在 C++ 中将字符串转换为整数数组
-
使用
std::getline
和std::stoi
函数在 C++ 中将string
转换为int
数组 -
在 C++ 中使用
std::string::find
和std::stoi
函数将string
转换为int
数组 -
使用
std::copy
和std::remove_if
函数在 C++ 中将string
转换为int
数组
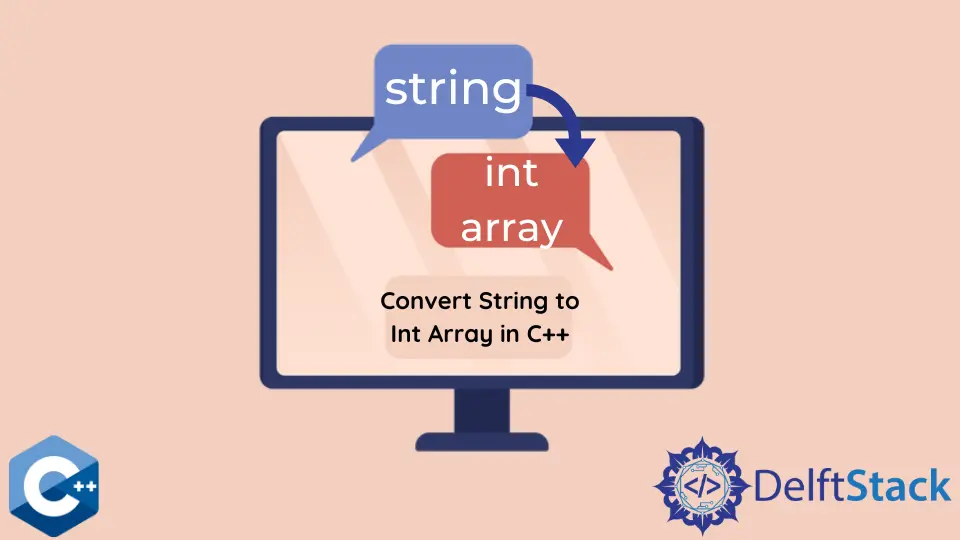
本文将演示有关如何在 C++ 中将字符串转换为整数数组的多种方法。
使用 std::getline
和 std::stoi
函数在 C++ 中将 string
转换为 int
数组
std::stoi
用于将字符串值转换为带符号的整数,它采用一个类型为 std::string
的强制性参数。可选地,该函数可以接受 2 个附加参数,其中第一个可用于存储最后一个未转换字符的索引。第三个参数可以选择指定输入的数字基数。注意,我们假设用逗号分隔的数字作为输入字符串,例如 .csv
文件。因此,我们使用 getline
函数来解析每个数字,然后将该值传递给 stoi
。通过在每次迭代中调用 push_back
方法,我们还将每个数字存储在 std::vector
容器中。
#include <iostream>
#include <iterator>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
using std::stringstream;
using std::vector;
int main(int argc, char *argv[]) {
string text = "125, 44, 24, 5543, 111";
vector<int> numbers;
int num;
stringstream text_stream(text);
string item;
while (std::getline(text_stream, item, ',')) {
numbers.push_back(stoi(item));
}
for (auto &n : numbers) {
cout << n << endl;
}
exit(EXIT_SUCCESS);
}
输出:
125
44
24
5543
111
在 C++ 中使用 std::string::find
和 std::stoi
函数将 string
转换为 int
数组
另外,我们可以利用 std::string
类的 find
内置方法来检索逗号分隔符的位置,然后调用 substr
函数来获取数字。然后,将 substr
结果传递到 stoi
,该 stoi
本身链接到 push_back
方法,以将数字存储到 vector
中。请注意,while
循环后有一行是提取序列中最后一个数字所必需的。
#include <iostream>
#include <iterator>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
using std::stringstream;
using std::vector;
int main(int argc, char *argv[]) {
string text = "125, 44, 24, 5543, 111";
vector<int> numbers;
size_t pos = 0;
while ((pos = text.find(',')) != string::npos) {
numbers.push_back(stoi(text.substr(0, pos)));
text.erase(0, pos + 1);
}
numbers.push_back(stoi(text.substr(0, pos)));
for (auto &n : numbers) {
cout << n << endl;
}
exit(EXIT_SUCCESS);
}
输出:
125
44
24
5543
111
使用 std::copy
和 std::remove_if
函数在 C++ 中将 string
转换为 int
数组
提取整数的另一种方法是将 std::copy
算法与 std::istream_iterator
和 std::back_inserter
结合使用。此解决方案将字符串值存储到向量
中,并将其输出到 cout
流,但是可以轻松添加 std::stoi
函数以将每个元素转换为 int
值。请注意,以下示例代码仅将数字存储为字符串值。
#include <iostream>
#include <iterator>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
using std::stringstream;
using std::vector;
int main(int argc, char *argv[]) {
string text = "125, 44, 24, 5543, 111";
vector<string> nums;
std::istringstream iss(text);
copy(std::istream_iterator<string>(iss), std::istream_iterator<string>(),
std::back_inserter(nums));
for (auto &n : nums) {
n.erase(std::remove_if(n.begin(), n.end(), ispunct), n.end());
cout << n << endl;
}
exit(EXIT_SUCCESS);
}
输出:
125
44
24
5543
111
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn