在 C++ 中使用 STL 堆栈容器
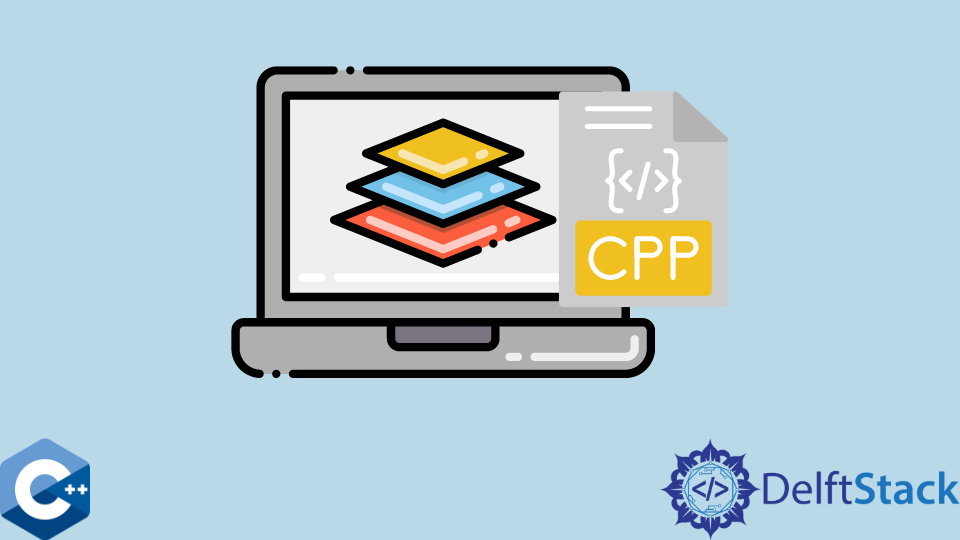
本文将演示如何在 C++ 中使用 STL stack
容器的多种方法。
使用 std::stack
在 C++ 中声明堆栈容器对象
std::stack
被称为容器适配器,它可以充当标准容器的包装器,但提供有限和专门的功能。例如,std::stack
类提供 LIFO(后进先出)数据结构,它可以映射到下面的 std::vector
或 std::deque
容器。std::stack
可以用另一个 stack
对象或兼容的序列容器(如 deque
、vector
和 list
)初始化。但是请注意,用于存储 stack
元素的默认容器是 deque
。此外,没有构造函数支持直接传递初始化列表或元素。一旦声明了 stack
对象,我们就需要使用 push
方法。
请注意,无法通过使用基于范围的循环来迭代 stack
对象。因此,我们实现了一个特殊的 while
循环来将每个元素打印到 cout
流。printStack
函数接受 stack
参数,但是无法将由 vector
对象初始化的 st1
传递给它,因为这两个对象的类型不同并且编译器会抛出错误。
#include <iostream>
#include <stack>
#include <vector>
using std::cout; using std::endl;
using std::stack; using std::vector;
template<typename T>
void printStack(stack<T> s) {
while (!s.empty()) {
cout << s.top() << "; ";
s.pop();
}
cout << endl;
}
int main()
{
vector<int> vec1 = { 1, 2, 3, 4, 11 };
stack st1{ vec1 };
stack<int> st2;
for (int i = 1; i <= 10; ++i) {
st2.push(i * 12);
}
// printStack(st1); Error - no matching function
printStack(st2);
return EXIT_SUCCESS;
}
使用 top()
函数访问 C++ 中最近添加的元素
top()
函数是一个成员函数,它返回堆栈中的顶部元素。请注意,此函数不会自动从 stack
对象中删除返回的元素。应该调用 pop
成员函数来删除它。
#include <iostream>
#include <stack>
using std::cout; using std::endl;
using std::stack;
int main()
{
stack<int> st2;
for (int i = 1; i <= 10; ++i) {
st2.push(i * 12);
}
cout << "top of the stack st2: ";
cout << st2.top() << endl;
return EXIT_SUCCESS;
}
输出:
top of the stack st2: 120
在 C++ 中使用 swap()
函数交换两个堆栈的内容
swap()
函数是 stack
容器的成员函数。它需要对 stack
对象的引用,并从这些堆栈中交换元素。请注意,使用 vector
对象初始化的 st1
对象不能调用 swap
函数或作为它的参数。为了使容器初始化的 stack
能够与 swap
函数一起使用,需要使用 std::move
调用来创建它,因为在以下代码示例中初始化了 st3
对象。后者也可以毫无问题地传递给 printStack
函数。最后,我们交换 st2
/st3
堆栈对象的元素并将结果打印到控制台。
#include <iostream>
#include <stack>
#include <vector>
#include <deque>
using std::cout; using std::endl;
using std::stack; using std::vector;
using std::deque;
template<typename T>
void printStack(stack<T> s) {
while (!s.empty()) {
cout << s.top() << "; ";
s.pop();
}
cout << endl;
}
int main()
{
deque<int> deq1 = { 11, 12, 13, 14 };
vector<int> vec1 = { 1, 2, 3, 4, 11 };
stack st1{ vec1 };
stack<int> st2;
for (int i = 1; i <= 10; ++i) {
st2.push(i * 12);
}
// st2.swap(st1); Error
stack<int> st3 {std::move(deq1)};
printStack(st2);
printStack(st3);
st2.swap(st3);
printStack(st2);
printStack(st3);
return EXIT_SUCCESS;
}
输出:
120; 108; 96; 84; 72; 60; 48; 36; 24; 12;
14; 13; 12; 11;
14; 13; 12; 11;
120; 108; 96; 84; 72; 60; 48; 36; 24; 12;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn