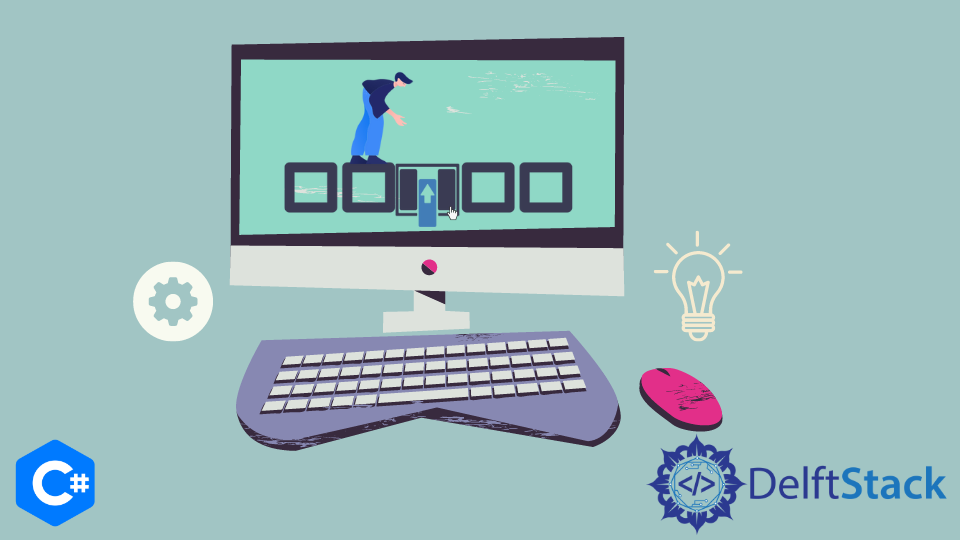
本教程将讨论在 C# 中向一个完全填充的数组添加新字符串的方法。
使用 C# 中的 List.Add()
方法将字符串添加到数组
不幸的是,没有内置的方法可以在 C# 中向数组添加新值。在 C# 中,List 数据结构应该被用于动态分配和取消分配。但是,如果我们有一个包含一些重要数据的填充数组,并且想向该数组添加一个新元素,则可以遵循以下过程。Linq 用于在 C# 中的数据结构上集成 SQL 的查询功能。我们可以使用 Linq 的 ToList()
方法将数组转换为列表,然后使用 C# 中的 List.Add()
方法将值添加到列表中。最后,我们可以使用 List.ToArray()
方法将列表转换回数组。下面的代码示例向我们展示了如何使用 C# 中的 List.Add()
方法将新元素添加到完全填充的数组中。
using System;
using System.Collections.Generic;
using System.Linq;
namespace Array_Add
{
class Program
{
static void Main(string[] args)
{
string[] arr = { "One", "Two", "Three" };
string newElement = "New Element";
List<string> list = new List<string>(arr.ToList());
list.Add(newElement);
arr = list.ToArray();
foreach(var e in arr)
{
Console.WriteLine(e);
}
}
}
}
输出:
One
Two
Three
New Element
我们在上面的代码中初始化了字符串 arr
和字符串变量 newElement
的数组。我们使用 arr.ToList()
方法将 arr
数组转换为列表 list
。然后,我们使用 list.Add(newElement)
方法将 newElement
添加到 list
中。最后,我们使用 C# 中的 list.ToArray()
方法将 list
列表转换回 arr
数组。
使用 C# 中的 Array.Resize()
方法将字符串添加到数组
我们还可以使用以下代码将新元素添加到 C# 中完全填充的数组中。Array.Resize()
方法更改 C# 中一维数组的元素数。Array.Resize()
方法将对数组及其新长度的引用作为参数,并调整数组的大小。每次必须向其中添加一个新元素时,我们都可以将数组大小增加一个元素。下面的代码示例向我们展示了如何使用 C# 中的 Array.Resize()
方法将一个新元素添加到一个完全填充的数组中。
using System;
using System.Collections.Generic;
namespace Array_Add
{
class Program
{
static void Main(string[] args)
{
string[] arr = { "One", "Two", "Three" };
string newElement = "New Element";
Array.Resize(ref arr, arr.Length + 1);
arr[arr.Length - 1] = newElement;
foreach (var e in arr)
{
Console.WriteLine(e);
}
}
}
}
输出:
One
Two
Three
New Element
我们在上面的代码中初始化了字符串 arr
和 newElement
字符串数组。我们使用 Array.Resize()
函数将 arr
数组的大小增加了一个元素,并将 newElement
分配给 arr
数组的最后一个索引。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn